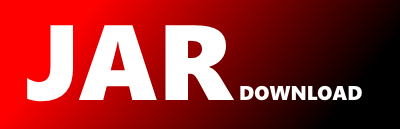
src.openwfe.org.engine.misc.ThreadedStorer Maven / Gradle / Ivy
/*
* Copyright (c) 2005, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: ThreadedStorer.java 2033 2005-08-14 09:50:21Z jmettraux $
*/
//
// ThreadedStorer.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.01 2004/05/19 ([email protected])
//
package openwfe.org.engine.misc;
import openwfe.org.engine.expressions.InFlowObject;
import openwfe.org.engine.expressions.FlowExpressionId;
/**
* Threaded storage for InFlowObject instances (expressions and workitems).
*
* CVS Info :
*
$Author: jmettraux $
*
$Id: ThreadedStorer.java 2033 2005-08-14 09:50:21Z jmettraux $
*
* @author [email protected]
*/
public abstract class ThreadedStorer
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(ThreadedStorer.class.getName());
//
// CONSTANTS & co
//
// FIELDS
private java.util.Set inStorage = new java.util.HashSet();
private java.util.Set inDeletion = new java.util.HashSet();
//
// CONSTRUCTORS
public ThreadedStorer ()
{
super();
}
//
// METHODS
public InFlowObject get (final FlowExpressionId fei)
{
if (this.inDeletion.contains(fei))
//
// preventing "dirty-reads"
{
log.debug("get() prevented dirty-read for "+fei);
return null;
}
return doGet(fei);
}
public void store (final InFlowObject o)
{
final FlowExpressionId id = o.getId();
if (inStorage.contains(id))
{
log.debug("store() discarding supplemental store() request");
return;
}
(new Thread()
{
public void run ()
{
try
{
doStore(o);
}
catch (final Throwable t)
{
log.warn("store() failure for "+o.getId(), t);
}
inStorage.remove(id);
//
// it's very important to remove the id, even
// if the storage was not successful.
log.debug("store() thread done.");
}
}).start();
log.debug("store() done.");
}
public void remove (final InFlowObject o)
{
(new Thread()
{
public void run ()
{
final FlowExpressionId fei = o.getId();
try
{
inDeletion.add(fei);
doRemove(o);
inDeletion.remove(fei);
log.debug("remove() thread done.");
}
catch (final Throwable t)
{
log.warn("remove() failure for "+fei, t);
}
}
}).start();
log.debug("remove() done.");
}
//
// ABSTRACT METHODS
/**
* This method has to be linked with the underlying storage mechanism.
*/
public abstract InFlowObject doGet (FlowExpressionId fei);
/**
* This method has to be linked with the underlying storage mechanism.
*/
public abstract void doStore (InFlowObject o)
throws Exception;
/**
* This method has to be linked with the underlying storage mechanism.
*/
public abstract void doRemove (InFlowObject o)
throws Exception;
//
// STATIC METHODS
}