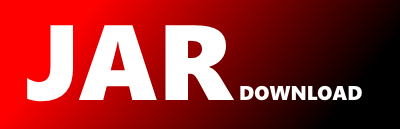
org.ops4j.pax.web.service.internal.DefaultSharedWebContainerContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pax-web-runtime Show documentation
Show all versions of pax-web-runtime Show documentation
Pax Web is a OSGi Http Service based on Jetty 6.
Detailed information to be found at http://wiki.ops4j.org/confluence/x/AYAz.
/* Copyright 2009 David Conde.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ops4j.pax.web.service.internal;
import java.io.IOException;
import java.net.URL;
import java.util.Enumeration;
import java.util.HashSet;
import java.util.Queue;
import java.util.Set;
import java.util.concurrent.ConcurrentLinkedQueue;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.ops4j.pax.web.service.SharedWebContainerContext;
import org.ops4j.pax.web.service.spi.util.Path;
import org.osgi.framework.Bundle;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class DefaultSharedWebContainerContext implements
SharedWebContainerContext {
private static final Logger LOG = LoggerFactory
.getLogger(DefaultSharedWebContainerContext.class);
private Queue bundles = new ConcurrentLinkedQueue();
@Override
public boolean registerBundle(Bundle bundle) {
if (!bundles.contains(bundle)) {
bundles.add(bundle);
return true;
}
return false;
}
@Override
public boolean deregisterBundle(Bundle bundle) {
return bundles.remove(bundle);
}
@Override
public Set getResourcePaths(String path) {
for (Bundle bundle : bundles) {
Set paths = getResourcePaths(bundle, path);
if (paths != null) {
return paths;
}
}
return null;
}
@Override
public String getMimeType(String arg0) {
return null;
}
@Override
public URL getResource(String path) {
for (Bundle bundle : bundles) {
URL pathUrl = getResource(bundle, path);
if (pathUrl != null) {
return pathUrl;
}
}
return null;
}
private URL getResource(Bundle bundle, final String name) {
final String normalizedname = Path.normalizeResourcePath(name);
LOG.debug("Searching bundle [" + bundle + "] for resource ["
+ normalizedname + "]");
return bundle.getResource(normalizedname);
}
private Set getResourcePaths(Bundle bundle, final String name) {
final String normalizedname = Path.normalizeResourcePath(name);
LOG.debug("Searching bundle [" + bundle + "] for resource paths of ["
+ normalizedname + "]");
final Enumeration entryPaths = bundle
.getEntryPaths(normalizedname);
if (entryPaths == null || !entryPaths.hasMoreElements()) {
return null;
}
Set foundPaths = new HashSet();
while (entryPaths.hasMoreElements()) {
foundPaths.add(entryPaths.nextElement());
}
return foundPaths;
}
@Override
public boolean handleSecurity(HttpServletRequest arg0,
HttpServletResponse arg1) throws IOException {
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy