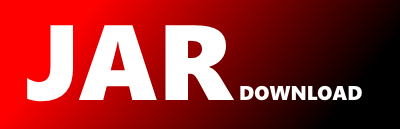
src.openwfe.org.engine.launch.Launcher Maven / Gradle / Ivy
/*
* Copyright (c) 2005-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: Launcher.java 2852 2006-06-22 07:04:12Z jmettraux $
*/
//
// Launcher.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.01 2004/05/19 ([email protected])
//
package openwfe.org.engine.launch;
import openwfe.org.engine.workitem.LaunchItem;
import openwfe.org.engine.workitem.InFlowWorkItem;
import openwfe.org.engine.expressions.BuildException;
import openwfe.org.engine.expressions.FlowExpression;
import openwfe.org.engine.expressions.FlowExpressionId;
import openwfe.org.engine.expressions.ProcessDefinition;
import openwfe.org.engine.expressions.AbstractFlowExpression;
import openwfe.org.engine.expressions.raw.RawExpression;
/**
* Methods for launching flows
*
* CVS Info :
*
$Author: jmettraux $
*
$Id: Launcher.java 2852 2006-06-22 07:04:12Z jmettraux $
*
* @author [email protected]
*/
public interface Launcher
{
/**
* When a launch request is processed and the workflow definition URL
* starts with that 'field:' prefix, the process definition is
* simply embedded within the launchitem / workitem in the given field
* (the name of the field is just after the prefix).
*/
public final static String URL_FIELD_PREFIX
= "field:";
/**
* Launches a flow.
*
* @param async if this param is set to false, the launch() call will
* only return when the flow launch succeded (takes more time than a 'async'
* call).
*/
public FlowExpressionId launch
(LaunchItem li, boolean async)
throws
LaunchException;
/**
* Launches a flow (possibly a subflow).
*
* @param async if this param is set to false, the launch() call will
* only return when the flow launch succeded (takes more time than a 'async'
* call).
*/
public FlowExpressionId launch
(InFlowWorkItem wi,
FlowExpressionId parentId,
String wfdUrl,
boolean async)
throws
LaunchException;
/**
* Launches a subflow.
*
* @param variables the set of variables that have to be put in the new
* environment of the expression. If this param is null, no new environment
* will be created and the expression will use the environment of the
* parent expression (if there is one).
* @param async if this param is set to false, the launch() call will
* only return when the flow launch succeded (takes more time than a 'async'
* call).
*/
public FlowExpressionId launchSub
(InFlowWorkItem wi,
FlowExpressionId parentId,
FlowExpressionId templateId,
java.util.Map variables,
boolean async)
throws
LaunchException;
/**
* Fetches the attributes from the uninterpreted raw version of a flow
* expression.
* (For SimpleXmlLauncher, the raw version is a jdom XML element).
*/
public java.util.Map fetchAttributes
(FlowExpression fe, Object rawExpression)
throws
BuildException;
/**
* This method is used by droflo to load completety a workflowd
* definition for display or edition.
*/
public ProcessDefinition loadProcessDefinition (String url)
throws BuildException;
/**
* This method is used by droflo to load completety a workflowd
* definition for display or edition.
*/
public ProcessDefinition loadProcessDefinitionFromString
(String xmlDefinition)
throws
BuildException;
/**
* Loads a sub process definition.
*/
public ProcessDefinition loadProcessDefinition
(FlowExpressionId parentId,
Object rawExpression)
throws
BuildException;
/**
* This is used by the EvalExpression and also by the
* SubProcessRefExpression when evaluation nested children.
* This method doesn't apply the newly created tree of expressions,
* it simply returns it.
*
* @param caller the FlowExpression calling this eval method.
* @param rawScript the snippet of POOL (Process Oriented OpenWFE Lisp)
* that has to be evaluated.
*
* @return the newly created RawExpression, ready to be applied.
*/
public RawExpression eval
(FlowExpression caller, Object rawScript, InFlowWorkItem wi)
throws
Exception;
}