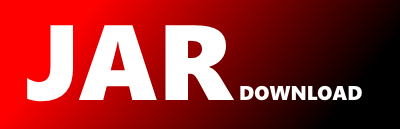
org.ow2.cmi.lb.policy.FirstAvailable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cmi-lb Show documentation
Show all versions of cmi-lb Show documentation
Define a set of basic load-balancing policies and utilities.
/**
* CMI : Cluster Method Invocation
* Copyright (C) 2007,2008 Bull S.A.S.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id:FirstAvailablePolicy.java 1124 2007-07-27 16:38:35Z loris $
* --------------------------------------------------------------------------
*/
package org.ow2.cmi.lb.policy;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Random;
import net.jcip.annotations.ThreadSafe;
import org.ow2.cmi.lb.LoadBalanceable;
import org.ow2.cmi.lb.NoLoadBalanceableException;
import org.ow2.cmi.lb.strategy.IStrategy;
import org.ow2.cmi.lb.strategy.NoStrategy;
import org.ow2.util.log.Log;
import org.ow2.util.log.LogFactory;
/**
* Implementation of a policy of load-balancing that always favors the first available load-balanceable.
* When the list of servers change, we try to keep the current elected load-balanceable if it still exists.
* If the list order changed, we were switching to another load-balanceable.
* @param The type of objects that are load-balanced
* @author The new CMI team
*/
@ThreadSafe
public final class FirstAvailable extends AbstractPolicy {
/**
* Logger.
*/
private static final Log LOGGER = LogFactory.getLog(FirstAvailable.class);
/**
* The current elected node.
*/
private T nodeSelected = null;
/**
* Random numbers.
*/
private final Random nodeRandom = new Random();
/**
* Build the first available policy.
*/
public FirstAvailable() {
}
/**
* Chooses the first load-balanceable available among the list of load-balanceable.
* @param loadBalanceables the list of load-balanceables
* @throws NoLoadBalanceableException if no server available
* @return the chosen load-balanceable
*/
@Override
public synchronized T choose(final Collection loadBalanceables) throws NoLoadBalanceableException {
if (loadBalanceables == null || loadBalanceables.isEmpty()) {
LOGGER.error("The given list is null or empty: " + loadBalanceables);
throw new NoLoadBalanceableException("The given list is null or empty: " + loadBalanceables);
}
List cmiRefsWithStrategy;
IStrategy strategy = getStrategy();
if(strategy != null) {
cmiRefsWithStrategy = strategy.choose(loadBalanceables);
// If no server corresponds at this strategy, we don't use it
if(cmiRefsWithStrategy.isEmpty()) {
cmiRefsWithStrategy = new ArrayList(loadBalanceables);
}
} else {
cmiRefsWithStrategy = new ArrayList(loadBalanceables);
}
if (nodeSelected != null && cmiRefsWithStrategy.contains(nodeSelected)) {
return nodeSelected;
} else {
int index;
// The initial pointer depends on the strategy
if(strategy != null && !(strategy instanceof NoStrategy)) {
// Use the first element chosen by the strategy
index = 0;
} else {
// No strategy, choose randomly the first element
index = nodeRandom.nextInt(cmiRefsWithStrategy.size());
}
nodeSelected = cmiRefsWithStrategy.get(index);
LOGGER.debug("New reference selected {1} at index {0}", index, nodeSelected);
return nodeSelected;
}
}
@Override
public String toString() {
return "FirstAvailable[nodeSelected: "
+ nodeSelected + " - strategy: " + getStrategy() + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy