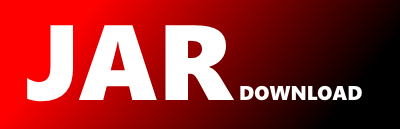
org.ow2.cmi.lb.data.PolicyData Maven / Gradle / Ivy
/**
* CMI : Cluster Method Invocation
* Copyright (C) 2007,2008 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA
* --------------------------------------------------------------------------
* $Id: PolicyData.java 2122 2008-09-27 09:41:35Z loris $
* --------------------------------------------------------------------------
*/
package org.ow2.cmi.lb.data;
import java.io.Serializable;
import java.util.Date;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import net.jcip.annotations.ThreadSafe;
/**
* Contains data for a policy of load-balancing.
* @author The new CMI team
*/
@ThreadSafe
public final class PolicyData implements Serializable, Cloneable {
/**
* Id for serializable class.
*/
private static final long serialVersionUID = 7135811410484151772L;
/**
* Type of policy.
*/
private volatile String policyType;
/**
* Properties for the policy of load-balancing.
*/
private volatile ConcurrentHashMap properties =
new ConcurrentHashMap();
/**
* Date of properties.
* When no property is used, the date is 0.
*/
private volatile long dateOfProperties;
/**
* Type of strategy.
*/
private volatile String strategyType;
/**
* Constructs data for load-balancing with the specified policy, strategy and properties.
* Generates a number of version for each datum.
* @param policyType a type of LB policy
* @param strategyType a type of LB strategy
* @param properties properties for the given LB policy
*/
public PolicyData(final String policyType, final String strategyType, final Map properties) {
this.policyType = policyType;
this.strategyType = strategyType;
if(properties == null || properties.isEmpty()) {
// Just an optimization to avoid of downloading an empty map
dateOfProperties = 0;
} else {
// Gets the date that will be a number of version for the properties
Date date = new Date();
dateOfProperties = date.getTime();
this.properties.putAll(properties);
}
}
/**
* Constructs data for load-balancing with the specified policy, strategy and properties.
* Uses the current number of version for each datum.
* @param policyType a type of LB policy
* @param strategyType a type of LB strategy
* @param properties properties for the given LB policy
* @param dateOfProperties a date of properties
*/
public PolicyData(
final String policyType, final String strategyType,
final Map properties, final long dateOfProperties) {
this.policyType = policyType;
this.strategyType = strategyType;
this.dateOfProperties = dateOfProperties;
if(dateOfProperties != 0) {
this.properties.putAll(properties);
}
}
/**
* @return the type of policy
*/
public String getPolicyType() {
return policyType;
}
/**
* Sets the type of policy.
* @param lbPolicyType a type of policy
*/
public void setPolicyType(final String lbPolicyType) {
policyType = lbPolicyType;
}
/**
* Returns the value of the property with the given name.
* @return properties for policy
*/
public Map getProperties() {
return properties;
}
/**
* Returns the value of the property with the given name.
* @param propertyName a name of a property
* @return the value of the property with the given name
*/
public Object getProperty(final String propertyName) {
return properties.get(propertyName);
}
/**
* Set new properties to the policy (remove the previous).
* @param properties properties
*/
public void setProperties(final Map properties) {
properties.clear();
properties.putAll(properties);
// Compute a new date to identify this version of properties
Date date = new Date();
dateOfProperties = date.getTime();
}
/**
* Adds a property to the policy.
* @param propertyName a name of a property
* @param propertyValue a value of a property
*/
public void setProperty(final String propertyName, final Object propertyValue) {
properties.put(propertyName, propertyValue);
// Compute a new date to identify this version of properties
Date date = new Date();
dateOfProperties = date.getTime();
}
/**
* @return the date of properties
*/
public long getDateOfProperties() {
return dateOfProperties;
}
/**
* @return the type of strategy for load-balancing
*/
public String getStrategyType() {
return strategyType;
}
/**
* Sets the type of strategy.
* @param lbStrategyType a type of policy
*/
public void setStrategyType(final String lbStrategyType) {
strategyType = lbStrategyType;
}
@Override
public boolean equals(final Object object) {
if(object == null || !(object instanceof PolicyData)) {
return false;
}
PolicyData lbPolicyData = (PolicyData) object;
return policyType.equals(lbPolicyData.policyType)
&& strategyType.equals(lbPolicyData.strategyType)
&& properties.equals(lbPolicyData.properties)
&& dateOfProperties == lbPolicyData.dateOfProperties;
}
public int hashCode() {
return (int) (policyType.hashCode() + strategyType.hashCode() + properties.hashCode() + dateOfProperties);
}
@Override
public String toString() {
return "[policyType=" + policyType + ",strategyType=" + strategyType
+ ",properties=" + properties + ",dateOfProperties=" + dateOfProperties + "]";
}
@Override
public PolicyData clone() throws CloneNotSupportedException {
PolicyData lbPolicyData = (PolicyData) super.clone();
lbPolicyData.properties = new ConcurrentHashMap(properties);
return lbPolicyData;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy