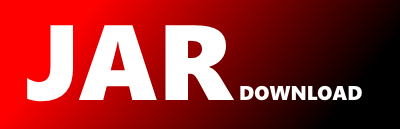
org.optaplanner.constraint.streams.common.tri.TriJoinerComber Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of optaplanner-constraint-streams-common Show documentation
Show all versions of optaplanner-constraint-streams-common Show documentation
OptaPlanner solves planning problems.
This lightweight, embeddable planning engine implements powerful and scalable algorithms
to optimize business resource scheduling and planning.
This module contains classes common to each implementation of Constraint streams.
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.optaplanner.constraint.streams.common.tri;
import java.util.ArrayList;
import java.util.List;
import org.optaplanner.core.api.function.TriPredicate;
import org.optaplanner.core.api.score.stream.tri.TriJoiner;
/**
* Combs an array of {@link TriJoiner} instances into a mergedJoiner and a mergedFiltering.
*
* @param
* @param
* @param
*/
public final class TriJoinerComber {
public static TriJoinerComber comb(TriJoiner[] joiners) {
List> defaultJoinerList = new ArrayList<>(joiners.length);
List> filteringList = new ArrayList<>(joiners.length);
int indexOfFirstFilter = -1;
// Make sure all indexing joiners, if any, come before filtering joiners. This is necessary for performance.
for (int i = 0; i < joiners.length; i++) {
TriJoiner joiner = joiners[i];
if (joiner instanceof FilteringTriJoiner) {
// From now on, only allow filtering joiners.
indexOfFirstFilter = i;
filteringList.add(((FilteringTriJoiner) joiner).getFilter());
} else if (joiner instanceof DefaultTriJoiner) {
if (indexOfFirstFilter >= 0) {
throw new IllegalStateException("Indexing joiner (" + joiner + ") must not follow " +
"a filtering joiner (" + joiners[indexOfFirstFilter] + ").\n" +
"Maybe reorder the joiners such that filtering() joiners are later in the parameter list.");
}
defaultJoinerList.add((DefaultTriJoiner) joiner);
} else {
throw new IllegalArgumentException("The joiner class (" + joiner.getClass() + ") is not supported.");
}
}
DefaultTriJoiner mergedJoiner = DefaultTriJoiner.merge(defaultJoinerList);
TriPredicate mergedFiltering = mergeFiltering(filteringList);
return new TriJoinerComber<>(mergedJoiner, mergedFiltering);
}
private static TriPredicate mergeFiltering(List> filteringList) {
if (filteringList.isEmpty()) {
return null;
}
switch (filteringList.size()) {
case 1:
return filteringList.get(0);
case 2:
return filteringList.get(0).and(filteringList.get(1));
default:
// Avoid predicate.and() when more than 2 predicates for debugging and potentially performance
return (A a, B b, C c) -> {
for (TriPredicate predicate : filteringList) {
if (!predicate.test(a, b, c)) {
return false;
}
}
return true;
};
}
}
private final DefaultTriJoiner mergedJoiner;
private final TriPredicate mergedFiltering;
public TriJoinerComber(DefaultTriJoiner mergedJoiner, TriPredicate mergedFiltering) {
this.mergedJoiner = mergedJoiner;
this.mergedFiltering = mergedFiltering;
}
/**
* @return never null
*/
public DefaultTriJoiner getMergedJoiner() {
return mergedJoiner;
}
/**
* @return null if not applicable
*/
public TriPredicate getMergedFiltering() {
return mergedFiltering;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy