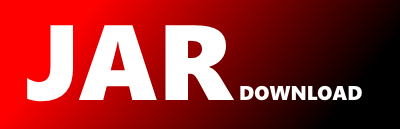
org.apache.wicket.util.template.PackageTextTemplate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.ops4j.pax.wicket.service Show documentation
Show all versions of org.ops4j.pax.wicket.service Show documentation
Pax Wicket Service is an OSGi extension of the Wicket framework, allowing for dynamic loading and
unloading of Wicket components and pageSources.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.wicket.util.template;
import java.io.IOException;
import java.util.Map;
import org.apache.wicket.Application;
import org.apache.wicket.util.io.Streams;
import org.apache.wicket.util.lang.Packages;
import org.apache.wicket.util.resource.IResourceStream;
import org.apache.wicket.util.resource.ResourceStreamNotFoundException;
import org.apache.wicket.util.resource.locator.ResourceStreamLocator;
import org.apache.wicket.util.string.interpolator.MapVariableInterpolator;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* A String
resource that can be appended to.
*
* @author Eelco Hillenius
* @since 1.2.6
*/
public class PackageTextTemplate extends TextTemplate
{
/** log. */
private static final Logger log = LoggerFactory.getLogger(PackageTextTemplate.class);
private static final long serialVersionUID = 1L;
/** The content type used if not provided in the constructor */
public static final String DEFAULT_CONTENT_TYPE = "text";
/** The encoding used if not provided in the constructor */
public static final String DEFAULT_ENCODING = null;
/** contents */
private final StringBuilder buffer = new StringBuilder();
/**
* Constructor.
*
* @param clazz
* the Class
to be used for retrieving the classloader for loading the
* PackagedTextTemplate
* @param fileName
* the name of the file, relative to the clazz
position
*/
public PackageTextTemplate(final Class> clazz, final String fileName)
{
this(clazz, fileName, DEFAULT_CONTENT_TYPE);
}
/**
* Constructor.
*
* @param clazz
* the Class
to be used for retrieving the classloader for loading the
* PackagedTextTemplate
* @param fileName
* the name of the file, relative to the clazz
position
* @param contentType
* the mime type of this resource, such as "image/jpeg
" or "
* text/html
"
*/
public PackageTextTemplate(final Class> clazz, final String fileName, final String contentType)
{
this(clazz, fileName, contentType, DEFAULT_ENCODING);
}
/**
* Constructor.
*
* @param clazz
* the Class
to be used for retrieving the classloader for loading the
* PackagedTextTemplate
* @param fileName
* the name of the file, relative to the clazz
position
* @param contentType
* the mime type of this resource, such as "image/jpeg
" or "
* text/html
"
* @param encoding
* the file's encoding, for example, "UTF-8
"
*/
public PackageTextTemplate(final Class> clazz, final String fileName,
final String contentType, final String encoding)
{
super(contentType);
String path = Packages.absolutePath(clazz, fileName);
Application app = Application.get();
// first try default class loading locator to find the resource
IResourceStream stream = app.getResourceSettings()
.getResourceStreamLocator()
.locate(clazz, path);
if (stream == null)
{
// if the default locator didn't find the resource then fallback
stream = new ResourceStreamLocator().locate(clazz, path);
}
if (stream == null)
{
throw new IllegalArgumentException("resource " + fileName + " not found for scope " +
clazz + " (path = " + path + ")");
}
setLastModified(stream.lastModifiedTime());
try
{
if (encoding != null)
{
buffer.append(Streams.readString(stream.getInputStream(), encoding));
}
else
{
buffer.append(Streams.readString(stream.getInputStream()));
}
}
catch (IOException e)
{
throw new RuntimeException(e);
}
catch (ResourceStreamNotFoundException e)
{
throw new RuntimeException(e);
}
finally
{
try
{
stream.close();
}
catch (IOException e)
{
log.error(e.getMessage(), e);
}
}
}
/**
* @see org.apache.wicket.util.resource.AbstractStringResourceStream#getString()
*/
@Override
public String getString()
{
return buffer.toString();
}
/**
* Interpolates a Map
of variables with the content and replaces the content with
* the result. Variables are denoted in the String
by the
* syntax ${variableName}
. The contents will be altered by replacing each variable
* of the form ${variableName}
with the value returned by
* variables.getValue("variableName")
.
*
* WARNING: there is no going back to the original contents after the interpolation is done. If
* you need to do different interpolations on the same original contents, use the method
* {@link #asString(Map)} instead.
*
*
* @param variables
* a Map
of variables to interpolate
* @return this for chaining
*/
@Override
public final TextTemplate interpolate(Map variables)
{
if (variables != null)
{
String result = new MapVariableInterpolator(buffer.toString(), variables).toString();
buffer.delete(0, buffer.length());
buffer.append(result);
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy