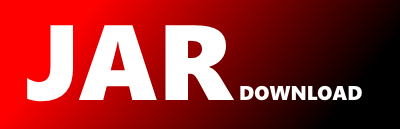
src.openwfe.org.engine.impl.participants.MailNotifiedParticipant Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: MailNotifiedParticipant.java 2494 2006-04-15 21:15:08Z jmettraux $
*/
//
// MailNotifiedParticipant.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.01 2004/05/19 ([email protected])
//
package openwfe.org.engine.impl.participants;
import javax.mail.Message;
import javax.mail.Session;
//import javax.mail.Transport;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.InternetAddress;
import org.apache.velocity.Template;
import org.apache.velocity.VelocityContext;
import org.apache.velocity.app.Velocity;
import openwfe.org.Utils;
import openwfe.org.MapUtils;
import openwfe.org.ApplicationContext;
import openwfe.org.mail.MailUtils;
import openwfe.org.engine.workitem.WorkItem;
import openwfe.org.engine.workitem.InFlowWorkItem;
import openwfe.org.engine.workitem.AttributeUtils;
import openwfe.org.engine.workitem.StringAttribute;
import openwfe.org.engine.dispatch.DispatchingException;
import openwfe.org.engine.expressions.FlowExpressionId;
import openwfe.org.engine.participants.ParticipantMap;
import openwfe.org.engine.participants.LeafParticipant;
/**
* A participant that also receives an email notification when a workitem
* is applied to it.
*
* CVS Info :
*
$Author: jmettraux $
*
$Id: MailNotifiedParticipant.java 2494 2006-04-15 21:15:08Z jmettraux $
*
* @author [email protected]
*/
public class MailNotifiedParticipant
extends LeafParticipant
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(MailNotifiedParticipant.class.getName());
//
// CONSTANTS & co
/**
* Use this parameter ('template') to tell the participant which text
* template file it should use in order to generate the mail message
* to send.
*/
public final static String P_TEMPLATE
= "template";
private final static String DEFAULT_TEMPLATE
= "This is a notification :\n you received an OpenWFE workitem ('${__subject__}')";
/**
* Use this parameter ('recipient-field') to tell the participant which
* field of the applied workitem contains the recipient (or recipient list)
* for the notification email.
*/
public final static String P_RECIPIENT_FIELD
= "recipient-field";
/**
* The default recipient workitem field is "__recipient__"
*/
public final static String DEFAULT_RECIPIENT_FIELD
= "__recipient__";
/* *
* Use this parameter ('smtp-host') to tell the participant which
* mail server it should use to send the notification email
* /
public final static String P_SMTP_SERVER
= "smtp-server";
/* *
* Use this parameter ('smtp-port') to tell the participant which port
* of the mail server it should use to send the notification email
* /
public final static String P_SMTP_PORT
= "smtp-port";
/**
* Use this parameter ('mail-from') to indicate who should be the
* apparent author of the notification email
*/
public final static String P_MAIL_FROM
= "mail-from";
/**
* Use this participant parameter named 'mail-encoding' to set the
* encoding to use for generating emails.
* By default, iso-8859-1 is used.
*/
public final static String P_MAIL_ENCODING
= "mail-encoding";
/*
* By default, emails are encoded using iso-8859-1
*/
private final static String DEFAULT_MAIL_ENCODING
= "iso-8859-1";
/**
* $workitem
*/
public final static String C_WORKITEM
= "workitem";
//
// FIELDS
//private String smtpServer = null;
//private int smtpPort = 25;
private String recipientField = null;
private String mailFrom = null;
private String mailEncoding = null;
private String templateFileName = null;
//
// CONSTRUCTORS
public void init
(//final ParticipantMap pMap,
final String regex,
final java.util.Map params)
{
//super.init(pMap, regex, params);
super.init(regex, params);
//this.smtpServer = MapUtils.getAsString
// (params, P_SMTP_SERVER, "127.0.0.1");
//this.smtpPort = MapUtils.getAsInt
// (params, P_SMTP_PORT, 25);
this.recipientField = MapUtils.getAsString
(params, P_RECIPIENT_FIELD, DEFAULT_RECIPIENT_FIELD);
this.mailFrom = MapUtils.getAsString
(params, P_MAIL_FROM);
this.mailEncoding = MapUtils.getAsString
(params, P_MAIL_ENCODING);
this.templateFileName = MapUtils.getAsString
(params, P_TEMPLATE);
}
//
// BEAN METHODS (getters and setters)
// not much for the moment...
//
// METHODS from Participant
/**
* Overrides LeafParticipant dispatch method, by dispatching
* then sending the email notification.
*/
public Object dispatch
(final ApplicationContext context, final WorkItem wi)
throws
DispatchingException
{
final Object result = super.dispatch(context, wi);
try
{
sendNotificationMail((InFlowWorkItem)wi);
log.debug("apply() notification email sucessfully sent.");
}
catch (final Exception e)
{
log.warn("apply() failed to send notification mail", e);
//throw new MinorDispatchingException
// ("Failed to send notification email", e);
}
return result;
}
//
// METHODS
private String[] splitSubjectAndBody (String in)
{
in = in.trim();
final int i = in.indexOf("\n");
if (i < 0) return new String[] { "", in };
return new String[] { in.substring(0, i), in.substring(i+1) };
}
private void sendNotificationMail
(final InFlowWorkItem wi)
throws
Exception
{
//
// prepare mail session
/*
final java.util.Properties props = new java.util.Properties();
props.put("mail.smtp.host", this.smtpServer);
props.put("mail.smtp.port", ""+this.smtpPort);
final Session mailSession = Session.getDefaultInstance(props, null);
*/
final Session mailSession = MailUtils.getMailSession(getParams());
//
// prepare mime message
final MimeMessage message = new MimeMessage(mailSession);
message.setSentDate(new java.util.Date());
MailUtils.setMailFrom(message, getParams());
//
// prepare templating context
final VelocityContext context = new VelocityContext();
java.util.Iterator it = wi.getAttributes().stringKeySet().iterator();
while (it.hasNext())
{
String k = (String)it.next();
context.put(k, wi.getAttributes().get(k).toString());
}
context.put(C_WORKITEM, wi);
//
// prepare recipient list
final java.util.List recipients = AttributeUtils.owfe2list
(wi.getAttributes().get(new StringAttribute(this.recipientField)),
", *");
it = recipients.iterator();
while (it.hasNext())
{
final String recipient = (String)it.next();
message.addRecipient
(Message.RecipientType.TO, new InternetAddress(recipient));
}
//
// do templating
Velocity.init();
//final Template template =
// Velocity.getTemplate(this.templateFileName, this.mailEncoding);
final Template template = Velocity.getTemplate(this.templateFileName);
final java.io.StringWriter writer = new java.io.StringWriter();
final java.io.BufferedWriter bw = new java.io.BufferedWriter(writer);
template.merge(context, bw);
bw.flush();
bw.close();
final String[] ss = splitSubjectAndBody(writer.toString());
String encoding = Utils.getEncoding();
if (mailEncoding != null) encoding = mailEncoding;
message.setFrom(new InternetAddress(this.mailFrom));
message.setSubject(ss[0]);
message.setText(ss[1], encoding);
//
// send mail
//Transport.send(message);
mailSession.getTransport("smtp").send(message);
log.debug("sendNotificationMail() done.");
}
}