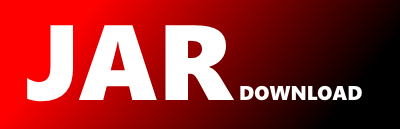
org.apache.wicket.util.resource.ZipResourceStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.ops4j.pax.wicket.service Show documentation
Show all versions of org.ops4j.pax.wicket.service Show documentation
Pax Wicket Service is an OSGi extension of the Wicket framework, allowing for dynamic loading and
unloading of Wicket components and pageSources.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.wicket.util.resource;
import java.io.BufferedInputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
import org.apache.wicket.WicketRuntimeException;
import org.apache.wicket.util.file.File;
import org.apache.wicket.util.time.Time;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* An IResourceStream that ZIPs a directory's contents on the fly
*
*
* NOTE 1. As a future improvement, cache a map of generated ZIP files for every directory
* and use a Watcher to detect modifications in this directory. Using ehcache would be good for
* that, but it's not in Wicket dependencies yet. No caching of the generated ZIP files is done
* yet.
*
*
*
* NOTE 2. As a future improvement, implement getLastModified() and request
* ResourceStreamRequestTarget to generate Last-Modified and Expires HTTP headers. No HTTP cache
* headers are provided yet. See WICKET-385
*
*
* @author Jean-Baptiste Quenot
*/
public class ZipResourceStream extends AbstractResourceStream
{
private static final long serialVersionUID = 1L;
private static final Logger log = LoggerFactory.getLogger(ZipResourceStream.class);
private final ByteArrayOutputStream bytearray;
/**
* Construct.
*
* @param dir
* The directory where to look for files. The directory itself will not be included
* in the ZIP.
* @param recursive
* If true, all subdirs will be zipped as well
*/
public ZipResourceStream(final File dir, final boolean recursive)
{
if ((dir == null) || !dir.isDirectory())
{
throw new IllegalArgumentException("Not a directory: " + dir);
}
bytearray = new ByteArrayOutputStream();
try
{
ZipOutputStream out = new ZipOutputStream(bytearray);
zipDir(dir, out, "", recursive);
}
catch (Exception e)
{
throw new WicketRuntimeException(e);
}
}
/**
* Construct. Until Wicket 1.4-RC3 recursive zip was not supported. In order not to change the
* behavior, using this constructor will default to recursive == false.
*
* @param dir
* The directory where to look for files. The directory itself will not be included
* in the ZIP.
*/
public ZipResourceStream(final File dir)
{
this(dir, false);
}
/**
* Recursive method for zipping the contents of a directory including nested directories.
*
* @param dir
* dir to be zipped
* @param out
* ZipOutputStream to write to
* @param path
* Path to nested dirs (used in resursive calls)
* @param recursive
* If true, all subdirs will be zipped as well
* @throws IOException
*/
private static void zipDir(final File dir, final ZipOutputStream out, final String path,
final boolean recursive) throws IOException
{
if (!dir.isDirectory())
{
throw new IllegalArgumentException("Not a directory: " + dir);
}
String[] files = dir.list();
int BUFFER = 2048;
BufferedInputStream origin = null;
byte data[] = new byte[BUFFER];
for (int i = 0; i < files.length; i++)
{
if (log.isDebugEnabled())
{
log.debug("Adding: " + files[i]);
}
File f = new File(dir, files[i]);
if (f.isDirectory())
{
if (recursive == true)
{
zipDir(f, out, path + f.getName() + "/", recursive);
}
}
else
{
out.putNextEntry(new ZipEntry(path.toString() + f.getName()));
FileInputStream fi = new FileInputStream(f);
origin = new BufferedInputStream(fi, BUFFER);
int count;
while ((count = origin.read(data, 0, BUFFER)) != -1)
{
out.write(data, 0, count);
}
origin.close();
}
}
if (path.equals(""))
{
out.close();
}
}
/**
* @see org.apache.wicket.util.resource.IResourceStream#close()
*/
public void close() throws IOException
{
}
/**
* @see org.apache.wicket.util.resource.IResourceStream#getContentType()
*/
@Override
public String getContentType()
{
return null;
}
/**
* @see org.apache.wicket.util.resource.IResourceStream#getInputStream()
*/
public InputStream getInputStream() throws ResourceStreamNotFoundException
{
return new ByteArrayInputStream(bytearray.toByteArray());
}
/**
* @see org.apache.wicket.util.resource.AbstractResourceStream#length()
*/
@Override
public long length()
{
return bytearray.size();
}
/**
* @see org.apache.wicket.util.resource.AbstractResourceStream#lastModifiedTime()
*/
@Override
public Time lastModifiedTime()
{
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy