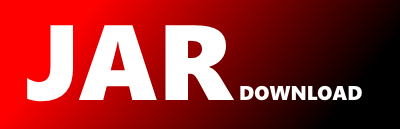
org.apache.wicket.model.Model Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.ops4j.pax.wicket.service Show documentation
Show all versions of org.ops4j.pax.wicket.service Show documentation
Pax Wicket Service is an OSGi extension of the Wicket framework, allowing for dynamic loading and
unloading of Wicket components and pageSources.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.wicket.model;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.wicket.Component;
import org.apache.wicket.WicketRuntimeException;
import org.apache.wicket.model.util.MapModel;
import org.apache.wicket.model.util.WildcardCollectionModel;
import org.apache.wicket.model.util.WildcardListModel;
import org.apache.wicket.model.util.WildcardSetModel;
import org.apache.wicket.util.lang.Objects;
/**
* Model
is the basic implementation of an IModel
. It just wraps a simple
* model object. The model object must be serializable, as it is stored in the session. If you have
* large objects to store, consider using {@link org.apache.wicket.model.LoadableDetachableModel}
* instead of this class.
*
* @author Chris Turner
* @author Eelco Hillenius
*
* @param
* The type of the Model Object
*/
public class Model implements IModel
{
private static final long serialVersionUID = 1L;
/** Backing object. */
private T object;
/**
* Construct the model without providing an object.
*/
public Model()
{
}
/**
* Construct the model, setting the given object as the wrapped object.
*
* @param object
* The model object proper
*/
public Model(final T object)
{
setObject(object);
}
/**
* @param
* type of key inside map
* @param
* type of value inside map
* @param map
* The Map, which may or may not be Serializable
* @deprecated see {@link Model#of(Map)}
* @return A Model object wrapping the Map
*/
@Deprecated
public static IModel
© 2015 - 2025 Weber Informatics LLC | Privacy Policy