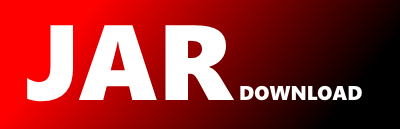
org.ops4j.pax.web.service.whiteboard.ServletMapping Maven / Gradle / Ivy
Show all versions of pax-web-api Show documentation
/*
* Copyright 2008 Alin Dreghiciu.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ops4j.pax.web.service.whiteboard;
import java.util.Map;
import javax.servlet.MultipartConfigElement;
import javax.servlet.Servlet;
/**
* Servlet mapping contains all the information required to register a {@link Servlet} (either directly or
* using whiteboard pattern).
*
* This interface may be used directly, when user registers OSGi services using it as
* {@link org.osgi.framework.Constants#OBJECTCLASS} (explicit whiteboard approach) or will be used internally,
* when user registers actual {@link Servlet} instance with service properties (or class annotations)
* (as recommended by Whiteboard specification).
*
* Registering a {@link Servlet} can be done in two ways:
* - registering a service with this interface - all the information is included in service itself
* (explicit Whiteboard approach)
* - registering a {@link Servlet} service, while required properties (mapping, name, parameters) are specified
* using service registration properties or annotations (OSGi CMPN Whiteboard approach)
*
*
* @author Alin Dreghiciu
* @author Grzegorz Grzybek
* @since 0.4.0, April 05, 2008
*/
public interface ServletMapping extends ContextRelated {
/**
* Get an actual {@link Servlet} instance to register.
* In whiteboard method, this is actual OSGi service instance.
*
* @return the servlet to register
*/
Servlet getServlet();
/**
* Get a class of {@link Servlet} to register. Matches {@code /} element from
* {@code web.xml}. If {@link #getServlet()} is also specified, servlet class isn't used.
* There's no whiteboard specific method to specify this class.
*
* @return the servlet's class to instantiate and register
*/
Class extends Servlet> getServletClass();
/**
* Get a name of the servlet being registered. Matches {@code /} element from
* {@code web.xml}.
* In whiteboard method, this can be specified as:
* - {@link org.osgi.service.http.whiteboard.HttpWhiteboardConstants#HTTP_WHITEBOARD_SERVLET_NAME}
* property
* - {@code servlet-name} service registration property (legacy Pax Web Whiteboard approach)
*
* If not specified, the name defaults to fully qualified class name of the servlet.
*
* @return name of the Servlet being mapped.
*/
String getServletName();
/**
* Get URL patterns to map into the servlet being registered. URL patterns should be specified according
* to Servlet API 4 specification (chapter 12.2) and OSGi CMPN R6+ Whiteboard specification (chapter 140.4).
* It matches {@code /} elements from {@code web.xml}.
* In whiteboard method, this can be specified as:
* - {@link org.osgi.service.http.whiteboard.HttpWhiteboardConstants#HTTP_WHITEBOARD_SERVLET_PATTERN}
* property
* - {@link org.osgi.service.http.whiteboard.propertytypes.HttpWhiteboardServletPattern} annotation
* - {@code urlPatterns} service registration property (legacy Pax Web Whiteboard approach)
*
When passing service registration property, it should be one of {@code String}, {@code String[]} or
* {@code Collection} types.
*
* @return an array of url patterns servlet maps to
*/
String[] getUrlPatterns();
/**
* Get an alias to use for servlet registration. An alias is defined in OSGi CMPN specification
* of {@link org.osgi.service.http.HttpService HTTP Service} and is often (even in specification) confused with
* servlet name: name in the URI namespace. For the purpose of Pax Web and consistency, single
* alias is treated as one-element array of URL Patterns if the patterns are not specified.
* There's no whiteboard specific method to specify an alias.
*
* @return resource alias - effectively changed into 1-element array of urlPatterns
*/
String getAlias();
/**
* Get error page declarations to use for the servlet being registered. The declarations mark the servlet
* as error servlet matching {@code /} and {@code /}
* elementss from {@code web.xml}.
* In whiteboard method, this can be specified as:
* - {@link org.osgi.service.http.whiteboard.HttpWhiteboardConstants#HTTP_WHITEBOARD_SERVLET_ERROR_PAGE}
* property
* - {@link org.osgi.service.http.whiteboard.propertytypes.HttpWhiteboardServletErrorPage} annotation
*
*
* @return Servlet async-supported flag
*/
String[] getErrorPages();
/**
* Get flag for supporting asynchronous servlet invocation. It matches {@code /}
* element from {@code web.xml}.
* In whiteboard method, this can be specified as:
* - {@link org.osgi.service.http.whiteboard.HttpWhiteboardConstants#HTTP_WHITEBOARD_SERVLET_ASYNC_SUPPORTED}
* property
* - {@link org.osgi.service.http.whiteboard.propertytypes.HttpWhiteboardServletAsyncSupported}
* annotation
*
*
* @return Servlet async-supported flag
*/
Boolean getAsyncSupported();
/**
* Get {@link MultipartConfigElement} to configuration multipart support for the servlet being registered.
* See Servlet API 4 specification (chapter 3.2 File upload) for details. It matches
* {@code /} element from {@code web.xml}.
* In whiteboard method, this can be specified as:
* - {@code org.osgi.service.http.whiteboard.HttpWhiteboardConstants#HTTP_WHITEBOARD_SERVLET_MULTIPART_*}
* properties
* - {@link org.osgi.service.http.whiteboard.propertytypes.HttpWhiteboardServletMultipart} annotation
*
*
* @return Servlet multipart configuration
*/
MultipartConfigElement getMultipartConfig();
/**
* Get init parameters for the servlet being registered. It matches {@code /}
* elements from {@code web.xml}.
* In whiteboard method, this can be specified as (no annotation here):
* - {@link org.osgi.service.http.whiteboard.HttpWhiteboardConstants#HTTP_WHITEBOARD_SERVLET_INIT_PARAM_PREFIX}
* prefixed properties (OSGi CMPN Whiteboard approach)
* - {@code init.} prefixed properties (or prefix may be specified using {@code init-prefix}
* service registration property (legacy Pax Web Whiteboard approach)
*
*
* @return map of initialization parameters.
*/
Map getInitParameters();
/**
* Get load on startup value for the servlet being registered. It matches
* {@code /} element from {@code web.xml}.
* Whiteboard specification doesn't mention this parameter.
*
* @return Servlet load-on-startup configuration
*/
Integer getLoadOnStartup();
}