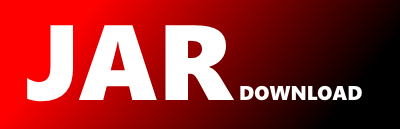
org.apache.wicket.util.value.IValueMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.ops4j.pax.wicket.service Show documentation
Show all versions of org.ops4j.pax.wicket.service Show documentation
Pax Wicket Service is an OSGi extension of the Wicket framework, allowing for dynamic loading and
unloading of Wicket components and pageSources.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.wicket.util.value;
import java.util.Map;
import org.apache.wicket.util.string.StringValue;
import org.apache.wicket.util.string.StringValueConversionException;
import org.apache.wicket.util.time.Duration;
import org.apache.wicket.util.time.Time;
/**
* A Map
interface that holds values, parses String
s, and exposes a
* variety of convenience methods.
*
* @author Johan Compagner
* @author Doug Donohoe
* @since 1.2.6
*/
public interface IValueMap extends Map
{
/**
* Retrieves a boolean
value by key.
*
* @param key
* the key
* @return the value
* @throws StringValueConversionException
*/
boolean getBoolean(final String key) throws StringValueConversionException;
/**
* Retrieves a double
value by key.
*
* @param key
* the key
* @return the value
* @throws StringValueConversionException
*/
double getDouble(final String key) throws StringValueConversionException;
/**
* Retrieves a double
value by key, using a default value if not found.
*
* @param key
* the key
* @param defaultValue
* value to use if no value is in this IValueMap
* @return the value
* @throws StringValueConversionException
*/
double getDouble(final String key, final double defaultValue)
throws StringValueConversionException;
/**
* Retrieves a Duration
by key.
*
* @param key
* the key
* @return the Duration
value
* @throws StringValueConversionException
*/
Duration getDuration(final String key) throws StringValueConversionException;
/**
* Retrieves an int
value by key.
*
* @param key
* the key
* @return the value
* @throws StringValueConversionException
*/
int getInt(final String key) throws StringValueConversionException;
/**
* Retrieves an int
value by key, using a default value if not found.
*
* @param key
* the key
* @param defaultValue
* value to use if no value is in this IValueMap
* @return the value
* @throws StringValueConversionException
*/
int getInt(final String key, final int defaultValue) throws StringValueConversionException;
/**
* Retrieves a long
value by key.
*
* @param key
* the key
* @return the value
* @throws StringValueConversionException
*/
long getLong(final String key) throws StringValueConversionException;
/**
* Retrieves a long
value by key, using a default value if not found.
*
* @param key
* the key
* @param defaultValue
* value to use if no value in this IValueMap
* @return the value
* @throws StringValueConversionException
*/
long getLong(final String key, final long defaultValue) throws StringValueConversionException;
/**
* Retrieves a String
by key, using a default value if not found.
*
* @param key
* the key
* @param defaultValue
* default value to return if value is null
* @return the String
*/
String getString(final String key, final String defaultValue);
/**
* Retrieves a String
by key.
*
* @param key
* the key
* @return the String
*/
String getString(final String key);
/**
* Retrieves a CharSequence
by key.
*
* @param key
* the key
* @return the CharSequence
*/
CharSequence getCharSequence(final String key);
/**
* Retrieves a String
array by key. If the value was a String[]
it
* will be returned directly. If it was a String
it will be converted to a
* String
array of length one. If it was an array of another type, a
* String
array will be made and each element will be converted to a
* String
.
*
* @param key
* the key
* @return the String
array of that key
*/
String[] getStringArray(final String key);
/**
* Retrieves a StringValue
object by key.
*
* @param key
* the key
* @return the StringValue
object
*/
StringValue getStringValue(final String key);
/**
* Retrieves a Time
object by key.
*
* @param key
* the key
* @return the Time
object
* @throws StringValueConversionException
*/
Time getTime(final String key) throws StringValueConversionException;
/**
* Returns whether or not this IValueMap
is immutable.
*
* @return whether or not this IValueMap
is immutable
*/
boolean isImmutable();
/**
* Makes this IValueMap
immutable by changing the underlying map representation to
* a Collections.unmodifiableMap
. After calling this method, any attempt to modify
* this IValueMap
will result in a RuntimeException
being thrown by
* the Collections
framework.
*
* @return this IValueMap
*/
IValueMap makeImmutable();
/**
* Provided that the hash key is a String
and you need to access the value ignoring
* the key's case (upper- or lowercase letters), then you may use this method to get the correct
* writing.
*
* @param key
* the key
* @return the key with the correct writing
*/
String getKey(final String key);
// //
// // getAs convenience methods
// //
/**
* Retrieves a Boolean
value by key.
*
* @param key
* the key
*
* @return the value or null if value is not a valid boolean or no value is in this
* IValueMap
*
*/
Boolean getAsBoolean(String key);
/**
* Retrieves a boolean
value by key.
*
* @param key
* the key
*
* @param defaultValue
* the default to return
*
* @return the value or defaultValue if value is not a valid boolean or no value is in this
* IValueMap
*
*/
boolean getAsBoolean(String key, boolean defaultValue);
/**
* Retrieves an Integer
value by key.
*
* @param key
* the key
*
* @return the value or null if value is not a valid integer or no value is in this
* IValueMap
*
*/
Integer getAsInteger(String key);
/**
* Retrieves an integer
value by key.
*
* @param key
* the key
*
* @param defaultValue
* the default to return
*
* @return the value or defaultValue if value is not a valid integer or no value is in this
* IValueMap
*
*/
int getAsInteger(String key, int defaultValue);
/**
* Retrieves a Long
value by key.
*
* @param key
* the key
*
* @return the value or null if value is not a valid long or no value is in this
* IValueMap
*
*/
Long getAsLong(String key);
/**
* Retrieves a long
value by key.
*
* @param key
* the key
*
* @param defaultValue
* the default to return
*
* @return the value or defaultValue if value is not a valid long or no value is in this
* IValueMap
*
*/
long getAsLong(String key, long defaultValue);
/**
* Retrieves a Double
value by key.
*
* @param key
* the key
*
* @return the value or null if value is not a valid double or no value is in this
* IValueMap
*
*/
Double getAsDouble(String key);
/**
* Retrieves a double
value by key.
*
* @param key
* the key
*
* @param defaultValue
* the default to return
*
* @return the value or defaultValue if value is not a valid double or no value is in this
* IValueMap
*
*/
double getAsDouble(String key, double defaultValue);
/**
* Retrieves a Duration
value by key.
*
* @param key
* the key
*
* @return the value or null if value is not a valid Duration or no value is in this
* IValueMap
*
*/
Duration getAsDuration(String key);
/**
* Retrieves a Duration
value by key.
*
* @param key
* the key
*
* @param defaultValue
* the default to return
*
* @return the value or defaultValue if value is not a valid Duration or no value is in this
* IValueMap
*
*/
Duration getAsDuration(String key, Duration defaultValue);
/**
* Retrieves a Time
value by key.
*
* @param key
* the key
*
* @return the value or null if value is not a valid Time or no value is in this
* IValueMap
*
*/
Time getAsTime(String key);
/**
* Retrieves a Time
value by key.
*
* @param key
* the key
*
* @param defaultValue
* the default to return
*
* @return the value or defaultValue if value is not a valid Time or no value is in this
* IValueMap
*
*/
Time getAsTime(String key, Time defaultValue);
/**
* Retrieves an Enum
value by key.
*
* @param
* type of enum
*
* @param key
* the key
*
* @param eClass
* the enumeration class
*
* @return the value or null if value is not a valid value of the Enumeration or no value is in
* this IValueMap
*
*/
> T getAsEnum(String key, Class eClass);
/**
* Retrieves an Enum
value by key.
*
* @param
* type of enum
*
* @param key
* the key
*
* @param defaultValue
* the default value from the Enumeration (cannot be null)
*
* @return the value or defaultValue if value is not a valid value of the Enumeration or no
* value is in this IValueMap
*
*/
> T getAsEnum(String key, T defaultValue);
/**
* Retrieves an Enum
value by key.
*
* @param
* type of enum
*
* @param key
* the key
*
* @param eClass
* the enumeration class
*
* @param defaultValue
* the default value from the Enumeration (may be null)
*
* @return the value or defaultValue if value is not a valid value of the Enumeration or no
* value is in this IValueMap
*
*/
> T getAsEnum(String key, Class eClass, T defaultValue);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy