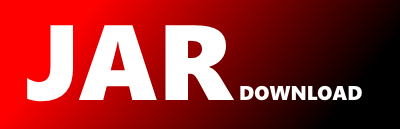
org.optaplanner.constraint.streams.bavet.bi.JoinBiNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of optaplanner-constraint-streams-bavet Show documentation
Show all versions of optaplanner-constraint-streams-bavet Show documentation
OptaPlanner solves planning problems.
This lightweight, embeddable planning engine implements powerful and scalable algorithms
to optimize business resource scheduling and planning.
This module contains implementation of Constraint streams (Bavet).
package org.optaplanner.constraint.streams.bavet.bi;
import java.util.Map;
import java.util.function.Function;
import org.optaplanner.constraint.streams.bavet.common.AbstractJoinNode;
import org.optaplanner.constraint.streams.bavet.common.TupleLifecycle;
import org.optaplanner.constraint.streams.bavet.common.index.IndexProperties;
import org.optaplanner.constraint.streams.bavet.common.index.Indexer;
import org.optaplanner.constraint.streams.bavet.uni.UniTuple;
final class JoinBiNode extends AbstractJoinNode, B, BiTuple, BiTupleImpl> {
private final Function mappingA;
private final int outputStoreSize;
public JoinBiNode(Function mappingA, Function mappingB,
int inputStoreIndexA, int inputStoreIndexB,
TupleLifecycle> nextNodesTupleLifecycle,
int outputStoreSize,
Indexer, Map, BiTupleImpl>> indexerA,
Indexer, Map, BiTupleImpl>> indexerB) {
super(mappingB, inputStoreIndexA, inputStoreIndexB, nextNodesTupleLifecycle, indexerA, indexerB);
this.mappingA = mappingA;
this.outputStoreSize = outputStoreSize;
}
@Override
protected IndexProperties createIndexPropertiesLeft(UniTuple leftTuple) {
return mappingA.apply(leftTuple.getFactA());
}
@Override
protected BiTupleImpl createOutTuple(UniTuple leftTuple, UniTuple rightTuple) {
return new BiTupleImpl<>(leftTuple.getFactA(), rightTuple.getFactA(), outputStoreSize);
}
@Override
protected void updateOutTupleLeft(BiTupleImpl outTuple, UniTuple leftTuple) {
outTuple.factA = leftTuple.getFactA();
}
@Override
protected void updateOutTupleRight(BiTupleImpl outTuple, UniTuple rightTuple) {
outTuple.factB = rightTuple.getFactA();
}
@Override
public String toString() {
return "JoinBiNode";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy