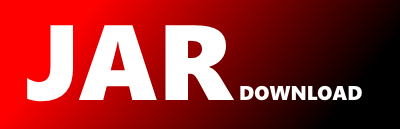
org.apache.wicket.util.tester.WicketTesterHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.ops4j.pax.wicket.service Show documentation
Show all versions of org.ops4j.pax.wicket.service Show documentation
Pax Wicket Service is an OSGi extension of the Wicket framework, allowing for dynamic loading and
unloading of Wicket components and pageSources.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.wicket.util.tester;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import junit.framework.Assert;
import org.apache.wicket.Component;
import org.apache.wicket.IClusterable;
import org.apache.wicket.Page;
import org.apache.wicket.Component.IVisitor;
import org.apache.wicket.util.string.Strings;
/**
* A WicketTester
-specific helper class.
*
* @author Ingram Chen
* @since 1.2.6
*/
public class WicketTesterHelper
{
/**
* ComponentData
class.
*
* @author Ingram Chen
* @since 1.2.6
*/
public static class ComponentData implements IClusterable
{
private static final long serialVersionUID = 1L;
/** Component path. */
public String path;
/** Component type. */
public String type;
/** Component value. */
public String value;
}
/**
* Gets recursively all Component
s of a given Page
, extracts the
* information relevant to us, and adds them to a List
.
*
* @param page
* the Page
to analyze
* @return a List
of Component
data objects
*/
public static List getComponentData(final Page page)
{
final List data = new ArrayList();
if (page != null)
{
page.visitChildren(new IVisitor()
{
public Object component(final Component component)
{
final ComponentData object = new ComponentData();
// anonymous class? Get the parent's class name
String name = component.getClass().getName();
if (name.indexOf("$") > 0)
{
name = component.getClass().getSuperclass().getName();
}
// remove the path component
name = Strings.lastPathComponent(name, Component.PATH_SEPARATOR);
object.path = component.getPageRelativePath();
object.type = name;
try
{
object.value = component.getDefaultModelObjectAsString();
}
catch (Exception e)
{
object.value = e.getMessage();
}
data.add(object);
return IVisitor.CONTINUE_TRAVERSAL;
}
});
}
return data;
}
/**
* Asserts that both Collection
s contain the same elements.
*
* @param expects
* a Collection
object
* @param actuals
* a Collection
object
*/
public static void assertEquals(final Collection> expects, final Collection> actuals)
{
if ((actuals.size() != expects.size()) || !expects.containsAll(actuals) ||
!actuals.containsAll(expects))
{
failWithVerboseMessage(expects, actuals);
}
}
/**
* Fails with a verbose error message.
*
* @param expects
* a Collection
object
* @param actuals
* a Collection
object
*/
public static void failWithVerboseMessage(final Collection> expects,
final Collection> actuals)
{
Assert.fail("\nexpect (" + expects.size() + "):\n" + asLined(expects) + "\nbut was (" +
actuals.size() + "):\n" + asLined(actuals));
}
/**
* A toString
method for the given Collection
.
*
* @param objects
* a Collection
object
* @return a String
representation of the Collection
*/
public static String asLined(final Collection> objects)
{
StringBuffer lined = new StringBuffer();
for (Iterator> iter = objects.iterator(); iter.hasNext();)
{
String objectString = iter.next().toString();
lined.append(" ");
lined.append(objectString);
if (iter.hasNext())
{
lined.append("\n");
}
}
return lined.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy