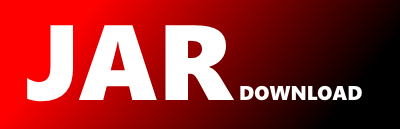
org.h2gis.drivers.kml.KMLGeometry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of h2drivers Show documentation
Show all versions of h2drivers Show documentation
Add H2 read/write support for file formats such as ESRI shape file
The newest version!
/**
* H2GIS is a library that brings spatial support to the H2 Database Engine
* .
*
* H2GIS is distributed under GPL 3 license. It is produced by CNRS
* .
*
* H2GIS is free software: you can redistribute it and/or modify it under the
* terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* H2GIS is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR
* A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with
* H2GIS. If not, see .
*
* For more information, please consult:
* or contact directly: info_at_h2gis.org
*/
package org.h2gis.drivers.kml;
import com.vividsolutions.jts.geom.Coordinate;
import com.vividsolutions.jts.geom.Geometry;
import com.vividsolutions.jts.geom.GeometryCollection;
import com.vividsolutions.jts.geom.LineString;
import com.vividsolutions.jts.geom.Point;
import com.vividsolutions.jts.geom.Polygon;
import java.sql.SQLException;
/**
* Tools to convert JTS geometry to KML representation
*
* @author Erwan Bocher
*/
public class KMLGeometry {
private KMLGeometry() {
}
/**
* Convert JTS geometry to a kml geometry representation.
*
* @param geom
* @param sb
* @throws SQLException
*/
public static void toKMLGeometry(Geometry geom, StringBuilder sb) throws SQLException {
toKMLGeometry(geom, ExtrudeMode.NONE, AltitudeMode.NONE, sb);
}
/**
* Convert JTS geometry to a kml geometry representation.
*
* @param geometry
* @param extrude
* @param altitudeModeEnum
* @param sb
*/
public static void toKMLGeometry(Geometry geometry, ExtrudeMode extrude, int altitudeModeEnum, StringBuilder sb) throws SQLException {
if (geometry instanceof Point) {
toKMLPoint((Point) geometry, extrude, altitudeModeEnum, sb);
} else if (geometry instanceof LineString) {
toKMLLineString((LineString) geometry, extrude, altitudeModeEnum, sb);
} else if (geometry instanceof Polygon) {
toKMLPolygon((Polygon) geometry, extrude, altitudeModeEnum, sb);
} else if (geometry instanceof GeometryCollection) {
toKMLMultiGeometry((GeometryCollection) geometry, extrude, altitudeModeEnum, sb);
} else {
throw new SQLException("This geometry type is not supported : " + geometry.toString());
}
}
/**
* A geographic location defined by longitude, latitude, and (optional)
* altitude.
*
* Syntax :
*
*
*
* 0
* clampToGround
*
*
* ...
*
*
* Supported syntax :
*
* 0
* clampToGround
* ...
*
*
* @param point
* @param extrude
* @param altitudeModeEnum
*/
public static void toKMLPoint(Point point, ExtrudeMode extrude, int altitudeModeEnum, StringBuilder sb) {
sb.append("");
appendExtrude(extrude, sb);
appendAltitudeMode(altitudeModeEnum, sb);
sb.append("");
Coordinate coord = point.getCoordinate();
sb.append(coord.x).append(",").append(coord.y);
if (!Double.isNaN(coord.z)) {
sb.append(",").append(coord.z);
}
sb.append(" ").append(" ");
}
/**
* Defines a connected set of line segments.
*
* Syntax :
*
*
*
* 0
* 0
* 0
* clampToGround
*
*
* 0
* ...
*
*
* Supported syntax :
*
*
* 0
* clampToGround
* ...
*
*
* @param lineString
*/
public static void toKMLLineString(LineString lineString, ExtrudeMode extrude, int altitudeModeEnum, StringBuilder sb) {
sb.append("");
appendExtrude(extrude, sb);
appendAltitudeMode(altitudeModeEnum, sb);
appendKMLCoordinates(lineString.getCoordinates(), sb);
sb.append(" ");
}
/**
* Defines a closed line string, typically the outer boundary of a Polygon.
*
* Syntax :
*
*
*
* 0
* 0
* 0
* clampToGround
*
*
* ...
*
*
* Supported syntax :
*
*
* 0
* clampToGround
* ...
*
*
* @param lineString
*/
public static void toKMLLinearRing(LineString lineString, ExtrudeMode extrude, int altitudeModeEnum, StringBuilder sb) {
sb.append("");
appendExtrude(extrude, sb);
appendAltitudeMode(altitudeModeEnum, sb);
appendKMLCoordinates(lineString.getCoordinates(), sb);
sb.append(" ");
}
/**
* A Polygon is defined by an outer boundary and 0 or more inner boundaries.
* The boundaries, in turn, are defined by LinearRings.
*
* Syntax :
*
*
*
* 0
* 0
* clampToGround
*
*
*
*
* ...
*
*
*
*
* ...
*
*
*
*
* Supported syntax :
*
*
* 0
* clampToGround
*
*
* ...
*
*
*
*
* ...
*
*
*
*
* @param polygon
*/
public static void toKMLPolygon(Polygon polygon, ExtrudeMode extrude, int altitudeModeEnum, StringBuilder sb) {
sb.append("");
appendExtrude(extrude, sb);
appendAltitudeMode(altitudeModeEnum, sb);
sb.append("");
toKMLLinearRing(polygon.getExteriorRing(), extrude, altitudeModeEnum, sb);
sb.append(" ");
for (int i = 0; i < polygon.getNumInteriorRing(); i++) {
sb.append("");
toKMLLinearRing(polygon.getInteriorRingN(i), extrude, altitudeModeEnum, sb);
sb.append(" ");
}
sb.append(" ");
}
/**
*
*
* A container for zero or more geometry primitives associated with the same
* feature.
*
*
*
*
*
*
* @param gc
*/
public static void toKMLMultiGeometry(GeometryCollection gc, ExtrudeMode extrude, int altitudeModeEnum, StringBuilder sb) {
sb.append("");
for (int i = 0; i < gc.getNumGeometries(); i++) {
Geometry geom = gc.getGeometryN(i);
if (geom instanceof Point) {
toKMLPoint((Point) geom, extrude, altitudeModeEnum, sb);
} else if (geom instanceof LineString) {
toKMLLineString((LineString) geom, extrude, altitudeModeEnum, sb);
} else if (geom instanceof Polygon) {
toKMLPolygon((Polygon) geom, extrude, altitudeModeEnum, sb);
}
}
sb.append(" ");
}
/**
* Build a string represention to kml coordinates
*
* Syntax :
*
* ...
*
* @param coords
*/
public static void appendKMLCoordinates(Coordinate[] coords, StringBuilder sb) {
sb.append("");
for (int i = 0; i < coords.length; i++) {
Coordinate coord = coords[i];
sb.append(coord.x).append(",").append(coord.y);
if (!Double.isNaN(coord.z)) {
sb.append(",").append(coord.z);
}
if (i < coords.length - 1) {
sb.append(" ");
}
}
sb.append(" ");
}
/**
* Append the extrude value
*
* Syntax :
*
* 0
*
* @param extrude
* @param sb
*/
private static void appendExtrude(ExtrudeMode extrude, StringBuilder sb) {
if (extrude.equals(ExtrudeMode.TRUE)) {
sb.append("").append(1).append(" ");
} else if (extrude.equals(ExtrudeMode.FALSE)) {
sb.append("").append(0).append(" ");
}
}
/**
* Append the altitudeMode
*
* Syntax :
*
* clampToGround
*
* @param altitudeModeEnum
* @param sb
*/
private static void appendAltitudeMode(int altitudeModeEnum, StringBuilder sb) {
AltitudeMode.append(altitudeModeEnum, sb);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy