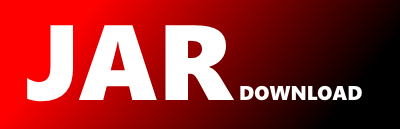
org.ow2.cmi.lb.policy.HASingleton Maven / Gradle / Ivy
/**
* CMI : Cluster Method Invocation
* Copyright (C) 2007,2008 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA
* --------------------------------------------------------------------------
* $Id: HASingleton.java 2122 2008-09-27 09:41:35Z loris $
* --------------------------------------------------------------------------
*/
package org.ow2.cmi.lb.policy;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import net.jcip.annotations.ThreadSafe;
import org.ow2.cmi.lb.LoadBalanceable;
import org.ow2.cmi.lb.NoLoadBalanceableException;
import org.ow2.cmi.lb.strategy.IStrategy;
import org.ow2.util.log.Log;
import org.ow2.util.log.LogFactory;
/**
* Implementation of a policy of load-balancing that always favors one server in the cluster.
* @param The type of objects that was load-balanced
* @author The new CMI team
*/
@ThreadSafe
public class HASingleton extends AbstractPolicy {
/**
* Logger.
*/
private static final Log LOGGER = LogFactory.getLog(HASingleton.class);
/**
* The list of servers that contains the order of selection (index 0 is the first chosen).
*/
private volatile List serverRefs = new ArrayList();
/**
* A policy to use if no singleton is declared.
*/
private volatile IPolicy policy;
/**
* Construct a new instance of policy ha-singleton with the policy first available as rescue.
*/
public HASingleton() {
try {
policy = new FirstAvailable();
} catch (Exception e) {
LOGGER.debug("Cannot instanciate FirstAvailable.class", e);
}
}
/**
* Always choose the same server (called singleton) on any client.
* @param cmiReferences a list of references
* @throws NoLoadBalanceableException if no server available
* @return the singleton
*/
@Override
public synchronized T choose(final Collection cmiReferences)
throws NoLoadBalanceableException {
if (cmiReferences == null || cmiReferences.isEmpty()) {
LOGGER.error("The given list is null or empty: " + cmiReferences);
throw new NoLoadBalanceableException("The given list is null or empty: " + cmiReferences);
}
// Search for an available singleton
for(String serverRef : serverRefs) {
for(T cmiReference : cmiReferences) {
if(serverRef.equals(cmiReference.getServerRef().getProviderURL())) {
LOGGER.debug("Found a singleton: {0}", serverRef);
return cmiReference;
}
}
}
// None singleton is available, use an other policy.
return policy.choose(cmiReferences);
}
/**
* Sets a strategy to modify the behavior of the rescue policy.
* It will be used only if no singleton is declared.
* @param strategy a strategy of load-balancing
*/
@Override
public void setStrategy(final IStrategy strategy) {
super.setStrategy(strategy);
policy.setStrategy(strategy);
}
/**
* Return the order to elect a singleton in the cluster.
* The first element will be the first elected.
* @return a list of reference on servers (e.g. {rmi://localhost:9000})
*/
public List getSingletons() {
return serverRefs;
}
/**
* Set the order to elect a singleton in the cluster.
* The first element will be the first elected.
* @param serverRefs a list of reference on servers (e.g. {rmi://localhost:9000})
*/
public synchronized void setSingletons(final List serverRefs) {
this.serverRefs=serverRefs;
}
/**
* Add a server in the list of singleton at the first position (it will be the new master).
* @param serverRef a reference on server (e.g. rmi://localhost:9000)
*/
public synchronized void setSingleton(final String serverRef) {
serverRefs.add(0, serverRef);
}
/**
* @return the reference on server that is at the first position
*/
public synchronized String getSingleton() {
return serverRefs.get(0);
}
@Override
public String toString() {
return "HASingleton[ServerRefs: " + serverRefs
+ " - master: " + serverRefs.get(0)
+ " - Rescue policy: " + policy
+ " - Rescue strategy: " + getStrategy() + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy