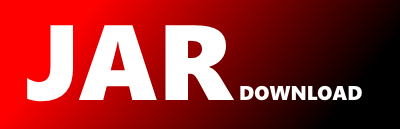
org.ow2.bonita.facade.ejb.ManagementAPIBean Maven / Gradle / Ivy
The newest version!
/**
* Copyright (C) 2006 Bull S. A. S.
* Bull, Rue Jean Jaures, B.P.68, 78340, Les Clayes-sous-Bois
* This library is free software; you can redistribute it and/or modify it under the terms
* of the GNU Lesser General Public License as published by the Free Software Foundation
* version 2.1 of the License.
* This library is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY;
* without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU Lesser General Public License for more details.
* You should have received a copy of the GNU Lesser General Public License along with this
* program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth
* Floor, Boston, MA 02110-1301, USA.
**/
package org.ow2.bonita.facade.ejb;
import java.net.URL;
import java.rmi.RemoteException;
import java.util.Collection;
import javax.ejb.SessionBean;
import javax.ejb.SessionContext;
import org.ow2.bonita.facade.ManagementAPI;
import org.ow2.bonita.facade.def.element.BusinessArchive;
import org.ow2.bonita.facade.def.majorElement.ProcessDefinition;
import org.ow2.bonita.facade.exception.DeploymentException;
import org.ow2.bonita.facade.exception.ProcessNotFoundException;
import org.ow2.bonita.facade.exception.UndeletableInstanceException;
import org.ow2.bonita.facade.exception.UndeletableProcessException;
import org.ow2.bonita.facade.impl.StandardAPIAccessorImpl;
import org.ow2.bonita.facade.internal.InternalManagementAPI;
import org.ow2.bonita.facade.uuid.ProcessDefinitionUUID;
public class ManagementAPIBean implements InternalManagementAPI, SessionBean {
/**
*
*/
private static final long serialVersionUID = 7816343722790053864L;
protected SessionContext ctx = null;
protected ManagementAPI managementAPI;
protected ManagementAPI getAPI() {
EJB2SecurityOwner.setUser(this.ctx.getCallerPrincipal().getName());
if (this.managementAPI == null) {
this.managementAPI = new StandardAPIAccessorImpl().getManagementAPI();
}
return this.managementAPI;
}
public void ejbCreate() {
}
public void ejbActivate() throws RemoteException {
}
public void ejbPassivate() throws RemoteException {
}
public void ejbRemove() throws RemoteException {
}
public void setSessionContext(final SessionContext arg0) throws RemoteException {
this.ctx = arg0;
}
public ProcessDefinition deploy(final BusinessArchive businessArchive) throws DeploymentException {
return getAPI().deploy(businessArchive);
}
public ProcessDefinition deployBar(final URL barFileURL) throws DeploymentException {
return getAPI().deployBar(barFileURL);
}
public ProcessDefinition deployBar(final byte[] barFile) throws DeploymentException {
return getAPI().deployBar(barFile);
}
public void deployClass(final byte[] clazz) throws DeploymentException {
getAPI().deployClass(clazz);
}
public void deployClasses(final Collection classes) throws DeploymentException {
getAPI().deployClasses(classes);
}
public void deployClassesInJar(final byte[] classesArchive) throws DeploymentException {
getAPI().deployClassesInJar(classesArchive);
}
public void undeploy(final ProcessDefinitionUUID processUUID) throws DeploymentException {
getAPI().undeploy(processUUID);
}
public void removeClass(final String className) throws DeploymentException {
getAPI().removeClass(className);
}
public void removeClasses(final String[] classNames) throws DeploymentException {
getAPI().removeClasses(classNames);
}
public void replaceClass(final String className, final byte[] newClazz) throws DeploymentException {
getAPI().replaceClass(className, newClazz);
}
public void deleteProcess(final ProcessDefinitionUUID processUUID)
throws ProcessNotFoundException, UndeletableProcessException, UndeletableInstanceException {
getAPI().deleteProcess(processUUID);
}
public void deleteAllProcesses() throws UndeletableInstanceException,
RemoteException, UndeletableProcessException {
getAPI().deleteAllProcesses();
}
public String getLoggedUser() throws RemoteException {
return getAPI().getLoggedUser();
}
public void addMetaData(String key, String value) throws RemoteException {
getAPI().addMetaData(key, value);
}
public void deleteMetaData(String key) throws RemoteException {
getAPI().deleteMetaData(key);
}
public String getMetaData(String key) throws RemoteException {
return getAPI().getMetaData(key);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy