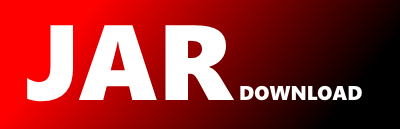
org.osgl.xls.SheetSelector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of excel-reader Show documentation
Show all versions of excel-reader Show documentation
An easy to use and flexible Excel file reader
package org.osgl.xls;
/*-
* #%L
* Java Excel Reader
* %%
* Copyright (C) 2017 OSGL (Open Source General Library)
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import org.apache.poi.ss.usermodel.Sheet;
import org.osgl.$;
import org.osgl.util.E;
import org.osgl.util.S;
import java.util.Arrays;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
/**
* Utility to define sheet select logic
*/
public class SheetSelector {
/**
* Select all sheets
*/
public static $.Predicate ALL = new $.Predicate() {
@Override
public boolean test(Sheet rows) {
return true;
}
};
/**
* Select sheets by name list. Name list is case insensitive
*
* The name list could be provided using string array or a single string
* with name list separated by comma `,`
*
* @param names the name list
* @return a predicate to select sheets
*/
public static $.Predicate byName(final String ... names) {
E.illegalArgumentIf(names.length == 0, "name list expected");
final Set nameList = new HashSet<>();
for (String s : names) {
if (S.blank(s)) {
continue;
}
s = s.trim().toLowerCase();
List splited = S.fastSplit(s, ",");
nameList.addAll(splited);
}
return new $.Predicate() {
@Override
public boolean test(Sheet sheet) {
return nameList.contains(sheet.getSheetName().toLowerCase());
}
};
}
/**
* Select sheets by exclude name list. Name list is case insensitive
*
* The name list could be provided using string array or a single string
* with name list separated by comma `,`
*
* @param names the exclude name list
* @return a predicate to select sheets
*/
public static $.Predicate excludeByName(final String... names) {
return byName(names).negate();
}
/**
* Select sheets by index list. Note index number is `0` based
*
* @param indexes the index list
* @return a predicate to select sheets
*/
public static $.Predicate byPosition(final int ... indexes) {
E.illegalArgumentIf(indexes.length == 0, "index list expected");
Arrays.sort(indexes);
return new $.Predicate() {
@Override
public boolean test(Sheet sheet) {
int myIndex = sheet.getWorkbook().getSheetIndex(sheet);
return Arrays.binarySearch(indexes, myIndex) > - 1;
}
};
}
/**
* Select sheets by exclude index list. Note index number is `0` based
*
* @param indexes the exclude index list
* @return a predicate to select sheets
*/
public static $.Predicate excludeByPosition(final int... indexes) {
return byPosition(indexes).negate();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy