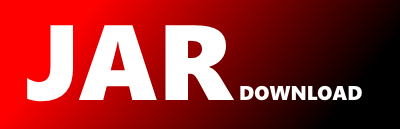
org.ovirt.engine.api.containers.V4VmContainer Maven / Gradle / Ivy
/*
Copyright (c) 2015 Red Hat, Inc.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package org.ovirt.engine.api.containers;
import java.lang.Boolean;
import java.lang.String;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.List;
import java.util.stream.Stream;
import org.ovirt.engine.api.types.V4Cpu;
import org.ovirt.engine.api.types.V4Disk;
import org.ovirt.engine.api.types.V4Vm;
public class V4VmContainer implements V4Vm {
private V4Cpu cpu;
private Date creationTime;
private Boolean deleteProtected;
private List disks;
private String fqdn;
private String id;
private BigInteger memory;
private String name;
private Boolean runOnce;
public V4Cpu getCpu() {
return cpu;
}
public void setCpu(V4Cpu newCpu) {
cpu = newCpu;
}
public boolean hasCpu() {
return cpu != null;
}
public Date getCreationTime() {
return creationTime;
}
public void setCreationTime(Date newCreationTime) {
creationTime = newCreationTime;
}
public boolean hasCreationTime() {
return creationTime != null;
}
public Boolean getDeleteProtected() {
return deleteProtected;
}
public void setDeleteProtected(Boolean newDeleteProtected) {
deleteProtected = newDeleteProtected;
}
public boolean hasDeleteProtected() {
return deleteProtected != null;
}
public List getDisks() {
if (disks == null) {
return Collections.emptyList();
}
else {
return Collections.unmodifiableList(disks);
}
}
public Stream getDisksStream() {
if (disks == null) {
return Stream.empty();
}
else {
return disks.stream();
}
}
public void setDisks(List newDisks) {
if (newDisks == null) {
disks = Collections.emptyList();
}
else {
disks = new ArrayList<>(newDisks);
}
}
public boolean hasDisks() {
return !disks.isEmpty();
}
public String getFqdn() {
return fqdn;
}
public void setFqdn(String newFqdn) {
fqdn = newFqdn;
}
public boolean hasFqdn() {
return fqdn != null;
}
public String getId() {
return id;
}
public void setId(String newId) {
id = newId;
}
public boolean hasId() {
return id != null;
}
public BigInteger getMemory() {
return memory;
}
public void setMemory(BigInteger newMemory) {
memory = newMemory;
}
public boolean hasMemory() {
return memory != null;
}
public String getName() {
return name;
}
public void setName(String newName) {
name = newName;
}
public boolean hasName() {
return name != null;
}
public Boolean getRunOnce() {
return runOnce;
}
public void setRunOnce(Boolean newRunOnce) {
runOnce = newRunOnce;
}
public boolean hasRunOnce() {
return runOnce != null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy