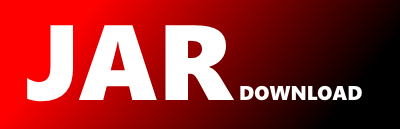
org.ow2.carol.cmi.config.ProviderURLsParser Maven / Gradle / Ivy
/**
* CMI : Cluster Method Invocation
* Copyright (C) 2007 Bull S.A.S.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id:ProviderURLsParser.java 1124 2007-07-27 16:38:35Z loris $
* --------------------------------------------------------------------------
*/
package org.ow2.carol.cmi.config;
import java.net.MalformedURLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.ow2.util.log.Log;
import org.ow2.util.log.LogFactory;
import net.jcip.annotations.Immutable;
/**
* To parse a list of provider URLs.
* @author The new CMI team
*/
@Immutable
public final class ProviderURLsParser {
/**
* Logger.
*/
private static Log logger = LogFactory.getLog(ProviderURLsParser.class);
/**
* Index if the '/' character when no host and no port are set in the URL.
*/
private static final int URL_HOSTPORT_INDEX = 2;
/**
* Private empty constructor because it's a utility class.
*/
private ProviderURLsParser() {}
/**
* Parse scheme.
* @param inputscheme scheme
* @return scheme
* @throws MalformedURLException if malformed scheme
*/
private static String parseScheme(final String inputscheme) throws MalformedURLException {
if (inputscheme.length() == 0) {
throw new MalformedURLException("Scheme cannot be empty");
} else {
if (inputscheme.length() > 1 && inputscheme.endsWith(":")) {
// non-empty scheme must contain at least one character and end with :
return inputscheme.substring(0, inputscheme.length() - 1);
} else {
throw new MalformedURLException("badly formed scheme");
}
}
}
/**
* Returns a list of provider URLs with scheme.
* @param scheme a scheme
* @param inputurl a list provider URLs without their scheme
* @return a list of provider URLs with scheme
* @throws MalformedURLException if malformed name
*/
private static List parseName(final String scheme, final String inputurl) throws MalformedURLException {
// inputurl is expected to start with //
String inputhostport = "";
int n = inputurl.indexOf("/", URL_HOSTPORT_INDEX);
if (n == -1 && inputurl.length() > URL_HOSTPORT_INDEX) {
inputhostport = inputurl.substring(URL_HOSTPORT_INDEX);
} else {
throw new MalformedURLException("The syntax for URL is: ://:(,:)*");
}
return parseHostsPorts(scheme, inputhostport);
}
/**
* Returns a list of provider URLs with scheme.
* @param scheme a scheme
* @param inputhostsports a list provider URLs without their scheme
* @return a list of provider URLs with scheme
* @throws MalformedURLException if parameters are malformed
*/
private static List parseHostsPorts(final String scheme, final String inputhostsports)
throws MalformedURLException {
int start = 0;
List providerURLs = new ArrayList();
do {
int end = inputhostsports.indexOf(',', start);
if (end < 0) {
// the last URL
providerURLs.add(scheme+"://"+inputhostsports.substring(start));
} else {
providerURLs.add(scheme+"://"+inputhostsports.substring(start, end));
}
start = end + 1;
} while (start > 0);
return providerURLs;
}
/**
* Returns a list of provider URLs with scheme.
* @param input a list of provider URLs without scheme (except the first)
* @return a list of provider URLs with scheme
* @throws MalformedURLException if the parameter is malformed
*/
public static List parse(final String input) throws MalformedURLException {
if (input == null || input.length() == 0) {
throw new MalformedURLException("null or empty registry URL");
}
if (input.indexOf("//") == -1) {
throw new MalformedURLException(
"badly formed registry URL " + input);
}
try {
String scheme = parseScheme(input.substring(0, input.indexOf("//")));
return parseName(scheme, input.substring(input.indexOf("//")));
} catch (Exception e) {
logger.error("badly formed registry URL {0}", input, e);
throw new MalformedURLException(
"badly formed registry URL " + input);
}
}
/**
* Return an association of protocol name with a list of provider URLs.
* Example of input: jrmp::rmi://193.145.1.6:1099,193.145.1.9:1099;irmi::rmi://193.145.1.6:1098;iiop::iiop://193.145.1.6:2001.
* @param input a sequential representation of an association of protocol name with a list of provider URLs
* @return an association of protocol name with a list of provider URLs
* @throws MalformedURLException if the parameter is malformed
*/
public static Map> parseMultiprotocol(final String input) throws MalformedURLException {
Map> mapProtocol2ProviderUrls = new HashMap>();
String[] seqProtocol2ProviderUrls = input.split(";");
for(String protocol2ProviderUrls : seqProtocol2ProviderUrls) {
if(!protocol2ProviderUrls.equals("")) {
String[] protocolAndProviderUrls = protocol2ProviderUrls.split("::");
try {
mapProtocol2ProviderUrls.put(protocolAndProviderUrls[0], parse(protocolAndProviderUrls[1]));
} catch(ArrayIndexOutOfBoundsException e) {
logger.error("badly formed association {0}", input, e);
throw new MalformedURLException();
}
}
}
return mapProtocol2ProviderUrls;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy