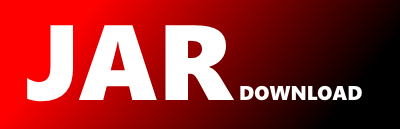
org.apache.wicket.util.value.LongValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.ops4j.pax.wicket.service Show documentation
Show all versions of org.ops4j.pax.wicket.service Show documentation
Pax Wicket Service is an OSGi extension of the Wicket framework, allowing for dynamic loading and
unloading of Wicket components and pageSources.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.wicket.util.value;
import java.io.Serializable;
import org.apache.wicket.util.lang.Primitives;
/**
* A base class based on the Java long
primitive for value classes that want to
* implement standard operations on that value without the pain of aggregating a Long
* object.
*
* @author Jonathan Locke
* @since 1.2.6
*/
public class LongValue implements Comparable, Serializable
{
private static final long serialVersionUID = 1L;
/** the long
value */
protected final long value;
/**
* Constructor.
*
* @param value
* the long
value
*/
public LongValue(final long value)
{
this.value = value;
}
/**
* Compares this Object
to a given Object
.
*
* @param that
* the Object
to compare with
* @return 0 if equal, -1 if less than the given Object
's value, or 1 if greater
* than given Object
's value
*/
public final int compareTo(final LongValue that)
{
if (value < that.value)
{
return -1;
}
if (value > that.value)
{
return 1;
}
return 0;
}
/**
* Tests for equality.
*
* @param that
* the Object
to compare with
* @return true
if this Object
's value is equal to the given
* Object
's value
*/
@Override
public final boolean equals(final Object that)
{
if (that instanceof LongValue)
{
return value == ((LongValue)that).value;
}
return false;
}
/**
* Compares this LongValue
with a primitive long
value.
*
* @param value
* the long
value to compare with
* @return true
if this LongValue
is greater than the given
* long
value
*/
public final boolean greaterThan(final long value)
{
return this.value > value;
}
/**
* Compares this LongValue
with another LongValue
.
*
* @param that
* the LongValue
to compare with
* @return true
if this LongValue
is greater than the given
* LongValue
*/
public final boolean greaterThan(final LongValue that)
{
return value > that.value;
}
/**
* Returns the hash code for this Object
.
*
* @return hash code for this Object
*/
@Override
public final int hashCode()
{
return Primitives.hashCode(value);
}
/**
* Compares this LongValue
with a primitive long
value.
*
* @param that
* the long
value to compare with
* @return true
if this LongValue
is less than the given
* long
value
*/
public final boolean lessThan(final long that)
{
return value < that;
}
/**
* Compares this LongValue
with another LongValue
.
*
* @param that
* the LongValue
value to compare with
* @return true
if this LongValue
is less than the given
* LongValue
*/
public final boolean lessThan(final LongValue that)
{
return value < that.value;
}
/**
* Converts this LongValue
to a String
.
*
* @return a String
representation of this LongValue
*/
@Override
public String toString()
{
return String.valueOf(value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy