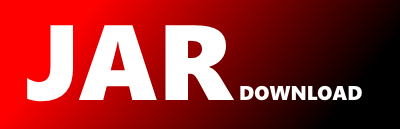
org.osiam.resources.scim.Email Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scim-schema Show documentation
Show all versions of scim-schema Show documentation
SCIM conform data types used by OSIAM services and OSIAM connector4Java for data exchange
/*
* Copyright (C) 2013 tarent AG
*
* Permission is hereby granted, free of charge, to any person obtaining
* a copy of this software and associated documentation files (the
* "Software"), to deal in the Software without restriction, including
* without limitation the rights to use, copy, modify, merge, publish,
* distribute, sublicense, and/or sell copies of the Software, and to
* permit persons to whom the Software is furnished to do so, subject to
* the following conditions:
*
* The above copyright notice and this permission notice shall be
* included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
* IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
* CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
* TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
* SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package org.osiam.resources.scim;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* This class represents a email attribute.
*
*
* For more detailed information please look at the SCIM core schema 2.0, section 3.2
*
*/
public class Email extends MultiValuedAttribute {
@JsonProperty
private Type type;
/**
* Default constructor for Jackson
*/
private Email() {
}
private Email(Builder builder) {
super(builder);
this.type = builder.type;
}
@Override
public String getOperation() {
return super.getOperation();
}
@Override
public String getValue() {
return super.getValue();
}
@Override
public String getDisplay() {
return super.getDisplay();
}
@Override
public boolean isPrimary() {
return super.isPrimary();
}
/**
* Gets the type of the attribute.
*
*
* For more detailed information please look at the SCIM core schema 2.0, section 3.2
*
*
* @return
*
* @return the actual type
*/
public Type getType() {
return type;
}
@Override
public int hashCode() {
final int prime = 31;
int result = super.hashCode();
result = prime * result + ((type == null) ? 0 : type.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (!super.equals(obj)) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
Email other = (Email) obj;
if (type == null) {
if (other.type != null) {
return false;
}
} else if (!type.equals(other.type)) {
return false;
}
return true;
}
/**
* Builder class that is used to build {@link Email} instances
*/
public static class Builder extends MultiValuedAttribute.Builder {
private Type type;
public Builder() {
}
/**
* builds an Builder based of the given Attribute
*
* @param email
* existing Attribute
*/
public Builder(Email email) {
super(email);
type = email.type;
}
@Override
public Builder setOperation(String operation) {
super.setOperation(operation);
return this;
}
@Override
public Builder setDisplay(String display) {
super.setDisplay(display);
return this;
}
@Override
public Builder setValue(String value) {
super.setValue(value);
return this;
}
/**
* Sets the label indicating the attribute's function (See {@link MultiValuedAttribute#getType()}).
*
* @param type
* the type of the attribute
* @return the builder itself
*/
public Builder setType(Type type) {
this.type = type;
return this;
}
@Override
public Builder setPrimary(boolean primary) {
super.setPrimary(primary);
return this;
}
@Override
public Email build() {
return new Email(this);
}
}
/**
* Represents an email type. Canonical values are available as static constants.
*/
public static class Type extends MultiValuedAttributeType {
public static final Type WORK = new Type("work");
public static final Type HOME = new Type("home");
public static final Type OTHER = new Type("other");
public Type(String value) {
super(value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy