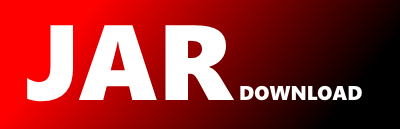
src.openwfe.org.engine.participants.LeafParticipant Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: LeafParticipant.java 2809 2006-06-13 07:47:34Z jmettraux $
*/
//
// LeafParticipant.java
//
// [email protected]
//
// generated with
// jtmpl 1.0.04 20.11.2001 John Mettraux ([email protected])
//
package openwfe.org.engine.participants;
import openwfe.org.Service;
import openwfe.org.MapUtils;
import openwfe.org.ApplicationContext;
import openwfe.org.engine.Definitions;
import openwfe.org.engine.history.History;
import openwfe.org.engine.workitem.WorkItem;
import openwfe.org.engine.workitem.InFlowItem;
import openwfe.org.engine.workitem.LaunchItem;
import openwfe.org.engine.workitem.InFlowWorkItem;
import openwfe.org.engine.dispatch.LocalDispatcher;
import openwfe.org.engine.dispatch.WorkItemDispatcher;
import openwfe.org.engine.dispatch.DispatchingException;
/**
* A participant to a workflow.
* Its name, its dispatcher class and a few parameters
*
* CVS Info :
*
$Author: jmettraux $
*
$Date: 2006-06-13 09:47:34 +0200 (Tue, 13 Jun 2006) $
*
$Id: LeafParticipant.java 2809 2006-06-13 07:47:34Z jmettraux $
*
* @author [email protected]
*/
public class LeafParticipant
extends AbstractParticipant
{
/*
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(LeafParticipant.class.getName());
*/
//
// CONSTANTS & co
/**
* The 'dispatcherClass' parameter is used to indicate to this leaf
* participant which java class it should use for doing the real
* dispatch work.
*/
public final static String P_DISPATCHER_CLASS
= "dispatcherClass";
//
// FIELDS
private String dispatcherClassName = null;
//
// CONSTRUCTORS
public void init
(final String regex,
final java.util.Map params)
{
super.init(regex, params);
this.dispatcherClassName = MapUtils
.getAsString(params, P_DISPATCHER_CLASS);
}
//
// BEAN METHODS
/**
* Returns the class of the dispatcher attached to this participant.
*/
public String getDispatcherClassName ()
{
return this.dispatcherClassName;
}
public void setDispatcherClassName (final String s)
{
this.dispatcherClassName = s;
}
//
// METHODS
/**
* Does the work of instantiating the dispatcher before delivery.
* If it's a local delivery, an instance of LocalDispatcher will
* be returned.
*/
protected WorkItemDispatcher instantiateDispatcher
(final ApplicationContext context, final WorkItem wi)
throws
DispatchingException
{
WorkItemDispatcher dispatcher = null;
String dClassName = null;
if (isLocalDelivery(context, wi))
dClassName = LocalDispatcher.class.getName();
else
dClassName = this.dispatcherClassName;
if (dClassName == null)
{
throw new DispatchingException
("participant '"+this.getRegex()+"' is missing a "+
"'dispatcherClass' parameter, "+
"cannot dispatch workitems to it");
}
try
{
Class dispatcherClass = Class.forName(this.dispatcherClassName);
dispatcher = (WorkItemDispatcher)dispatcherClass.newInstance();
java.util.Map dispatcherParams = new java.util.HashMap();
dispatcherParams.putAll(getParams());
dispatcherParams.put(PARTICIPANT_NAME, this.getRegex());
//
// init dispatcher
dispatcher.init
(getRegex()+".dispatcher",
//((Service)getParticipantMap()).getContext(),
context,
dispatcherParams);
}
catch (Exception e)
{
throw new DispatchingException
("Failed to instantiate dispatcher '"+this.dispatcherClassName+
"' for participant '"+getRegex()+"'", e);
}
return dispatcher;
}
/**
* Returns true if it's an InFlowWorkItem and the target participant
* is the current engine.
*/
protected boolean isLocalDelivery
(final ApplicationContext context, final WorkItem wi)
{
String participantName = null;
final String selfParticipantName = context.getApplicationName();
if (wi instanceof InFlowItem)
{
return selfParticipantName
.equals(((InFlowItem)wi).getParticipantName());
}
/*
* the SubProcessRefExpression class takes care of not sending
* launchitems locally, it spares us this test.
*
if (wi instanceof LaunchItem)
{
return ((LaunchItem)wi).getWorkflowDefinitionUrl()
.startsWith(selfParticipantName+"::");
}
*/
return false;
}
/**
* Handles the workitem for despatchement to this LeafParticipant.
*/
public Object dispatch
(final ApplicationContext context, final WorkItem wi)
throws
DispatchingException
{
final WorkItemDispatcher dispatcher =
instantiateDispatcher(context, wi);
//
// set information that might be relevant
// for a worklist or any other kind of workitem recipient
if (wi instanceof InFlowItem)
{
final InFlowItem ifi = (InFlowItem)wi;
if (ifi.getParticipantName() == null)
ifi.setParticipantName(getRegex());
//if (log.isDebugEnabled())
//{
// log.debug
// ("dispatch() set participant name to >"+getRegex()+
// "< as it was missing.");
//}
}
wi.touch();
//
// history
final History history = Definitions.getHistory
//(((Service)getParticipantMap()).getContext());
//(getParticipantMap().getContext());
(context);
if (wi instanceof InFlowWorkItem && history != null)
{
final InFlowWorkItem ifwi = (InFlowWorkItem)wi;
history.log
(ifwi.getLastExpressionId(),
ifwi,
History.EVT_DISPATCH,
ifwi.getParticipantName(),
this.getClass().getName());
}
//
// dispatch
return dispatcher.dispatch(wi);
}
/**
* Replies to the engine (this method is only used by certain
* extensions of this class).
* This method is protected as well, but there shouldn't be any need
* to override it.
*
* @param context the engine ApplicationContext
* @param wi the param as it should resume along its flow.
*/
protected void reply
(final ApplicationContext context, final WorkItem wi)
throws
DispatchingException
{
final InFlowWorkItem ifwi = (InFlowWorkItem)wi;
try
{
Definitions.getExpressionPool(context)
.reply(ifwi.getId(), ifwi);
}
catch (final Throwable t)
{
throw new DispatchingException
("failed to feed back workitem to engine", t);
}
}
}