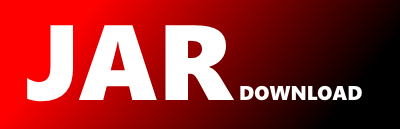
org.apache.wicket.util.string.AppendingStringBuffer Maven / Gradle / Ivy
Show all versions of org.ops4j.pax.wicket.service Show documentation
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.wicket.util.string;
import java.io.IOException;
/**
* This is a copy or combination of java.lang.StringBuffer
and
* java.lang.String
It has a special method getValue() which returns the internal char
* array.
*
* Hashcode and equals methods are also implemented.
*
* This AppendingStringBuffer is not synchronized.
*
* @author Johan Compagner
* @see java.lang.StringBuffer
*/
public final class AppendingStringBuffer implements java.io.Serializable, CharSequence
{
/** use serialVersionUID from JDK 1.0.2 for interoperability */
static final long serialVersionUID = 1L;
private static final AppendingStringBuffer NULL = new AppendingStringBuffer("null");
private static final StringBuffer SB_NULL = new StringBuffer("null");
/**
* The value is used for character storage.
*
* @serial
*/
private char value[];
/**
* The count is the number of characters in the buffer.
*
* @serial
*/
private int count;
/**
* Constructs a string buffer with no characters in it and an initial capacity of 16 characters.
*/
public AppendingStringBuffer()
{
this(16);
}
/**
* Constructs a string buffer with no characters in it and an initial capacity specified by the
* length
argument.
*
* @param length
* the initial capacity.
* @exception NegativeArraySizeException
* if the length
argument is less than 0
.
*/
public AppendingStringBuffer(int length)
{
value = new char[length];
}
/**
* Constructs a string buffer so that it represents the same sequence of characters as the
* string argument; in other words, the initial contents of the string buffer is a copy of the
* argument string. The initial capacity of the string buffer is 16
plus the length
* of the string argument.
*
* @param str
* the initial contents of the buffer.
* @exception NullPointerException
* if str
is null
*/
public AppendingStringBuffer(CharSequence str)
{
this(str.length() + 16);
append(str);
}
/**
* Returns the length (character count) of this string buffer.
*
* @return the length of the sequence of characters currently represented by this string buffer.
*/
public int length()
{
return count;
}
/**
* Returns the current capacity of the String buffer. The capacity is the amount of storage
* available for newly inserted characters; beyond which an allocation will occur.
*
* @return the current capacity of this string buffer.
*/
public int capacity()
{
return value.length;
}
/**
* Ensures that the capacity of the buffer is at least equal to the specified minimum. If the
* current capacity of this string buffer is less than the argument, then a new internal buffer
* is allocated with greater capacity. The new capacity is the larger of:
*
* - The
minimumCapacity
argument.
* - Twice the old capacity, plus
2
.
*
* If the minimumCapacity
argument is nonpositive, this method takes no action and
* simply returns.
*
* @param minimumCapacity
* the minimum desired capacity.
*/
public void ensureCapacity(int minimumCapacity)
{
if (minimumCapacity > value.length)
{
expandCapacity(minimumCapacity);
}
}
/**
* This implements the expansion semantics of ensureCapacity but is unsynchronized for use
* internally by methods which are already synchronized.
*
* @param minimumCapacity
*
* @see java.lang.StringBuffer#ensureCapacity(int)
*/
private void expandCapacity(int minimumCapacity)
{
int newCapacity = (value.length + 1) * 2;
if (newCapacity < 0)
{
newCapacity = Integer.MAX_VALUE;
}
else if (minimumCapacity > newCapacity)
{
newCapacity = minimumCapacity;
}
char newValue[] = new char[newCapacity];
System.arraycopy(value, 0, newValue, 0, count);
value = newValue;
}
/**
* Sets the length of this String buffer. This string buffer is altered to represent a new
* character sequence whose length is specified by the argument. For every nonnegative index
* k less than newLength
, the character at index k in the new
* character sequence is the same as the character at index k in the old sequence if
* k is less than the length of the old character sequence; otherwise, it is the null
* character '\u0000'
.
*
* In other words, if the newLength
argument is less than the current length of the
* string buffer, the string buffer is truncated to contain exactly the number of characters
* given by the newLength
argument.
*
* If the newLength
argument is greater than or equal to the current length,
* sufficient null characters ('\u0000'
) are appended to the string buffer so that length becomes
* the newLength
argument.
*
* The newLength
argument must be greater than or equal to 0
.
*
* @param newLength
* the new length of the buffer.
* @exception IndexOutOfBoundsException
* if the newLength
argument is negative.
* @see java.lang.StringBuffer#length()
*/
public void setLength(int newLength)
{
if (newLength < 0)
{
throw new StringIndexOutOfBoundsException(newLength);
}
if (newLength > value.length)
{
expandCapacity(newLength);
}
if (count < newLength)
{
for (; count < newLength; count++)
{
value[count] = '\0';
}
}
else
{
count = newLength;
}
}
/**
* The specified character of the sequence currently represented by the string buffer, as
* indicated by the index
argument, is returned. The first character of a string
* buffer is at index 0
, the next at index 1
, and so on, for array
* indexing.
*
* The index argument must be greater than or equal to 0
, and less than the length
* of this string buffer.
*
* @param index
* the index of the desired character.
* @return the character at the specified index of this string buffer.
* @exception IndexOutOfBoundsException
* if index
is negative or greater than or equal to
* length()
.
* @see java.lang.StringBuffer#length()
*/
public char charAt(int index)
{
if ((index < 0) || (index >= count))
{
throw new StringIndexOutOfBoundsException(index);
}
return value[index];
}
/**
* Characters are copied from this string buffer into the destination character array
* dst
. The first character to be copied is at index srcBegin
; the
* last character to be copied is at index srcEnd-1
. The total number of characters
* to be copied is srcEnd-srcBegin
. The characters are copied into the subarray of
* dst
starting at index dstBegin
and ending at index:
*
*
*
*
* dstbegin + (srcEnd - srcBegin) - 1
*
*
*
*
* @param srcBegin
* start copying at this offset in the string buffer.
* @param srcEnd
* stop copying at this offset in the string buffer.
* @param dst
* the array to copy the data into.
* @param dstBegin
* offset into dst
.
* @exception NullPointerException
* if dst
is null
.
* @exception IndexOutOfBoundsException
* if any of the following is true:
*
* srcBegin
is negative dstBegin
is negative
* - the
srcBegin
argument is greater than the srcEnd
* argument. srcEnd
is greater than this.length()
,
* the current length of this string buffer. dstBegin+srcEnd-srcBegin
*
is greater than dst.length
*
*/
public void getChars(int srcBegin, int srcEnd, char dst[], int dstBegin)
{
if (srcBegin < 0)
{
throw new StringIndexOutOfBoundsException(srcBegin);
}
if ((srcEnd < 0) || (srcEnd > count))
{
throw new StringIndexOutOfBoundsException(srcEnd);
}
if (srcBegin > srcEnd)
{
throw new StringIndexOutOfBoundsException("srcBegin > srcEnd");
}
System.arraycopy(value, srcBegin, dst, dstBegin, srcEnd - srcBegin);
}
/**
* The character at the specified index of this string buffer is set to ch
. The
* string buffer is altered to represent a new character sequence that is identical to the old
* character sequence, except that it contains the character ch
at position
* index
.
*
* The index argument must be greater than or equal to 0
, and less than the length
* of this string buffer.
*
* @param index
* the index of the character to modify.
* @param ch
* the new character.
* @exception IndexOutOfBoundsException
* if index
is negative or greater than or equal to
* length()
.
* @see java.lang.StringBuffer#length()
*/
public void setCharAt(int index, char ch)
{
if ((index < 0) || (index >= count))
{
throw new StringIndexOutOfBoundsException(index);
}
value[index] = ch;
}
/**
* Appends the string representation of the Object
argument to this string buffer.
*
* The argument is converted to a string as if by the method String.valueOf
, and
* the characters of that string are then appended to this string buffer.
*
* @param obj
* an Object
.
* @return a reference to this AppendingStringBuffer
object.
* @see java.lang.String#valueOf(java.lang.Object)
* @see java.lang.StringBuffer#append(java.lang.String)
*/
public AppendingStringBuffer append(Object obj)
{
if (obj instanceof AppendingStringBuffer)
{
return append((AppendingStringBuffer)obj);
}
else if (obj instanceof StringBuffer)
{
return append((StringBuffer)obj);
}
return append(String.valueOf(obj));
}
/**
* Appends the string to this string buffer.
*
* The characters of the String
argument are appended, in order, to the contents of
* this string buffer, increasing the length of this string buffer by the length of the
* argument. If str
is null
, then the four characters
* "null"
are appended to this string buffer.
*
* Let n be the length of the old character sequence, the one contained in the string
* buffer just prior to execution of the append
method. Then the character at index
* k in the new character sequence is equal to the character at index k in the old
* character sequence, if k is less than n; otherwise, it is equal to the
* character at index k-n in the argument str
.
*
* @param str
* a string.
* @return a reference to this AppendingStringBuffer
.
*/
public AppendingStringBuffer append(String str)
{
if (str == null)
{
str = String.valueOf(str);
}
int len = str.length();
int newcount = count + len;
if (newcount > value.length)
{
expandCapacity(newcount);
}
str.getChars(0, len, value, count);
count = newcount;
return this;
}
/**
* Appends the specified AppendingStringBuffer to this AppendingStringBuffer.
*
* The characters of the AppendingStringBuffer argument are appended, in order, to the
* contents of this AppendingStringBuffer, increasing the length of this
* AppendingStringBuffer by the length of the argument. If sb is null
* , then the four characters "null" are appended to this
* AppendingStringBuffer.
*
* Let n be the length of the old character sequence, the one contained in the
* AppendingStringBuffer just prior to execution of the append method. Then
* the character at index k in the new character sequence is equal to the character at
* index k in the old character sequence, if k is less than n; otherwise,
* it is equal to the character at index k-n in the argument sb
.
*
* The method ensureCapacity is first called on this AppendingStringBuffer
* with the new buffer length as its argument. (This ensures that the storage of this
* AppendingStringBuffer is adequate to contain the additional characters being
* appended.)
*
* @param sb
* the AppendingStringBuffer to append.
* @return a reference to this AppendingStringBuffer.
* @since 1.4
*/
public AppendingStringBuffer append(AppendingStringBuffer sb)
{
if (sb == null)
{
sb = NULL;
}
int len = sb.length();
int newcount = count + len;
if (newcount > value.length)
{
expandCapacity(newcount);
}
sb.getChars(0, len, value, count);
count = newcount;
return this;
}
/**
* Appends the specified AppendingStringBuffer to this AppendingStringBuffer.
*
* The characters of the AppendingStringBuffer argument are appended, in order, to the
* contents of this AppendingStringBuffer, increasing the length of this
* AppendingStringBuffer by the length of the argument. If sb is null
* , then the four characters "null" are appended to this
* AppendingStringBuffer.
*
* Let n be the length of the old character sequence, the one contained in the
* AppendingStringBuffer just prior to execution of the append method. Then
* the character at index k in the new character sequence is equal to the character at
* index k in the old character sequence, if k is less than n; otherwise,
* it is equal to the character at index k-n in the argument sb
.
*
* The method ensureCapacity is first called on this AppendingStringBuffer
* with the new buffer length as its argument. (This ensures that the storage of this
* AppendingStringBuffer is adequate to contain the additional characters being
* appended.)
*
* @param sb
* the AppendingStringBuffer to append.
* @return a reference to this AppendingStringBuffer.
* @since 1.4
*/
public AppendingStringBuffer append(StringBuffer sb)
{
if (sb == null)
{
sb = SB_NULL;
}
int len = sb.length();
int newcount = count + len;
if (newcount > value.length)
{
expandCapacity(newcount);
}
sb.getChars(0, len, value, count);
count = newcount;
return this;
}
/**
* Appends the specified AppendingStringBuffer to this AppendingStringBuffer.
*
* The characters of the AppendingStringBuffer argument are appended, in order, to the
* contents of this AppendingStringBuffer, increasing the length of this
* AppendingStringBuffer by the length of the argument. If sb is null
* , then the four characters "null" are appended to this
* AppendingStringBuffer.
*
* Let n be the length of the old character sequence, the one contained in the
* AppendingStringBuffer just prior to execution of the append method. Then
* the character at index k in the new character sequence is equal to the character at
* index k in the old character sequence, if k is less than n; otherwise,
* it is equal to the character at index k-n in the argument sb
.
*
* The method ensureCapacity is first called on this AppendingStringBuffer
* with the new buffer length as its argument. (This ensures that the storage of this
* AppendingStringBuffer is adequate to contain the additional characters being
* appended.)
*
* @param sb
* the AppendingStringBuffer to append.
* @param from
* The index where it must start from
* @param length
* The length that must be copied
* @return a reference to this AppendingStringBuffer.
*/
public AppendingStringBuffer append(StringBuffer sb, int from, int length)
{
if (sb == null)
{
sb = SB_NULL;
}
int newcount = count + length;
if (newcount > value.length)
{
expandCapacity(newcount);
}
sb.getChars(from, length, value, count);
count = newcount;
return this;
}
/**
* Appends the string representation of the char
array argument to this string
* buffer.
*
* The characters of the array argument are appended, in order, to the contents of this string
* buffer. The length of this string buffer increases by the length of the argument.
*
* The overall effect is exactly as if the argument were converted to a string by the method
* {@link String#valueOf(char[])} and the characters of that string were then
* {@link #append(String) appended} to this AppendingStringBuffer
object.
*
* @param str
* the characters to be appended.
* @return a reference to this AppendingStringBuffer
object.
*/
public AppendingStringBuffer append(char str[])
{
int len = str.length;
int newcount = count + len;
if (newcount > value.length)
{
expandCapacity(newcount);
}
System.arraycopy(str, 0, value, count, len);
count = newcount;
return this;
}
/**
* Appends the string representation of a subarray of the char
array argument to
* this string buffer.
*
* Characters of the character array str
, starting at index offset
,
* are appended, in order, to the contents of this string buffer. The length of this string
* buffer increases by the value of len
.
*
* The overall effect is exactly as if the arguments were converted to a string by the method
* {@link String#valueOf(char[],int,int)} and the characters of that string were then
* {@link #append(String) appended} to this AppendingStringBuffer
object.
*
* @param str
* the characters to be appended.
* @param offset
* the index of the first character to append.
* @param len
* the number of characters to append.
* @return a reference to this AppendingStringBuffer
object.
*/
public AppendingStringBuffer append(char str[], int offset, int len)
{
int newcount = count + len;
if (newcount > value.length)
{
expandCapacity(newcount);
}
System.arraycopy(str, offset, value, count, len);
count = newcount;
return this;
}
/**
* Appends the string representation of the boolean
argument to the string buffer.
*
* The argument is converted to a string as if by the method String.valueOf
, and
* the characters of that string are then appended to this string buffer.
*
* @param b
* a boolean
.
* @return a reference to this AppendingStringBuffer
.
* @see java.lang.String#valueOf(boolean)
* @see java.lang.StringBuffer#append(java.lang.String)
*/
public AppendingStringBuffer append(boolean b)
{
if (b)
{
int newcount = count + 4;
if (newcount > value.length)
{
expandCapacity(newcount);
}
value[count++] = 't';
value[count++] = 'r';
value[count++] = 'u';
value[count++] = 'e';
}
else
{
int newcount = count + 5;
if (newcount > value.length)
{
expandCapacity(newcount);
}
value[count++] = 'f';
value[count++] = 'a';
value[count++] = 'l';
value[count++] = 's';
value[count++] = 'e';
}
return this;
}
/**
* Appends the string representation of the char
argument to this string buffer.
*
* The argument is appended to the contents of this string buffer. The length of this string
* buffer increases by 1
.
*
* The overall effect is exactly as if the argument were converted to a string by the method
* {@link String#valueOf(char)} and the character in that string were then
* {@link #append(String) appended} to this AppendingStringBuffer
object.
*
* @param c
* a char
.
* @return a reference to this AppendingStringBuffer
object.
*/
public AppendingStringBuffer append(char c)
{
int newcount = count + 1;
if (newcount > value.length)
{
expandCapacity(newcount);
}
value[count++] = c;
return this;
}
/**
* Appends the string representation of the int
argument to this string buffer.
*
* The argument is converted to a string as if by the method String.valueOf
, and
* the characters of that string are then appended to this string buffer.
*
* @param i
* an int
.
* @return a reference to this AppendingStringBuffer
object.
* @see java.lang.String#valueOf(int)
* @see java.lang.StringBuffer#append(java.lang.String)
*/
public AppendingStringBuffer append(int i)
{
return append(String.valueOf(i));
}
/**
* Appends the string representation of the long
argument to this string buffer.
*
* The argument is converted to a string as if by the method String.valueOf
, and
* the characters of that string are then appended to this string buffer.
*
* @param l
* a long
.
* @return a reference to this AppendingStringBuffer
object.
* @see java.lang.String#valueOf(long)
* @see java.lang.StringBuffer#append(java.lang.String)
*/
public AppendingStringBuffer append(long l)
{
return append(String.valueOf(l));
}
/**
* Appends the string representation of the float
argument to this string buffer.
*
* The argument is converted to a string as if by the method String.valueOf
, and
* the characters of that string are then appended to this string buffer.
*
* @param f
* a float
.
* @return a reference to this AppendingStringBuffer
object.
* @see java.lang.String#valueOf(float)
* @see java.lang.StringBuffer#append(java.lang.String)
*/
public AppendingStringBuffer append(float f)
{
return append(String.valueOf(f));
}
/**
* Appends the string representation of the double
argument to this string buffer.
*
* The argument is converted to a string as if by the method String.valueOf
, and
* the characters of that string are then appended to this string buffer.
*
* @param d
* a double
.
* @return a reference to this AppendingStringBuffer
object.
* @see java.lang.String#valueOf(double)
* @see java.lang.StringBuffer#append(java.lang.String)
*/
public AppendingStringBuffer append(double d)
{
return append(String.valueOf(d));
}
/**
* Removes the characters in a substring of this AppendingStringBuffer
. The
* substring begins at the specified start
and extends to the character at index
* end - 1
or to the end of the AppendingStringBuffer
if no such
* character exists. If start
is equal to end
, no changes are made.
*
* @param start
* The beginning index, inclusive.
* @param end
* The ending index, exclusive.
* @return This string buffer.
* @exception StringIndexOutOfBoundsException
* if start
is negative, greater than length()
, or
* greater than end
.
* @since 1.2
*/
public AppendingStringBuffer delete(int start, int end)
{
if (start < 0)
{
throw new StringIndexOutOfBoundsException(start);
}
if (end > count)
{
end = count;
}
if (start > end)
{
throw new StringIndexOutOfBoundsException();
}
int len = end - start;
if (len > 0)
{
System.arraycopy(value, start + len, value, start, count - end);
count -= len;
}
return this;
}
/**
* Removes the character at the specified position in this AppendingStringBuffer
* (shortening the AppendingStringBuffer
by one character).
*
* @param index
* Index of character to remove
* @return This string buffer.
* @exception StringIndexOutOfBoundsException
* if the index
is negative or greater than or equal to
* length()
.
* @since 1.2
*/
public AppendingStringBuffer deleteCharAt(int index)
{
if ((index < 0) || (index >= count))
{
throw new StringIndexOutOfBoundsException();
}
System.arraycopy(value, index + 1, value, index, count - index - 1);
count--;
return this;
}
/**
* Replaces the characters in a substring of this AppendingStringBuffer
with
* characters in the specified String
. The substring begins at the specified
* start
and extends to the character at index end - 1
or to the end
* of the AppendingStringBuffer
if no such character exists. First the characters
* in the substring are removed and then the specified String
is inserted at
* start
. (The AppendingStringBuffer
will be lengthened to accommodate
* the specified String if necessary.)
*
* @param start
* The beginning index, inclusive.
* @param end
* The ending index, exclusive.
* @param str
* String that will replace previous contents.
* @return This string buffer.
* @exception StringIndexOutOfBoundsException
* if start
is negative, greater than length()
, or
* greater than end
.
* @since 1.2
*/
public AppendingStringBuffer replace(int start, int end, String str)
{
if (start < 0)
{
throw new StringIndexOutOfBoundsException(start);
}
if (end > count)
{
end = count;
}
if (start > end)
{
throw new StringIndexOutOfBoundsException();
}
int len = str.length();
int newCount = count + len - (end - start);
if (newCount > value.length)
{
expandCapacity(newCount);
}
System.arraycopy(value, end, value, start + len, count - end);
str.getChars(0, len, value, start);
count = newCount;
return this;
}
/**
* Returns a new String
that contains a subsequence of characters currently
* contained in this AppendingStringBuffer
.The substring begins at the specified
* index and extends to the end of the AppendingStringBuffer
.
*
* @param start
* The beginning index, inclusive.
* @return The new string.
* @exception StringIndexOutOfBoundsException
* if start
is less than zero, or greater than the length of this
* AppendingStringBuffer
.
* @since 1.2
*/
public String substring(int start)
{
return substring(start, count);
}
/**
* Returns a new character sequence that is a subsequence of this sequence.
*
*
* An invocation of this method of the form
*
*
*
*
* sb.subSequence(begin, end)
*
*
*
*
* behaves in exactly the same way as the invocation
*
*
*
*
* sb.substring(begin, end)
*
*
*
*
* This method is provided so that the AppendingStringBuffer class can implement the
* {@link CharSequence} interface.
*
*
* @param start
* the start index, inclusive.
* @param end
* the end index, exclusive.
* @return the specified subsequence.
*
* @throws IndexOutOfBoundsException
* if start or end are negative, if end is greater than
* length(), or if start is greater than end
*
* @since 1.4
* @spec JSR-51
*/
public CharSequence subSequence(int start, int end)
{
return this.substring(start, end);
}
/**
* Returns a new String
that contains a subsequence of characters currently
* contained in this AppendingStringBuffer
. The substring begins at the specified
* start
and extends to the character at index end - 1
. An exception
* is thrown if
*
* @param start
* The beginning index, inclusive.
* @param end
* The ending index, exclusive.
* @return The new string.
* @exception StringIndexOutOfBoundsException
* if start
or end
are negative or greater than
* length()
, or start
is greater than end
.
* @since 1.2
*/
public String substring(int start, int end)
{
if (start < 0)
{
throw new StringIndexOutOfBoundsException(start);
}
if (end > count)
{
throw new StringIndexOutOfBoundsException(end);
}
if (start > end)
{
throw new StringIndexOutOfBoundsException(end - start);
}
return new String(value, start, end - start);
}
/**
* Inserts the string representation of a subarray of the str
array argument into
* this string buffer. The subarray begins at the specified offset
and extends
* len
characters. The characters of the subarray are inserted into this string
* buffer at the position indicated by index
. The length of this
* AppendingStringBuffer
increases by len
characters.
*
* @param index
* position at which to insert subarray.
* @param str
* A character array.
* @param offset
* the index of the first character in subarray to to be inserted.
* @param len
* the number of characters in the subarray to to be inserted.
* @return This string buffer.
* @exception StringIndexOutOfBoundsException
* if index
is negative or greater than length()
, or
* offset
or len
are negative, or
* (offset+len)
is greater than str.length
.
* @since 1.2
*/
public AppendingStringBuffer insert(int index, char str[], int offset, int len)
{
if ((index < 0) || (index > count))
{
throw new StringIndexOutOfBoundsException();
}
if ((offset < 0) || (offset + len < 0) || (offset + len > str.length))
{
throw new StringIndexOutOfBoundsException(offset);
}
if (len < 0)
{
throw new StringIndexOutOfBoundsException(len);
}
int newCount = count + len;
if (newCount > value.length)
{
expandCapacity(newCount);
}
System.arraycopy(value, index, value, index + len, count - index);
System.arraycopy(str, offset, value, index, len);
count = newCount;
return this;
}
/**
* Inserts the string representation of the Object
argument into this string
* buffer.
*
* The second argument is converted to a string as if by the method String.valueOf
,
* and the characters of that string are then inserted into this string buffer at the indicated
* offset.
*
* The offset argument must be greater than or equal to 0
, and less than or equal
* to the length of this string buffer.
*
* @param offset
* the offset.
* @param obj
* an Object
.
* @return a reference to this AppendingStringBuffer
object.
* @exception StringIndexOutOfBoundsException
* if the offset is invalid.
* @see java.lang.String#valueOf(java.lang.Object)
* @see AppendingStringBuffer#insert(int, java.lang.String)
* @see AppendingStringBuffer#length()
*/
public AppendingStringBuffer insert(int offset, Object obj)
{
if (obj instanceof AppendingStringBuffer)
{
AppendingStringBuffer asb = (AppendingStringBuffer)obj;
return insert(offset, asb.value, 0, asb.count);
}
else if (obj instanceof StringBuffer)
{
return insert(offset, (StringBuffer)obj);
}
return insert(offset, String.valueOf(obj));
}
/**
* Inserts the string into this string buffer.
*
* The characters of the String
argument are inserted, in order, into this string
* buffer at the indicated offset, moving up any characters originally above that position and
* increasing the length of this string buffer by the length of the argument. If
* str
is null
, then the four characters "null"
are
* inserted into this string buffer.
*
* The character at index k in the new character sequence is equal to:
*
* - the character at index k in the old character sequence, if k is less than
*
offset
* - the character at index k
-offset
in the argument str
, if
* k is not less than offset
but is less than
* offset+str.length()
* - the character at index k
-str.length()
in the old character sequence,
* if k is not less than offset+str.length()
*
*
* The offset argument must be greater than or equal to 0
, and less than or equal
* to the length of this string buffer.
*
* @param offset
* the offset.
* @param str
* a string.
* @return a reference to this AppendingStringBuffer
object.
* @exception StringIndexOutOfBoundsException
* if the offset is invalid.
* @see java.lang.StringBuffer#length()
*/
public AppendingStringBuffer insert(int offset, String str)
{
if ((offset < 0) || (offset > count))
{
throw new StringIndexOutOfBoundsException();
}
if (str == null)
{
str = String.valueOf(str);
}
int len = str.length();
int newcount = count + len;
if (newcount > value.length)
{
expandCapacity(newcount);
}
System.arraycopy(value, offset, value, offset + len, count - offset);
str.getChars(0, len, value, offset);
count = newcount;
return this;
}
/**
* Inserts the string into this string buffer.
*
* The characters of the String
argument are inserted, in order, into this string
* buffer at the indicated offset, moving up any characters originally above that position and
* increasing the length of this string buffer by the length of the argument. If
* str
is null
, then the four characters "null"
are
* inserted into this string buffer.
*
* The character at index k in the new character sequence is equal to:
*
* - the character at index k in the old character sequence, if k is less than
*
offset
* - the character at index k
-offset
in the argument str
, if
* k is not less than offset
but is less than
* offset+str.length()
* - the character at index k
-str.length()
in the old character sequence,
* if k is not less than offset+str.length()
*
*
* The offset argument must be greater than or equal to 0
, and less than or equal
* to the length of this string buffer.
*
* @param offset
* the offset.
* @param str
* a string.
* @return a reference to this AppendingStringBuffer
object.
* @exception StringIndexOutOfBoundsException
* if the offset is invalid.
* @see java.lang.StringBuffer#length()
*/
public AppendingStringBuffer insert(int offset, StringBuffer str)
{
if ((offset < 0) || (offset > count))
{
throw new StringIndexOutOfBoundsException();
}
if (str == null)
{
str = SB_NULL;
}
int len = str.length();
int newcount = count + len;
if (newcount > value.length)
{
expandCapacity(newcount);
}
System.arraycopy(value, offset, value, offset + len, count - offset);
str.getChars(0, len, value, offset);
count = newcount;
return this;
}
/**
* Inserts the string representation of the char
array argument into this string
* buffer.
*
* The characters of the array argument are inserted into the contents of this string buffer at
* the position indicated by offset
. The length of this string buffer increases by
* the length of the argument.
*
* The overall effect is exactly as if the argument were converted to a string by the method
* {@link String#valueOf(char[])} and the characters of that string were then
* {@link #insert(int,String) inserted} into this AppendingStringBuffer
object at
* the position indicated by offset
.
*
* @param offset
* the offset.
* @param str
* a character array.
* @return a reference to this AppendingStringBuffer
object.
* @exception StringIndexOutOfBoundsException
* if the offset is invalid.
*/
public AppendingStringBuffer insert(int offset, char str[])
{
if ((offset < 0) || (offset > count))
{
throw new StringIndexOutOfBoundsException();
}
int len = str.length;
int newcount = count + len;
if (newcount > value.length)
{
expandCapacity(newcount);
}
System.arraycopy(value, offset, value, offset + len, count - offset);
System.arraycopy(str, 0, value, offset, len);
count = newcount;
return this;
}
/**
* Inserts the string representation of the boolean
argument into this string
* buffer.
*
* The second argument is converted to a string as if by the method String.valueOf
,
* and the characters of that string are then inserted into this string buffer at the indicated
* offset.
*
* The offset argument must be greater than or equal to 0
, and less than or equal
* to the length of this string buffer.
*
* @param offset
* the offset.
* @param b
* a boolean
.
* @return a reference to this AppendingStringBuffer
object.
* @exception StringIndexOutOfBoundsException
* if the offset is invalid.
* @see java.lang.String#valueOf(boolean)
* @see java.lang.StringBuffer#insert(int, java.lang.String)
* @see java.lang.StringBuffer#length()
*/
public AppendingStringBuffer insert(int offset, boolean b)
{
return insert(offset, String.valueOf(b));
}
/**
* Inserts the string representation of the char
argument into this string buffer.
*
* The second argument is inserted into the contents of this string buffer at the position
* indicated by offset
. The length of this string buffer increases by one.
*
* The overall effect is exactly as if the argument were converted to a string by the method
* {@link String#valueOf(char)} and the character in that string were then
* {@link #insert(int, String) inserted} into this AppendingStringBuffer
object at
* the position indicated by offset
.
*
* The offset argument must be greater than or equal to 0
, and less than or equal
* to the length of this string buffer.
*
* @param offset
* the offset.
* @param c
* a char
.
* @return a reference to this AppendingStringBuffer
object.
* @exception IndexOutOfBoundsException
* if the offset is invalid.
* @see java.lang.StringBuffer#length()
*/
public AppendingStringBuffer insert(int offset, char c)
{
int newcount = count + 1;
if (newcount > value.length)
{
expandCapacity(newcount);
}
System.arraycopy(value, offset, value, offset + 1, count - offset);
value[offset] = c;
count = newcount;
return this;
}
/**
* Inserts the string representation of the second int
argument into this string
* buffer.
*
* The second argument is converted to a string as if by the method String.valueOf
,
* and the characters of that string are then inserted into this string buffer at the indicated
* offset.
*
* The offset argument must be greater than or equal to 0
, and less than or equal
* to the length of this string buffer.
*
* @param offset
* the offset.
* @param i
* an int
.
* @return a reference to this AppendingStringBuffer
object.
* @exception StringIndexOutOfBoundsException
* if the offset is invalid.
* @see java.lang.String#valueOf(int)
* @see java.lang.StringBuffer#insert(int, java.lang.String)
* @see java.lang.StringBuffer#length()
*/
public AppendingStringBuffer insert(int offset, int i)
{
return insert(offset, String.valueOf(i));
}
/**
* Inserts the string representation of the long
argument into this string buffer.
*
* The second argument is converted to a string as if by the method String.valueOf
,
* and the characters of that string are then inserted into this string buffer at the position
* indicated by offset
.
*
* The offset argument must be greater than or equal to 0
, and less than or equal
* to the length of this string buffer.
*
* @param offset
* the offset.
* @param l
* a long
.
* @return a reference to this AppendingStringBuffer
object.
* @exception StringIndexOutOfBoundsException
* if the offset is invalid.
* @see java.lang.String#valueOf(long)
* @see java.lang.StringBuffer#insert(int, java.lang.String)
* @see java.lang.StringBuffer#length()
*/
public AppendingStringBuffer insert(int offset, long l)
{
return insert(offset, String.valueOf(l));
}
/**
* Inserts the string representation of the float
argument into this string buffer.
*
* The second argument is converted to a string as if by the method String.valueOf
,
* and the characters of that string are then inserted into this string buffer at the indicated
* offset.
*
* The offset argument must be greater than or equal to 0
, and less than or equal
* to the length of this string buffer.
*
* @param offset
* the offset.
* @param f
* a float
.
* @return a reference to this AppendingStringBuffer
object.
* @exception StringIndexOutOfBoundsException
* if the offset is invalid.
* @see java.lang.String#valueOf(float)
* @see java.lang.StringBuffer#insert(int, java.lang.String)
* @see java.lang.StringBuffer#length()
*/
public AppendingStringBuffer insert(int offset, float f)
{
return insert(offset, String.valueOf(f));
}
/**
* Inserts the string representation of the double
argument into this string
* buffer.
*
* The second argument is converted to a string as if by the method String.valueOf
,
* and the characters of that string are then inserted into this string buffer at the indicated
* offset.
*
* The offset argument must be greater than or equal to 0
, and less than or equal
* to the length of this string buffer.
*
* @param offset
* the offset.
* @param d
* a double
.
* @return a reference to this AppendingStringBuffer
object.
* @exception StringIndexOutOfBoundsException
* if the offset is invalid.
* @see java.lang.String#valueOf(double)
* @see java.lang.StringBuffer#insert(int, java.lang.String)
* @see java.lang.StringBuffer#length()
*/
public AppendingStringBuffer insert(int offset, double d)
{
return insert(offset, String.valueOf(d));
}
/**
* Returns the index within this string of the first occurrence of the specified substring. The
* integer returned is the smallest value k such that:
*
*
* this.toString().startsWith(str, <i>k</i>)
*
*
*
is true
.
*
* @param str
* any string.
* @return if the string argument occurs as a substring within this object, then the index of
* the first character of the first such substring is returned; if it does not occur as
* a substring, -1
is returned.
* @exception java.lang.NullPointerException
* if str
is null
.
* @since 1.4
*/
public int indexOf(String str)
{
return indexOf(str, 0);
}
/**
* Returns the index within this string of the first occurrence of the specified substring,
* starting at the specified index. The integer returned is the smallest value k for
* which:
*
*
* k >= Math.min(fromIndex, str.length()) && this.toString().startsWith(str, k)
*
*
*
If no such value of k exists, then -1 is returned.
*
* @param str
* the substring for which to search.
* @param fromIndex
* the index from which to start the search.
* @return the index within this string of the first occurrence of the specified substring,
* starting at the specified index.
* @exception java.lang.NullPointerException
* if str
is null
.
* @since 1.4
*/
public int indexOf(String str, int fromIndex)
{
return indexOf(value, 0, count, str.toCharArray(), 0, str.length(), fromIndex);
}
static int indexOf(char[] source, int sourceOffset, int sourceCount, char[] target,
int targetOffset, int targetCount, int fromIndex)
{
if (fromIndex >= sourceCount)
{
return (targetCount == 0 ? sourceCount : -1);
}
if (fromIndex < 0)
{
fromIndex = 0;
}
if (targetCount == 0)
{
return fromIndex;
}
char first = target[targetOffset];
int i = sourceOffset + fromIndex;
int max = sourceOffset + (sourceCount - targetCount);
startSearchForFirstChar : while (true)
{
/* Look for first character. */
while (i <= max && source[i] != first)
{
i++;
}
if (i > max)
{
return -1;
}
/* Found first character, now look at the rest of v2 */
int j = i + 1;
int end = j + targetCount - 1;
int k = targetOffset + 1;
while (j < end)
{
if (source[j++] != target[k++])
{
i++;
/* Look for str's first char again. */
continue startSearchForFirstChar;
}
}
return i - sourceOffset; /* Found whole string. */
}
}
/**
* Returns the index within this string of the rightmost occurrence of the specified substring.
* The rightmost empty string "" is considered to occur at the index value
* this.length()
. The returned index is the largest value k such that
*
*
*
* this.toString().startsWith(str, k)
*
*
*
is true.
*
* @param str
* the substring to search for.
* @return if the string argument occurs one or more times as a substring within this object,
* then the index of the first character of the last such substring is returned. If it
* does not occur as a substring, -1
is returned.
* @exception java.lang.NullPointerException
* if str
is null
.
* @since 1.4
*/
public int lastIndexOf(String str)
{
return lastIndexOf(str, count);
}
/**
* Returns the index within this string of the last occurrence of the specified substring. The
* integer returned is the largest value k such that:
*
*
* k <= Math.min(fromIndex, str.length()) && this.toString().startsWith(str, k)
*
*
*
If no such value of k exists, then -1 is returned.
*
* @param str
* the substring to search for.
* @param fromIndex
* the index to start the search from.
* @return the index within this string of the last occurrence of the specified substring.
* @exception java.lang.NullPointerException
* if str
is null
.
* @since 1.4
*/
public int lastIndexOf(String str, int fromIndex)
{
return lastIndexOf(value, 0, count, str.toCharArray(), 0, str.length(), fromIndex);
}
static int lastIndexOf(char[] source, int sourceOffset, int sourceCount, char[] target,
int targetOffset, int targetCount, int fromIndex)
{
/*
* Check arguments; return immediately where possible. For consistency, don't check for null
* str.
*/
int rightIndex = sourceCount - targetCount;
if (fromIndex < 0)
{
return -1;
}
if (fromIndex > rightIndex)
{
fromIndex = rightIndex;
}
/* Empty string always matches. */
if (targetCount == 0)
{
return fromIndex;
}
int strLastIndex = targetOffset + targetCount - 1;
char strLastChar = target[strLastIndex];
int min = sourceOffset + targetCount - 1;
int i = min + fromIndex;
startSearchForLastChar : while (true)
{
while (i >= min && source[i] != strLastChar)
{
i--;
}
if (i < min)
{
return -1;
}
int j = i - 1;
int start = j - (targetCount - 1);
int k = strLastIndex - 1;
while (j > start)
{
if (source[j--] != target[k--])
{
i--;
continue startSearchForLastChar;
}
}
return start - sourceOffset + 1;
}
}
/**
* Tests if this AppendingStringBuffer starts with the specified prefix beginning a specified
* index.
*
* @param prefix
* the prefix.
* @param toffset
* where to begin looking in the string.
* @return true
if the character sequence represented by the argument is a prefix
* of the substring of this object starting at index toffset
;
* false
otherwise. The result is false
if
* toffset
is negative or greater than the length of this
* String
object; otherwise the result is the same as the result of the
* expression
*
*
* this.subString(toffset).startsWith(prefix)
*
*/
public boolean startsWith(CharSequence prefix, int toffset)
{
char ta[] = value;
int to = toffset;
int po = 0;
int pc = prefix.length();
// Note: toffset might be near -1>>>1.
if ((toffset < 0) || (toffset > count - pc))
{
return false;
}
while (--pc >= 0)
{
if (ta[to++] != prefix.charAt(po++))
{
return false;
}
}
return true;
}
/**
* Tests if this AppendingStringBuffer starts with the specified prefix.
*
* @param prefix
* the prefix.
* @return true
if the character sequence represented by the argument is a prefix
* of the character sequence represented by this AppendingStringBuffer;
* false
otherwise. Note also that true
will be returned if
* the argument is an empty string or is equal to this
* AppendingStringBuffer
object as determined by the
* {@link #equals(Object)} method.
* @since 1. 0
*/
public boolean startsWith(CharSequence prefix)
{
return startsWith(prefix, 0);
}
/**
* Tests if this AppendingStringBuffer ends with the specified suffix.
*
* @param suffix
* the suffix.
* @return true
if the character sequence represented by the argument is a suffix
* of the character sequence represented by this AppendingStringBuffer;
* false
otherwise. Note that the result will be true
if the
* argument is the empty string or is equal to this AppendingStringBuffer
* object as determined by the {@link #equals(Object)} method.
*/
public boolean endsWith(CharSequence suffix)
{
return startsWith(suffix, count - suffix.length());
}
/**
* Converts to a string representing the data in this AppendingStringBuffer. A new
* String
object is allocated and initialized to contain the character sequence
* currently represented by this string buffer. This String
is then returned.
* Subsequent changes to the string buffer do not affect the contents of the String
* .
*
* Implementation advice: This method can be coded so as to create a new String
* object without allocating new memory to hold a copy of the character sequence. Instead, the
* string can share the memory used by the string buffer. Any subsequent operation that alters
* the content or capacity of the string buffer must then make a copy of the internal buffer at
* that time. This strategy is effective for reducing the amount of memory allocated by a string
* concatenation operation when it is implemented using a string buffer.
*
* @return a string representation of the string buffer.
*/
@Override
public String toString()
{
return new String(value, 0, count);
}
/**
* This method returns the internal char array. So it is not
*
* @return The internal char array
*/
public final char[] getValue()
{
return value;
}
/**
* readObject is called to restore the state of the AppendingStringBuffer from a stream.
*
* @param s
* @throws ClassNotFoundException
* @throws IOException
*/
private void readObject(java.io.ObjectInputStream s) throws IOException, ClassNotFoundException
{
s.defaultReadObject();
value = value.clone();
}
/**
* Compares this AppendingStringBuffer to the specified object. The result is true
* if and only if the argument is not null
and is a
* AppendingStringBuffer
object or another charsequence object! that represents the
* same sequence of characters as this object.
*
* @param anObject
* the object to compare this AppendingStringBuffer
against.
* @return true
if the AppendingStringBuffer
are equal;
* false
otherwise.
*/
@Override
public boolean equals(Object anObject)
{
if (this == anObject)
{
return true;
}
if (anObject instanceof AppendingStringBuffer)
{
AppendingStringBuffer anotherString = (AppendingStringBuffer)anObject;
int n = count;
if (n == anotherString.count)
{
char v1[] = value;
char v2[] = anotherString.value;
int i = 0;
while (n-- != 0)
{
if (v1[i] != v2[i++])
{
return false;
}
}
return true;
}
}
else if (anObject instanceof CharSequence)
{
CharSequence sequence = (CharSequence)anObject;
int n = count;
if (sequence.length() == count)
{
char v1[] = value;
int i = 0;
while (n-- != 0)
{
if (v1[i] != sequence.charAt(i++))
{
return false;
}
}
return true;
}
}
return false;
}
/**
* Returns a hash code for this AppendingStringBuffer. The hash code for a
* AppendingStringBuffer
object is computed as
*
*
* s[0]*31ˆ(n-1) + s[1]*31ˆ(n-2) + ... + s[n-1]
*
*
*
using int
arithmetic, where s[i]
is the ith
* character of the AppendingStringBuffer, n
is the length of the
* AppendingStringBuffer, and ^
indicates exponentiation. (The hash value of the
* empty AppendingStringBuffer is zero.)
*
* @return a hash code value for this object.
*/
@Override
public int hashCode()
{
int h = 0;
if (h == 0)
{
int off = 0;
char val[] = value;
int len = count;
for (int i = 0; i < len; i++)
{
h = 31 * h + val[off++];
}
}
return h;
}
/**
* Clears the buffer contents, but leaves the allocated size intact
*/
public void clear()
{
count = 0;
}
}