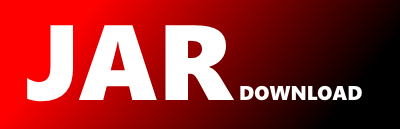
org.osgl.util.DelegatingList Maven / Gradle / Ivy
The newest version!
package org.osgl.util;
/*-
* #%L
* Java Tool
* %%
* Copyright (C) 2014 - 2017 OSGL (Open Source General Library)
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import java.io.Serializable;
import java.util.*;
/**
* Implement {@link C.List} with a backing {@link java.util.List} instance
*/
class DelegatingList extends ListBase implements C.List, Serializable {
private static EnumSet freeFeatures = EnumSet.of(C.Feature.LAZY, C.Feature.PARALLEL);
private static EnumSet setableFeatures = EnumSet.of(C.Feature.READONLY); static {
setableFeatures.addAll(freeFeatures);
}
protected java.util.List data;
protected DelegatingList(boolean noInit) {
if (noInit) {
return;
}
data = C.randomAccessListFact.create(10);
}
DelegatingList() {
this(10, C.randomAccessListFact);
}
DelegatingList(int initialCapacity) {
this(initialCapacity, C.randomAccessListFact);
}
DelegatingList(C.ListFactory fact) {
this(10, fact);
}
DelegatingList(int initialCapacity, C.ListFactory fact) {
data = fact.create(initialCapacity);
}
DelegatingList(Iterable extends T> iterable) {
this(iterable, C.randomAccessListFact);
}
DelegatingList(Iterable extends T> iterable, C.ListFactory fact) {
E.NPE(iterable);
if (iterable instanceof Collection) {
data = fact.create((Collection extends T>)iterable);
} else {
data = fact.create();
for (T t : iterable) {
data.add(t);
}
}
}
private DelegatingList(java.util.List list, boolean wrapDirectly) {
E.NPE(list);
if (wrapDirectly) {
data = list;
} else {
data = C.randomAccessListFact.create(list);
}
}
DelegatingList(Collection col, C.ListFactory fact) {
data = fact.create(col);
}
// -----------------------------------------------------------------------------------------
@Override
protected EnumSet initFeatures() {
EnumSet fs = (data instanceof C.List) ?
((C.List)data).features()
: EnumSet.of(C.Feature.LIMITED, C.Feature.ORDERED);
if (data instanceof RandomAccess) {
fs.add(C.Feature.RANDOM_ACCESS);
}
return fs;
}
@Override
public ListIterator listIterator(int index) {
return data.listIterator(index);
}
@Override
public int size() {
return data.size();
}
@Override
public T get(int index) {
return data.get(index);
}
@Override
public T set(int index, T element) {
if (isMutable()) {
return data.set(index, element);
}
throw new UnsupportedOperationException();
}
@Override
public void add(int index, T element) {
if (isMutable()) {
data.add(index, element);
return;
}
throw new UnsupportedOperationException();
}
@Override
public T remove(int index) {
if (isMutable()) {
return data.remove(index);
}
throw new UnsupportedOperationException();
}
static C.List wrap(java.util.List list) {
if (list instanceof C.List) {
C.List cl = (C.List)list;
if (cl.is(C.Feature.IMMUTABLE) && cl.isEmpty()) {
return Nil.list();
}
}
if (list instanceof DelegatingList) {
list = ((DelegatingList)list).data;
}
return new DelegatingList(list, true);
}
static C.List wrap(java.util.List list, C.Feature f1) {
C.List l = wrap(list);
if (l instanceof DelegatingList) {
DelegatingList dl = (DelegatingList)l;
if (setableFeatures.contains(f1)) {
dl.features_().add(f1);
}
}
return l;
}
static C.List wrap(java.util.List list, C.Feature f1, C.Feature f2) {
C.List l = wrap(list);
if (l instanceof DelegatingList) {
DelegatingList dl = (DelegatingList)l;
if (setableFeatures.contains(f1)) {
dl.features_().add(f1);
}
if (setableFeatures.contains(f2)) {
dl.features_().add(f2);
}
}
return l;
}
static C.List wrap(java.util.List list, C.Feature f1, C.Feature f2, C.Feature f3) {
C.List l = wrap(list);
if (l instanceof DelegatingList) {
DelegatingList dl = (DelegatingList)l;
if (setableFeatures.contains(f1)) {
dl.features_().add(f1);
}
if (setableFeatures.contains(f2)) {
dl.features_().add(f2);
}
if (setableFeatures.contains(f3)) {
dl.features_().add(f3);
}
}
return l;
}
static DelegatingList copyOf(Iterable iterable) {
return new DelegatingList(iterable);
}
static DelegatingList copyOf(Iterable iterable, C.Feature f1) {
DelegatingList l = copyOf(iterable);
if (setableFeatures.contains(f1)) {
l.features_().add(f1);
}
return l;
}
static DelegatingList copyOf(Iterable iterable, C.Feature f1, C.Feature f2) {
DelegatingList l = copyOf(iterable);
if (setableFeatures.contains(f1)) {
l.features_().add(f1);
}
if (setableFeatures.contains(f2)) {
l.features_().add(f2);
}
return l;
}
static DelegatingList copyOf(Iterable iterable, C.Feature f1, C.Feature f2, C.Feature f3) {
DelegatingList l = copyOf(iterable);
if (setableFeatures.contains(f1)) {
l.features_().add(f1);
}
if (setableFeatures.contains(f2)) {
l.features_().add(f2);
}
if (setableFeatures.contains(f3)) {
l.features_().add(f3);
}
return l;
}
private void writeObject(java.io.ObjectOutputStream s) throws java.io.IOException{
s.defaultWriteObject();
s.writeObject(data);
s.writeObject(features_());
}
private void readObject(java.io.ObjectInputStream s)
throws java.io.IOException, ClassNotFoundException {
s.defaultReadObject();
data = (List)s.readObject();
EnumSet features = (EnumSet)s.readObject();
features_().addAll(features);
}
}
class DelegatingStringList extends DelegatingList implements S.List {
public DelegatingStringList(boolean noInit) {
super(noInit);
}
public DelegatingStringList() {
}
public DelegatingStringList(int initialCapacity) {
super(initialCapacity);
}
public DelegatingStringList(C.ListFactory fact) {
super(fact);
}
public DelegatingStringList(int initialCapacity, C.ListFactory fact) {
super(initialCapacity, fact);
}
public DelegatingStringList(Iterable extends String> iterable) {
super(iterable);
}
public DelegatingStringList(Iterable extends String> iterable, C.ListFactory fact) {
super(iterable, fact);
}
public DelegatingStringList(Collection col, C.ListFactory fact) {
super(col, fact);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy