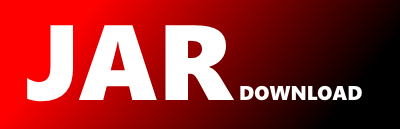
org.osgl.util.Nil Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2013 The Java Tool project
* Gelin Luo
*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.osgl.util;
/*-
* #%L
* Java Tool
* %%
* Copyright (C) 2014 - 2017 OSGL (Open Source General Library)
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import org.osgl.$;
import org.osgl.Lang;
import org.osgl.exception.NotAppliedException;
import java.io.Serializable;
import java.util.*;
/**
* Implementing immutable empty collection types
*/
abstract class Nil extends SequenceBase implements C.Traversable, Collection, Serializable {
public static final EmptySequence SEQUENCE = EmptySequence.INSTANCE;
public static final EmptyReversibleSequence REVERSIBLE_SEQUENCE = EmptyReversibleSequence.INSTANCE;
public static final EmptyRange RANGE = EmptyRange.INSTANCE;
public static final EmptyList LIST = EmptyList.INSTANCE;
public static final Empty EMPTY = Empty.INSTANCE;
private static final long serialVersionUID = -5058901899659394002L;
public static EmptySet SET = EmptySet.INSTANCE;
public static final C.Map EMPTY_MAP = new C.Map(true);
// public static final EmptySet SET = EmptySet.INSTANCE;
//
// @SuppressWarnings("unchecked")
// public static EmptySet set() {
// return (EmptySet) EmptySet.INSTANCE;
// }
//
// public static final EmptySortedSet SORTED_SET = EmptySortedSet.INSTANCE;
//
// @SuppressWarnings("unchecked")
// public static EmptySortedSet sortedSet() {
// return (EmptySortedSet) EmptySortedSet.INSTANCE;
// }
//
private Nil() {
}
@Override
public C.ListOrSet collect(String path) {
return EMPTY;
}
@Override
public C.List asList() {
return list();
}
public static Map emptyMap() {
return $.cast(EMPTY_MAP);
}
@SuppressWarnings("unchecked")
public static EmptySequence seq() {
return (EmptySequence) SEQUENCE;
}
@SuppressWarnings("unchecked")
public static EmptyReversibleSequence rseq() {
return (EmptyReversibleSequence) REVERSIBLE_SEQUENCE;
}
@SuppressWarnings("unchecked")
public static EmptyRange range() {
return (EmptyRange) RANGE;
}
@SuppressWarnings("unchecked")
public static EmptyList list() {
return (EmptyList) EmptyList.INSTANCE;
}
@SuppressWarnings("unchecked")
public static EmptySet set() {
return (EmptySet) EmptySet.INSTANCE;
}
@SuppressWarnings("unchecked")
public static Empty empty() {
return (Empty) Empty.INSTANCE;
}
@Override
protected EnumSet initFeatures() {
return EnumSet.of(C.Feature.IMMUTABLE, C.Feature.ORDERED, C.Feature.LIMITED, C.Feature.RANDOM_ACCESS, C.Feature.READONLY, C.Feature.SORTED, C.Feature.PARALLEL);
}
protected final java.util.List emptyJavaList() {
return Collections.emptyList();
}
protected abstract Nil singleton();
@Override
protected final Object clone() throws CloneNotSupportedException {
throw new CloneNotSupportedException();
}
public final Iterator iterator() {
return emptyJavaList().iterator();
}
@Override
public final int size() {
return 0;
}
@Override
public final boolean contains(Object o) {
return false;
}
@Override
public final Object[] toArray() {
return new Object[0];
}
@Override
public final T1[] toArray(T1[] a) {
return emptyJavaList().toArray(a);
}
@Override
public final boolean add(T t) {
throw new UnsupportedOperationException();
}
@Override
public final boolean remove(Object o) {
throw new UnsupportedOperationException();
}
@Override
public final boolean containsAll(Collection> c) {
return c.isEmpty();
}
@Override
public final boolean addAll(Collection extends T> c) {
if (c.isEmpty()) {
return false;
}
throw new UnsupportedOperationException();
}
@Override
public final boolean removeAll(Collection> c) {
return false;
}
@Override
public final boolean retainAll(Collection> c) {
return false;
}
@Override
public final void clear() {
}
@Override
public final boolean isEmpty() {
return true;
}
@Override
public C.Sequence map($.Function super T, ? extends R> mapper) {
return singleton();
}
@Override
public C.Sequence flatMap($.Function super T, ? extends Iterable extends R>> mapper) {
return singleton();
}
@Override
public Nil filter($.Function super T, Boolean> predicate) {
return this;
}
@Override
public final R reduce(R identity, $.Func2 accumulator) {
return identity;
}
@Override
public final $.Option reduce($.Func2 accumulator) {
return $.none();
}
@Override
public final boolean allMatch($.Function super T, Boolean> predicate) {
return false;
}
@Override
public final boolean anyMatch($.Function super T, Boolean> predicate) {
return false;
}
@Override
public final boolean noneMatch($.Function super T, Boolean> predicate) {
return true;
}
@Override
public final $.Option findOne($.Function super T, Boolean> predicate) {
return $.none();
}
@Override
public Nil accept($.Visitor super T> visitor) {
return this;
}
@Override
public int hashCode() {
return 1;
}
@Override
public boolean equals(Object obj) {
return obj == this;
}
static class EmptySequence extends Nil implements C.Sequence {
private static final EmptySequence INSTANCE = new EmptySequence();
private EmptySequence() {
}
protected EmptySequence singleton() {
return INSTANCE;
}
/**
* {@inheritDoc}
* Returns an immutable singleton list contains the element
* specified
*
* @param t the element to be appended to this sequence
* @return a singleton list of the element
*/
@Override
public C.Sequence append(T t) {
return C.list(t);
}
/**
* {@inheritDoc}
* Returns an immutable singleton list contains the
* element specified
*
* @param t the element to be prepended to this sequence
* @return a singleton list of the element
*/
@Override
public C.Sequence prepend(T t) {
return C.list(t);
}
/**
* {@inheritDoc}
* Returns identity specified
*
* @param identity {@inheritDoc}
* @param accumulator {@inheritDoc}
* @param the type of identity and result
* @return {@inheritDoc}
*/
@Override
public R reduceLeft(R identity, $.Func2 accumulator) {
return identity;
}
@Override
public $.Option reduceLeft($.Func2 accumulator) {
return $.none();
}
@Override
public $.Option findFirst($.Function super T, Boolean> predicate) {
return $.none();
}
@Override
public EmptySequence acceptLeft($.Visitor super T> visitor) {
return this;
}
@Override
public EmptySequence accept($.Visitor super T> visitor) {
return this;
}
@Override
public T first() {
throw new NoSuchElementException();
}
@Override
public EmptySequence head(int n) {
return this;
}
@Override
public EmptySequence tail() throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
@Override
public EmptySequence take(int n) {
return this;
}
@Override
public EmptySequence takeWhile($.Function super T, Boolean> predicate) {
return this;
}
@Override
public EmptySequence drop(int n) {
return this;
}
@Override
public EmptySequence dropWhile($.Function super T, Boolean> predicate) {
return this;
}
@Override
public EmptySequence map($.Function super T, ? extends R> mapper) {
return singleton();
}
@Override
public EmptySequence flatMap($.Function super T, ? extends Iterable extends R>> mapper) {
return singleton();
}
@Override
public EmptySequence filter($.Function super T, Boolean> predicate) {
return this;
}
@Override
public C.Sequence append(C.Sequence extends T> seq) {
return Lang.cast(seq);
}
@Override
public C.Sequence prepend(C.Sequence extends T> seq) {
return Lang.cast(seq);
}
@Override
public C.Sequence prepend(Iterable extends T> iterable) {
if (!iterable.iterator().hasNext()) {
return this;
}
return C.seq(iterable);
}
@Override
public C.Sequence prepend(Iterator extends T> iterator) {
if (!iterator.hasNext()) {
return this;
}
return C.seq(iterator);
}
@Override
public C.Sequence prepend(Enumeration extends T> enumeration) {
if (!enumeration.hasMoreElements()) {
return this;
}
return C.seq(enumeration);
}
@Override
public C.Sequence append(Iterator extends T> iterator) {
return C.seq(iterator);
}
@Override
public C.Sequence append(Enumeration extends T> enumeration) {
return C.seq(enumeration);
}
// Preserves singleton property
private Object readResolve() {
return INSTANCE;
}
}
static class EmptyReversibleSequence
extends EmptySequence implements C.ReversibleSequence {
private static final EmptyReversibleSequence INSTANCE = new EmptyReversibleSequence();
@Override
protected EmptyReversibleSequence singleton() {
return INSTANCE;
}
@Override
public C.ReversibleSequence lazy() {
return (C.ReversibleSequence) super.lazy();
}
@Override
public C.ReversibleSequence eager() {
return (C.ReversibleSequence) super.eager();
}
@Override
public C.ReversibleSequence parallel() {
return (C.ReversibleSequence) super.parallel();
}
@Override
public C.ReversibleSequence sequential() {
return (C.ReversibleSequence) super.sequential();
}
@Override
public T last() throws UnsupportedOperationException, NoSuchElementException {
throw new NoSuchElementException();
}
@Override
public EmptyReversibleSequence reverse() throws UnsupportedOperationException {
return this;
}
@Override
public EmptyReversibleSequence take(int n) {
return this;
}
@Override
public EmptyReversibleSequence head(int n) {
return this;
}
@Override
public EmptyReversibleSequence tail() throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
@Override
public EmptyReversibleSequence tail(int n) throws UnsupportedOperationException {
return this;
}
@Override
public EmptyReversibleSequence drop(int n) {
return this;
}
@Override
public Iterator reverseIterator() {
return iterator();
}
@Override
public EmptyReversibleSequence accept($.Visitor super T> visitor) {
return this;
}
@Override
public EmptyReversibleSequence each($.Visitor super T> visitor) {
return this;
}
@Override
public EmptyReversibleSequence forEach($.Visitor super T> visitor) {
return this;
}
@Override
public EmptyReversibleSequence takeWhile($.Function super T, Boolean> predicate) {
return this;
}
@Override
public EmptyReversibleSequence dropWhile($.Function super T, Boolean> predicate) {
return this;
}
@Override
public EmptyReversibleSequence map($.Function super T, ? extends R> mapper) {
return singleton();
}
@Override
public EmptyReversibleSequence flatMap($.Function super T, ? extends Iterable extends R>> mapper) {
return singleton();
}
@Override
public EmptyReversibleSequence filter($.Function super T, Boolean> predicate) {
return this;
}
@Override
public EmptyReversibleSequence acceptLeft($.Visitor super T> visitor) {
return this;
}
@Override
public R reduceRight(R identity, $.Func2 accumulator) {
return identity;
}
@Override
public $.Option reduceRight($.Func2 accumulator) {
return $.none();
}
@Override
public $.Option findLast($.Function super T, Boolean> predicate) {
return $.none();
}
@Override
public EmptyReversibleSequence acceptRight($.Visitor super T> visitor) {
return this;
}
@Override
public C.ReversibleSequence append(C.ReversibleSequence seq) {
return seq;
}
@Override
public C.ReversibleSequence append(T t) {
return C.list(t);
}
@Override
public C.ReversibleSequence prepend(C.ReversibleSequence seq) {
return seq;
}
@Override
public C.ReversibleSequence prepend(T t) {
return C.list(t);
}
@Override
public C.ReversibleSequence<$.Binary> zip(C.ReversibleSequence rseq) {
return rseq();
}
@Override
public C.ReversibleSequence<$.Binary> zipAll(C.ReversibleSequence rseq, final T def1, final T2 def2) {
return rseq.map(new $.F1>() {
@Override
public $.Binary apply(T2 t) throws NotAppliedException, $.Break {
return $.T2(def1, t);
}
});
}
}
static class EmptyRange extends EmptySequence implements C.Range, RandomAccess {
private static final EmptyRange INSTANCE = new EmptyRange();
@Override
public T from() {
throw new NoSuchElementException();
}
@Override
public T to() {
throw new NoSuchElementException();
}
@Override
public T last() {
throw new NoSuchElementException();
}
@Override
public boolean containsAll(C.Range r2) {
return false;
}
@Override
@SuppressWarnings("unchecked")
public Comparator order() {
return (Comparator) $.F.NATURAL_ORDER;
}
@Override
public $.Func2 step() {
return $.f2();
}
@Override
public C.Range merge(C.Range r2) {
return r2;
}
@Override
public EmptyRange accept($.Visitor super T> visitor) {
return this;
}
@Override
public EmptyRange forEach($.Visitor super T> visitor) {
return this;
}
@Override
public EmptyRange each($.Visitor super T> visitor) {
return this;
}
@Override
public EmptyRange acceptLeft($.Visitor super T> visitor) {
return this;
}
@Override
public C.Range acceptRight($.Visitor super T> visitor) {
return this;
}
@Override
public C.Range tail(int n) {
return this;
}
@Override
public C.Range reverse() {
return this;
}
@Override
public Iterator reverseIterator() {
return iterator();
}
@Override
public R reduceRight(R identity, $.Func2 accumulator) {
return identity;
}
@Override
public $.Option reduceRight($.Func2 accumulator) {
return $.none();
}
@Override
public $.Option findLast($.Function super T, Boolean> predicate) {
return $.none();
}
}
static class EmptyList extends ImmutableList implements C.List, RandomAccess {
private static final long serialVersionUID = 2142813031316831861L;
private static final EmptyList> INSTANCE = new EmptyList();
private EmptyList() {
super((T[]) new Object[0]);
}
@SuppressWarnings("unchecked")
protected EmptyList singleton() {
return (EmptyList) INSTANCE;
}
@Override
public final boolean isEmpty() {
return true;
}
@Override
public Lang.T2, C.List> split(Lang.Function super T, Boolean> predicate) {
C.List empty = this;
return $.T2(empty, empty);
}
// Preserves singleton property
private Object readResolve() {
return INSTANCE;
}
}
static class EmptyStringList extends EmptyList implements S.List {
private static final long serialVersionUID = 2142813031316831811L;
private static final EmptyStringList INSTANCE = new EmptyStringList();
@SuppressWarnings("unchecked")
protected EmptyStringList singleton() {
return INSTANCE;
}
// Preserves singleton property
private Object readResolve() {
return INSTANCE;
}
}
static class EmptySet extends ImmutableSet implements C.Set, Serializable {
private static final long serialVersionUID = 4142843931316831861L;
private static final EmptySet> INSTANCE = new EmptySet();
private EmptySet() {
super(Collections.EMPTY_SET);
}
@SuppressWarnings("unchecked")
protected EmptySet singleton() {
return (EmptySet) INSTANCE;
}
@Override
public int size() {
return 0;
}
@Override
public boolean contains(Object o) {
return false;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public boolean containsAll(Collection> c) {
return c.isEmpty();
}
@Override
public EmptySet accept($.Visitor super T> visitor) {
return this;
}
@Override
public C.Set onlyIn(Collection extends T> col) {
return C.Set(col);
}
@Override
public C.Set withIn(Collection extends T> col) {
return this;
}
@Override
public C.Set without(Collection super T> col) {
return this;
}
@Override
public C.Set with(Collection extends T> col) {
return C.Set(col);
}
@Override
public C.Set with(T element) {
return C.set(element);
}
@Override
public C.Set with(T element, T... elements) {
return C.set(element, elements);
}
@Override
public C.Set without(T element) {
return null;
}
@Override
public C.Set without(T element, T... elements) {
return null;
}
// Preserves singleton property
private Object readResolve() {
return INSTANCE;
}
}
static class Empty extends EmptyList implements C.ListOrSet {
private static final Empty INSTANCE = new Empty();
@Override
public Empty parallel() {
return this;
}
@Override
public Empty lazy() {
return this;
}
@Override
public Empty filter($.Function super T, Boolean> predicate) {
return this;
}
@Override
public Empty eager() {
return this;
}
@Override
public Empty sequential() {
return this;
}
@Override
public Empty accept($.Visitor super T> visitor) {
return this;
}
@Override
public Empty each($.Visitor super T> visitor) {
return this;
}
@Override
public Empty forEach($.Visitor super T> visitor) {
return this;
}
@Override
public Empty without(Collection super T> col) {
return this;
}
@Override
public Empty without(T element) {
return this;
}
@Override
public Empty without(T element, T... elements) {
return this;
}
@Override
public C.Set with(Collection extends T> col) {
return C.Set(col);
}
@Override
public C.Set with(T element) {
return C.set(element);
}
@Override
public C.Set with(T element, T... elements) {
return C.set(element, elements);
}
@Override
public C.Set onlyIn(Collection extends T> col) {
return C.Set(col);
}
@Override
public Empty withIn(Collection extends T> col) {
return this;
}
@Override
public Empty map($.Function super T, ? extends R> mapper) {
return (Empty) this;
}
@Override
public Empty flatMap($.Function super T, ? extends Iterable extends R>> mapper) {
return (Empty) this;
}
}
//
// static class EmptySortedSet extends EmptyReversibleSequence implements C.SortedSet, Serializable {
//
// private static final long serialVersionUID = 8142843931221131271L;
//
// private EmptySortedSet() {
// }
//
// private static final EmptySortedSet> INSTANCE = new EmptySortedSet();
//
// @Override
// @SuppressWarnings("unchecked")
// protected EmptySortedSet singleton() {
// return (EmptySortedSet) INSTANCE;
// }
//
// @Override
// public EmptySortedSet accept(_.Function super T, ?> visitor) {
// return this;
// }
//
// @Override
// public Comparator super T> comparator() {
// throw new UnsupportedOperationException();
// }
//
// @Override
// public SortedSet subSet(T fromElement, T toElement) {
// return this;
// }
//
// @Override
// public SortedSet headSet(T toElement) {
// return this;
// }
//
// @Override
// public SortedSet tailSet(T fromElement) {
// return this;
// }
//
// // Preserves singleton property
// private Object readResolve() {
// return INSTANCE;
// }
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy