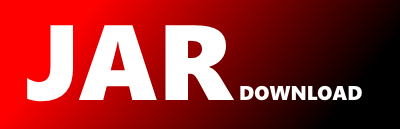
org.organicdesign.fp.function.Function1 Maven / Gradle / Ivy
// Copyright 2013-12-30 PlanBase Inc. & Glen Peterson
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package org.organicdesign.fp.function;
import org.organicdesign.fp.Option;
import org.organicdesign.fp.collections.UnmodIterable;
import org.organicdesign.fp.xform.Transformable;
import org.organicdesign.fp.xform.Xform;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.function.Consumer;
import java.util.function.Function;
/**
This is like Java 8's java.util.function.Function, but retrofitted to turn checked exceptions
into unchecked ones.
*/
@FunctionalInterface
public interface Function1 extends Function, Consumer {
/** Implement this one method and you don't have to worry about checked exceptions. */
U applyEx(T t) throws Exception;
/** Call this convenience method so that you don't have to worry about checked exceptions. */
@Override default U apply(T t) {
try {
return applyEx(t);
} catch (RuntimeException re) {
throw re;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
@Override default void accept(T t) { apply(t); }
@SuppressWarnings("unchecked")
default Function1 compose(final Function1 super S, ? extends T> f) {
if (f == IDENTITY) {
// This violates type safety, but makes sense - composing any function with the
// identity function should return the original function unchanged. If you mess up the
// types, then that's your problem. With generics and type erasure this may be the
// best you can do.
return (Function1) this;
}
final Function1 parent = this;
return new Function1() {
@Override
public U applyEx(S s) throws Exception {
return parent.applyEx(f.applyEx(s));
}
};
}
public static final Function1
© 2015 - 2025 Weber Informatics LLC | Privacy Policy