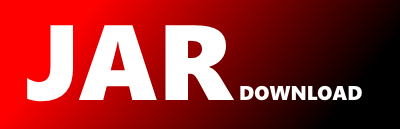
org.ops4j.pax.cdi.openwebbeans.impl.ProxyWeavingHook Maven / Gradle / Ivy
/*
* Copyright 2012 Harald Wellmann.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ops4j.pax.cdi.openwebbeans.impl;
import java.util.List;
import java.util.Map;
import java.util.WeakHashMap;
import org.osgi.framework.Bundle;
import org.osgi.framework.hooks.weaving.WeavingHook;
import org.osgi.framework.hooks.weaving.WovenClass;
import org.osgi.framework.wiring.BundleWire;
import org.osgi.framework.wiring.BundleWiring;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* A weaving hook which adds a dynamic package import for {@code javassist.util.proxy} for all
* classes from bean bundles. This is required for Javassist proxies for managed beans generated by
* OpenWebBeans.
*
* @author Harald Wellmann
*/
public class ProxyWeavingHook implements WeavingHook {
private static Logger log = LoggerFactory.getLogger(ProxyWeavingHook.class);
private Map bundleMap = new WeakHashMap();
@Override
public void weave(WovenClass wovenClass) {
Bundle bundle = wovenClass.getBundleWiring().getBundle();
Boolean seen = bundleMap.get(bundle);
if (seen != null) {
return;
}
boolean isBeanBundle = false;
if (isBeanBundle(bundle)) {
log.debug("weaving {}", wovenClass.getClassName());
wovenClass.getDynamicImports().add("org.apache.webbeans.proxy");
wovenClass.getDynamicImports().add("org.apache.webbeans.intercept");
isBeanBundle = true;
}
bundleMap.put(bundle, isBeanBundle);
// pax-cdi-extension fires CDI events and hence needs dynamic imports
// for OpenWebBeans proxies
if (bundle.getSymbolicName().equals("org.ops4j.pax.cdi.extension")) {
wovenClass.getDynamicImports().add("org.apache.webbeans.*");
}
}
/**
* TODO Copied from BeanBundles.isBeanBundle(). Using that method from pax-cdi-spi
* causes a ClassCircularityError. Is there a better way to avoid this?
* @param candidate
* @return
*/
private static boolean isBeanBundle(Bundle candidate) {
List wires = candidate.adapt(BundleWiring.class).getRequiredWires(
"osgi.extender");
for (BundleWire wire : wires) {
Object object = wire.getCapability().getAttributes().get("osgi.extender");
if (object instanceof String) {
String extender = (String) object;
if (extender.equals("pax.cdi")) {
return true;
}
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy