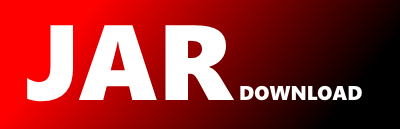
src.openwfe.org.engine.impl.participants.FileArchivingParticipant Maven / Gradle / Ivy
/*
* Copyright (c) 2005-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: FileArchivingParticipant.java 2694 2006-05-29 21:00:05Z jmettraux $
*/
//
// FileArchivingParticipant.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.01 2004/05/19 ([email protected])
//
package openwfe.org.engine.impl.participants;
import openwfe.org.ApplicationContext;
import openwfe.org.engine.Definitions;
import openwfe.org.engine.workitem.WorkItem;
import openwfe.org.engine.workitem.InFlowWorkItem;
import openwfe.org.engine.dispatch.DispatchingException;
import openwfe.org.engine.expressions.FlowExpressionId;
import openwfe.org.engine.participants.ParticipantMap;
import openwfe.org.engine.participants.LeafParticipant;
import openwfe.org.engine.impl.workitem.xml.XmlWorkItemCoder;
/**
* This is an example of custom participant : instances of this class
* simply dump the passing workitem in a file (as XML) and then
* reply immediately to the engine.
*
* Note that archiving a workitem does not equate to terminating its flow.
*
* This class extends LeafParticipant and overrides some of its method
* to provide the desired behaviour.
*
* CVS Info :
*
$Author: jmettraux $
*
$Id: FileArchivingParticipant.java 2694 2006-05-29 21:00:05Z jmettraux $
*
* @author [email protected]
*/
public class FileArchivingParticipant
extends LeafParticipant
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(FileArchivingParticipant.class.getName());
//
// CONSTANTS & co
/**
* The path to where (directory) the archived workitems should be put.
* The default value for this parameter 'archivalDir' is
* simply 'archive'.
*/
public final static String P_ARCHIVAL_DIR
= "archivalDir";
//
// FIELDS
private String archivalDir = null;
//
// CONSTRUCTORS
/**
* When instantiating a participant, the participant map calls
* this method on it to make it ready to operate.
*
* @param regex the name (or regular expression) which maps to this
* participant implementation
* @param params the initialization parameters for this participant, as
* found in the participant map
*/
public void init
(String regex,
java.util.Map params)
{
super.init(regex, params);
//
// archival path
this.archivalDir = (String)params.get(P_ARCHIVAL_DIR);
if (this.archivalDir == null)
this.archivalDir = "archive";
if ( ! this.archivalDir.endsWith(java.io.File.separator))
this.archivalDir += java.io.File.separator;
log.info("init() archivalDir is '"+this.archivalDir+"'");
}
//
// METHODS from Participant
/**
* Overrides LeafParticipant's dispatch method, will take care
* of saving the incoming workitem in file and then will hand back the
* workitem to the engine (via the expression pool of course).
*
* @param context the engine context
* @param wi the workitem handed by the engine
*/
public Object dispatch
(ApplicationContext context, WorkItem wi)
throws
DispatchingException
{
save(context, wi);
reply(context, wi);
return null;
//
// no feedback required
}
//
// METHODS
/**
* Returns the path to where the archive files are to be stored.
*/
public String getArchivalDir ()
{
return this.archivalDir;
}
/**
* Saves the workitem.
* This method is protected so that it can easily get overridden by
* further extending classes.
*
* @param context the engine context
* @param wi the workitem has handled by the engine to this participant
*/
protected void save (ApplicationContext context, WorkItem wi)
throws DispatchingException
{
XmlWorkItemCoder coder = Definitions.getXmlCoder
//(this.getParticipantMap().getContext());
(context);
String fileName = determineFileName(((InFlowWorkItem)wi).getId());
java.io.FileOutputStream fos = null;
try
{
fos = new java.io.FileOutputStream(fileName);
byte[] bWi = coder.doEncode(wi);
fos.write(bWi);
fos.flush();
if (log.isDebugEnabled())
log.debug("save() archived wi to '"+fileName+"'");
}
catch (Throwable t)
{
throw new DispatchingException
("failed to archive (save) workitem to '"+fileName+"'", t);
}
finally
//
// making sure the file is really closed.
{
try
{
fos.close();
}
catch (Throwable t)
{
// ignore
}
}
}
/**
* Overridable as well, determines, based on the flow expression id, where
* the workitem should be stored (in the archive directory though).
*
* @param fei the FlowExpressionId of the workitem to 'archive'.
*/
protected String determineFileName (FlowExpressionId fei)
{
StringBuffer sb = new StringBuffer();
sb.append(getArchivalDir());
sb
.append("arc_")
.append(fei.getWorkflowDefinitionName())
.append("_")
.append(fei.getWorkflowDefinitionRevision())
.append("__")
.append(fei.getWorkflowInstanceId())
.append("_e")
.append(fei.getExpressionId())
.append(".xml");
return sb.toString();
}
/* *
* (this method got transferred to the parent class 'LeafParticipant')
*
*
* Replies to the engine.
* This method is protected as well, but there shouldn't be any need
* to override it.
*
* @param context the engine ApplicationContext
* @param wi the param as it should resume along its flow.
* /
protected void reply (ApplicationContext context, WorkItem wi)
throws DispatchingException
{
InFlowWorkItem ifwi = (InFlowWorkItem)wi;
try
{
Definitions.getExpressionPool(context)
.reply(ifwi.getId(), ifwi);
}
catch (Throwable t)
{
throw new DispatchingException
("failed to feed back workitem to engine", t);
}
}
*/
//
// STATIC METHODS
}