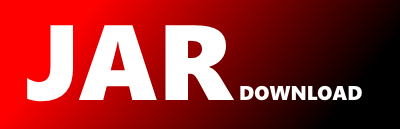
org.ow2.carol.cmi.config.CMIConfig Maven / Gradle / Ivy
/**
* CMI : Cluster Method Invocation
* Copyright (C) 2007,2008 Bull S.A.S.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id:CmiConfig.java 914 2007-05-25 16:48:16Z loris $
* --------------------------------------------------------------------------
*/
package org.ow2.carol.cmi.config;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Enumeration;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import net.jcip.annotations.ThreadSafe;
import org.ow2.carol.cmi.controller.server.ServerClusterViewManager;
import org.ow2.util.log.Log;
import org.ow2.util.log.LogFactory;
/**
* Utility to configure the manager of the cluster view located on the server-side.
* @author The new CMI team
*/
@ThreadSafe
public final class CMIConfig {
/**
* Logger.
*/
private static final Log LOGGER = LogFactory.getLog(CMIConfig.class);
/**
* Filename for the properties.
*/
public static final String CMI_PROPERTIES = "cmi.properties";
/*------------ Begin of default values: ----------------*/
/**
* Default duration to refresh the client view (in millis).
*/
private static final int DEFAULT_REFRESH_TIME = 30000;
/**
* JGroupsClusterViewManager is the default manager.
*/
private static final String DEFAULT_MANAGER =
"org.ow2.carol.cmi.controller.server.impl.jgroups.JGroupsClusterViewManager";
/**
* Default name for binding an instance of ClientClusterViewProvider.
*/
private static final String DEFAULT_PROVIDER_BIND_NAME = "client_provider";
/**
* Default name for binding an instance of the dummy objects representing registry.
*/
private static final String DEFAULT_DUMMY_REGISTRY_BIND_NAME = "dummy_registry";
/**
* Default values for the load.
*/
public static final int DEFAULT_LOAD_FACTOR = 100;
/*------------ End of default values ----------------*/
/**
* Configure only once.
*/
private static boolean configured = false;
/**
* True if the replication is enabled on the server.
*/
private static boolean replicationEnabled = false;
/**
* Properties that are unrecognized by this configurator.
*/
private static final Properties UNRECOGNIZED_PROPS = new Properties();
/**
* Time to refresh the client view.
*/
private static int refreshTime = DEFAULT_REFRESH_TIME;
/**
* Manager for the cluster view at the server-side.
*/
private static Class extends ServerClusterViewManager> serverClusterViewManagerClass = null;
/**
* Name of domain for MBeans.
*/
private static String mbeanDomainName = "CMI";
/**
* Name of CMIAdmin MBean.
*/
private static String cmiAdminMBeanName = "CMIAdmin";
/**
* Protocols that must have a RMI connector.
*/
private static List protocolsOfTheConnectors = new ArrayList();
/**
* Indicate if a provider (of the cluster view) should be bound. True by default.
*/
private static boolean providerBound = true;
/**
* Indicate if the local registry of this server can be accessed to lookup unclustered objects.
*/
private static boolean registryBound = true;
/**
* Name of the ClientClusterViewProvider that is used to bind it.
*/
private static String bindNameForProvider = DEFAULT_PROVIDER_BIND_NAME;
/**
* Name of the DummyContext that is used to bind it.
*/
private static final String bindNameForDummyRegistry = DEFAULT_DUMMY_REGISTRY_BIND_NAME;
/**
* Load factor.
*/
private static int loadFactor = DEFAULT_LOAD_FACTOR;
/**
* True if CMI is embedded.
*/
private static volatile boolean embedded = false;
/**
* Associate a protocol with a list of cluster view provider URLs.
*/
private static Map> clusterViewProviderUrls;
/**
* Path to get the configuration files.
*/
private static String confDir;
/**
* Utility class, so not public constructor.
*/
private CMIConfig() {}
/**
* Initializes the CMI properties with reading the file cmi.properties.
* @throws CMIConfigException if the configuration files are not correct
*/
public static synchronized void init() throws CMIConfigException {
setProperties(Thread.currentThread().getContextClassLoader().getResource(CMIConfig.CMI_PROPERTIES));
}
/**
* Initializes the CMI properties with reading the given properties.
* @param props properties
* @throws CMIConfigException if the configuration files are not correct
*/
@SuppressWarnings("unchecked")
public static synchronized void setProperties(final Properties props) throws CMIConfigException {
// Configure only once
if (configured) {
return;
}
LOGGER.debug("Starting the configuration of CMI...");
LOGGER.debug("CMI props: {0}", props.toString());
String repEnabled = props.getProperty(CMIProperty.REPLICATION_ENABLED.getPropertyName());
if(repEnabled != null) {
replicationEnabled = Boolean.parseBoolean(repEnabled);
}
// Set name of MBean
if(embedded) {
if(replicationEnabled) {
cmiAdminMBeanName = "CMIServer";
} else {
cmiAdminMBeanName = "CMIClient";
}
} else {
String mbName = props.getProperty(CMIProperty.CMIADMIN_MBEAN_NAME.getPropertyName());
if(cmiAdminMBeanName != null) {
cmiAdminMBeanName = mbName;
}
}
for(Enumeration> e = props.propertyNames(); e.hasMoreElements();) {
String k = (String) e.nextElement();
String s = props.getProperty(k);
if (k.equals(CMIProperty.REFRESH_TIME.getPropertyName())) {
// Set refresh time
refreshTime = Integer.parseInt(s);
} else if (k.equals(CMIProperty.REPLICATION_MANAGER_CLASS.getPropertyName())) {
// Set class for manager of cluster views (server-side)
if(replicationEnabled) {
if(s == null) {
s = DEFAULT_MANAGER;
}
try {
serverClusterViewManagerClass = (Class extends ServerClusterViewManager>) Class.forName(s);
} catch (ClassNotFoundException e1) {
LOGGER.error("Cannot load the specified class of manager: {0}.", s);
throw new CMIConfigException("Cannot load the specified class of manager: "+s, e1);
}
}
} else if (k.equals(CMIProperty.MBEAN_DOMAIN_NAME.getPropertyName())){
// Set name of MBean domain
mbeanDomainName = s;
} else if(k.equals(CMIProperty.PROTOCOLS_OF_THE_CONNECTORS.getPropertyName())) {
String[] protocolsArray = s.split(",");
protocolsOfTheConnectors = Arrays.asList(protocolsArray);
} else if(k.equals(CMIProperty.PROVIDER_BOUND.getPropertyName())) {
providerBound = Boolean.parseBoolean(s);
} else if(k.equals(CMIProperty.REGISTRY_BOUND.getPropertyName())) {
registryBound = Boolean.parseBoolean(s);
} else if(k.equals(CMIProperty.PROVIDER_BIND_NAME.getPropertyName())) {
bindNameForProvider = s;
} else if(k.equals(CMIProperty.LOAD_FACTOR.getPropertyName())) {
loadFactor = Integer.parseInt(s);
} else if(k.equals(CMIProperty.CLUSTER_VIEW_PROVIDER_URLS.getPropertyName())) {
try {
clusterViewProviderUrls = ProviderURLsParser.parseMultiprotocol(s);
} catch (MalformedURLException e1) {
LOGGER.error("Cannot parse the following urls: {0}.", s, e1);
throw new CMIConfigException("Cannot parse the following urls: " + s, e1);
}
} else {
UNRECOGNIZED_PROPS.setProperty(k, s);
}
}
configured = true;
LOGGER.debug("Configuration of CMI is done.");
}
/**
* Initializes the CMI properties with reading the file at a given location.
* @param url the URL to load CMI configuration
* @throws CMIConfigException If the configuration files are not correct.
*/
public static synchronized void setProperties(final URL url) throws CMIConfigException {
if(url==null) {
LOGGER.error("The given URL is null");
throw new CMIConfigException("The given URL is null");
}
// Load properties from configuration file
Properties props = UtilConfig.getPropertiesFromURL(url);
setProperties(props);
}
/**
* @return The duration between each refresh of the client view.
*/
public static int getRefreshTime() {
return refreshTime;
}
/**
* @return The class of the ServerClusterViewManager.
*/
public static Class extends ServerClusterViewManager> getServerClusterViewManagerClass() {
return serverClusterViewManagerClass;
}
/**
* Get the value for a CMI property.
* @param key The key.
* @return The associated value.
*/
public static String getCMIProperty(final String key) {
return UNRECOGNIZED_PROPS.getProperty(key);
}
/**
* @return the name of domain for MBeans
*/
public static String getMBeanDomainName() {
return mbeanDomainName;
}
/**
* @return the name of CMIAdmin MBean
*/
public static String getCMIAdminMBeanName() {
return cmiAdminMBeanName;
}
/**
* @param protocol a protocol name
* @return true if a RMI connector must be created for the given protocol
*/
public static boolean isConnectorEnabled(final String protocol) {
return protocolsOfTheConnectors.contains(protocol);
}
/**
* @return true if a provider of the cluster view should be bound
*/
public static boolean isProviderBound() {
return providerBound;
}
/**
* @return name of the ClientClusterViewProvider that is used to bind it
*/
public static String getBindNameForProvider() {
return bindNameForProvider;
}
/**
* @return the bind name of the dummy objects representing registry.
*/
public static String getBindNameForDummyRegistry() {
return bindNameForDummyRegistry;
}
/**
* @return the load factor
*/
public static int getLoadFactor() {
return loadFactor;
}
/**
* @return true if CMI is configured
*/
public static boolean isConfigured() {
return configured;
}
/**
* @return true if the registry is bound
*/
public static boolean isRegistryBound() {
return registryBound;
}
/**
* @return true if CMI is embedded
*/
public static boolean isEmbedded() {
return embedded;
}
/**
* Set that CMI is embedded.
*/
public static void setEmbedded() {
embedded = true;
}
/**
* @param protocol a protocol name
* @return the list of cluster view provider URLs
*/
public static List getClusterViewProviderUrls(final String protocol) {
List res = clusterViewProviderUrls.get(protocol);
if(res == null) {
return new ArrayList();
}
return res;
}
/**
* @return true if the replication is enabled on the server
*/
public static boolean isReplicationEnabled() {
return replicationEnabled;
}
/**
* @return the path to get the configuration files
*/
public static String getConfDir() {
return confDir;
}
/**
* Set the path to get the configuration files.
* @param confDir the path to get the configuration files
*/
public static void setConfDir(final String confDir) {
CMIConfig.confDir = confDir;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy