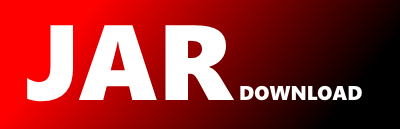
org.ovirt.engine.api.containers.V4VmContainer Maven / Gradle / Ivy
/*
Copyright (c) 2015 Red Hat, Inc.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package org.ovirt.engine.api.containers;
import java.lang.Boolean;
import java.lang.String;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.List;
import java.util.stream.Stream;
import org.ovirt.engine.api.types.V4Cpu;
import org.ovirt.engine.api.types.V4Disk;
import org.ovirt.engine.api.types.V4Vm;
import org.ovirt.engine.api.types.V4VmType;
public class V4VmContainer extends V4Container implements V4Vm {
private V4Cpu cpu;
private Date creationTime;
private Boolean deleteProtected;
private List disks;
private String fqdn;
private String id;
private BigInteger memory;
private String name;
private Boolean runOnce;
private V4VmType type;
public V4Cpu cpu() {
return cpu;
}
public void cpu(V4Cpu newCpu) {
cpu = newCpu;
}
public boolean cpuPresent() {
return cpu != null;
}
public Date creationTime() {
if (creationTime == null) {
return null;
}
else {
return new Date(creationTime.getTime());
}
}
public void creationTime(Date newCreationTime) {
if (newCreationTime == null) {
creationTime = null;
}
else {
creationTime = new Date(newCreationTime.getTime());
}
}
public boolean creationTimePresent() {
return creationTime != null;
}
public boolean deleteProtected() {
return deleteProtected;
}
public void deleteProtected(boolean newDeleteProtected) {
deleteProtected = Boolean.valueOf(newDeleteProtected);
}
public void deleteProtected(Boolean newDeleteProtected) {
deleteProtected = newDeleteProtected;
}
public boolean deleteProtectedPresent() {
return deleteProtected != null;
}
public List disks() {
if (disks == null) {
return Collections.emptyList();
}
else {
return Collections.unmodifiableList(disks);
}
}
public Stream disksStream() {
if (disks == null) {
return Stream.empty();
}
else {
return disks.stream();
}
}
public void disks(List newDisks) {
if (newDisks == null) {
disks = Collections.emptyList();
}
else {
disks = new ArrayList<>(newDisks);
}
}
public boolean disksPresent() {
return !disks.isEmpty();
}
public String fqdn() {
return fqdn;
}
public void fqdn(String newFqdn) {
fqdn = newFqdn;
}
public boolean fqdnPresent() {
return fqdn != null;
}
public String id() {
return id;
}
public void id(String newId) {
id = newId;
}
public boolean idPresent() {
return id != null;
}
public BigInteger memory() {
return memory;
}
public void memory(BigInteger newMemory) {
memory = newMemory;
}
public boolean memoryPresent() {
return memory != null;
}
public String name() {
return name;
}
public void name(String newName) {
name = newName;
}
public boolean namePresent() {
return name != null;
}
public boolean runOnce() {
return runOnce;
}
public void runOnce(boolean newRunOnce) {
runOnce = Boolean.valueOf(newRunOnce);
}
public void runOnce(Boolean newRunOnce) {
runOnce = newRunOnce;
}
public boolean runOncePresent() {
return runOnce != null;
}
public V4VmType type() {
return type;
}
public void type(V4VmType newType) {
type = newType;
}
public boolean typePresent() {
return type != null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy