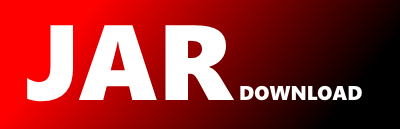
org.osgl.util.ReverseList Maven / Gradle / Ivy
The newest version!
package org.osgl.util;
/*-
* #%L
* Java Tool
* %%
* Copyright (C) 2014 - 2017 OSGL (Open Source General Library)
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import org.osgl.$;
import java.util.EnumSet;
import java.util.Iterator;
import java.util.ListIterator;
import java.util.NoSuchElementException;
/**
* Created with IntelliJ IDEA.
* User: luog
* Date: 28/10/13
* Time: 9:39 PM
* To change this template use File | Settings | File Templates.
*/
class ReverseList extends DelegatingList implements C.List {
private ReverseList(C.List list) {
super(true);
data = list;
}
C.List data() {
return (C.List)data;
}
@Override
protected EnumSet initFeatures() {
EnumSet fs = data().features();
fs.add(C.Feature.READONLY);
return fs;
}
@Override
public ListIterator listIterator(int index) {
return new ReverseListIterator(data().listIterator(size() - index));
}
@Override
public T get(int index) {
return data.get(size() - index - 1);
}
@Override
protected void forEachLeft($.Visitor super T> visitor) throws $.Break {
data().acceptRight(visitor);
}
@Override
protected void forEachRight($.Visitor super T> visitor) throws $.Break {
data().acceptLeft(visitor);
}
@Override
public R reduceLeft(R identity, $.Func2 accumulator) {
return data().reduceRight(identity, accumulator);
}
@Override
public $.Option reduceLeft($.Func2 accumulator) {
return data().reduceRight(accumulator);
}
@Override
public R reduceRight(R identity, $.Func2 accumulator) {
return data().reduceLeft(identity, accumulator);
}
@Override
public $.Option reduceRight($.Func2 accumulator) {
return data().reduceLeft(accumulator);
}
@Override
public T head() throws NoSuchElementException {
return data().last();
}
@Override
public T last() throws NoSuchElementException {
return data().head();
}
@Override
public Iterator reverseIterator() {
return data().iterator();
}
@Override
public C.List reverse() {
return data();
}
@Override
public C.List subList(int fromIndex, int toIndex) {
return data().subList(toIndex - 1, fromIndex - 1);
}
static C.List wrap(C.List list) {
if (list instanceof ReverseList) {
return ((ReverseList)list).data();
}
if (list.size() == 0 && list.is(C.Feature.IMMUTABLE)) {
return Nil.list();
}
return new ReverseList(list);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy