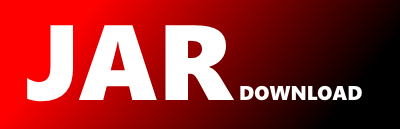
org.ow2.cmi.lb.decision.BasicDecisionManager Maven / Gradle / Ivy
/**
* Copyright (C) 2007-2008 Bull S.A.S.
*
* CMI : Cluster Method Invocation
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: BasicDecisionManager.java 1811 2008-05-30 16:16:32Z loris $
* --------------------------------------------------------------------------
*/
package org.ow2.cmi.lb.decision;
import net.jcip.annotations.Immutable;
/**
* Encapsulates a decision and an eventually returned value.
* @param the type of the returned value (can be {@link Void})
* @author The new CMI team
*/
@Immutable
public final class BasicDecisionManager implements DecisionManager {
/**
* Chosen Decision.
*/
private final Decision decision;
/**
* Value associated with return decision.
*/
private final ReturnType retVal;
/**
* An exception to throw.
*/
private final Throwable throwable;
/**
* Creates a RETRY decision.
*/
private BasicDecisionManager() {
this.decision = Decision.RETRY;
this.retVal = null;
this.throwable = null;
}
/**
* Creates a RETURN decision.
* @param retVal the value to return
*/
private BasicDecisionManager(final ReturnType retVal) {
this.decision = Decision.RETURN;
this.retVal = retVal;
this.throwable = null;
}
/**
* Creates a THROW decision.
* @param decision value of the decision
*/
private BasicDecisionManager(final Throwable throwable) {
this.decision = Decision.THROW;
this.retVal = null;
this.throwable = throwable;
}
/**
* Creates a RETRY decision.
* @return RETRY
*/
public static BasicDecisionManager doRetry() {
return new BasicDecisionManager();
}
/**
* Creates a THROW decision.
* @param throwable an exception to throw
* @return THROW
*/
public static BasicDecisionManager doThrow(final Throwable throwable) {
return new BasicDecisionManager(throwable);
}
/**
* Creates a RETURN decision.
* @param retVal the value to return
* @return RETURN
* @param the type of the returned value (can be {@link Void})
*/
public static BasicDecisionManager doReturn(final ReturnType retVal) {
return new BasicDecisionManager(retVal);
}
/**
* @return the decision
*/
public Decision getDecision() {
return decision;
}
/**
* @return the returned value
*/
public ReturnType getRetVal() {
return retVal;
}
/**
* @return an exception to throw
*/
public Throwable getThrowable() {
return throwable;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy