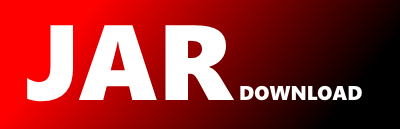
org.ow2.cmi.lb.decision.DecisionUtil Maven / Gradle / Ivy
/**
* CMI : Cluster Method Invocation
* Copyright (C) 2007,2008 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA
* --------------------------------------------------------------------------
* $Id:DecisionUtil.java 1123 2007-07-27 10:07:09Z loris $
* --------------------------------------------------------------------------
*/
package org.ow2.cmi.lb.decision;
import java.io.EOFException;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.UndeclaredThrowableException;
import java.net.SocketException;
import java.rmi.ConnectException;
import java.rmi.ConnectIOException;
import java.rmi.NoSuchObjectException;
import java.rmi.ServerException;
import java.rmi.UnmarshalException;
import java.util.Set;
import javax.ejb.EJBException;
import javax.naming.CommunicationException;
import javax.naming.NameNotFoundException;
import javax.naming.ServiceUnavailableException;
import net.jcip.annotations.Immutable;
import org.ow2.cmi.controller.common.ClusterViewManager;
import org.ow2.cmi.lb.LoadBalanceable;
import org.ow2.cmi.pool.NamingPoolException;
import org.ow2.cmi.reference.CMIReference;
import org.ow2.cmi.reference.ObjectNotFoundException;
import org.ow2.util.log.Log;
import org.ow2.util.log.LogFactory;
/**
* Utilities for failover.
* @author The new CMI team
*/
@Immutable
public final class DecisionUtil {
/**
* Logger.
*/
private static Log logger = LogFactory.getLog(DecisionUtil.class);
/**
* Private default constructor.
*/
private DecisionUtil() {
}
/**
* Checks if the distributor must fail over depending of the exception.
* @param ex The exception to check
* @param clusterViewManager a manager of the cluster view
* @param loadBalanceable a reference on a clustered object
* @return true if the distributor must fail over
*/
public static boolean mustFailoverOnInvoke(
final Throwable ex,
final ClusterViewManager clusterViewManager,
final LoadBalanceable loadBalanceable) {
Throwable cause = ex;
// Extract cause
while(cause instanceof UndeclaredThrowableException
|| cause instanceof InvocationTargetException
|| cause instanceof IllegalStateException
|| cause instanceof NamingPoolException) {
if(cause instanceof UndeclaredThrowableException) {
cause = ((UndeclaredThrowableException) cause).getUndeclaredThrowable();
} else if(cause instanceof InvocationTargetException) {
cause = ((InvocationTargetException) cause).getTargetException();
} else {
cause = cause.getCause();
}
}
if(cause instanceof EJBException) {
if(clusterViewManager == null) {
logger.error("Cannot retrieve the manager of the cluster view to resolve this EJBException: ", cause);
return false;
}
cause = ((EJBException) cause).getCausedByException();
// Check if the wrapped exception is an application exception
String objectName = null;
try {
objectName = ((CMIReference) loadBalanceable).getObjectName();
Set applicationExceptionNames = clusterViewManager.getApplicationExceptionNames(objectName);
if(applicationExceptionNames.contains(cause.getClass().getName())) {
return false;
}
} catch (ObjectNotFoundException e) {
logger.error("Object name not found: {0}", objectName, e);
}
return true;
}
if (cause instanceof UnmarshalException) {
cause = cause.getCause();
if ((cause instanceof EOFException)
|| (cause instanceof SocketException)) {
return true;
}
}
if (cause instanceof ConnectException
|| cause instanceof ConnectIOException
|| cause instanceof NoSuchObjectException
|| cause instanceof org.omg.CORBA.TRANSIENT
|| cause instanceof org.omg.CORBA.COMM_FAILURE
|| cause instanceof ServiceUnavailableException
|| cause instanceof NameNotFoundException
|| cause instanceof CommunicationException
|| cause instanceof ServerException) {
return true;
}
return false;
}
/**
* Checks if the distributor must fail over depending of the exception.
* @param ex The exception to check
* @param clusterViewManager a manager of the cluster view
* @param loadBalanceable a reference on a clustered object
* @return true if the distributor must fail over
*/
public static boolean mustFailoverOnLookup(
final Throwable ex,
final ClusterViewManager clusterViewManager,
final LoadBalanceable loadBalanceable) {
return mustFailoverOnInvoke(ex, clusterViewManager, loadBalanceable);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy