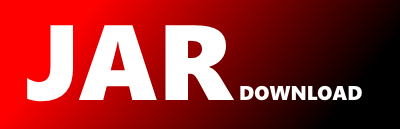
org.ow2.cmi.lb.policy.Random Maven / Gradle / Ivy
/**
* CMI : Cluster Method Invocation
* Copyright (C) 2007,2008 Bull S.A.S.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id:RandomPolicy.java 1124 2007-07-27 16:38:35Z loris $
* --------------------------------------------------------------------------
*/
package org.ow2.cmi.lb.policy;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import net.jcip.annotations.ThreadSafe;
import org.ow2.cmi.lb.LoadBalanceable;
import org.ow2.cmi.lb.NoLoadBalanceableException;
import org.ow2.cmi.lb.strategy.IStrategy;
import org.ow2.util.log.Log;
import org.ow2.util.log.LogFactory;
/**
* Implementation of a policy of load-balancing that always selects randomly a load-balanceable.
* @param The type of objects that are load-balanced
* @author The new CMI team
*/
@ThreadSafe
public final class Random extends AbstractPolicy {
/**
* Logger.
*/
private static final Log LOGGER = LogFactory.getLog(Random.class);
/**
* Random numbers.
*/
private final java.util.Random random = new java.util.Random();
/**
* Build the random policy.
*/
public Random() {
}
/**
* Chooses randomly a load-balanceable among the list of load-balanceables.
* @param loadBalanceables the list of load-balanceables
* @throws NoLoadBalanceableException if no server available
* @return the chosen load-balanceable
*/
@Override
public synchronized T choose(final Collection loadBalanceables) throws NoLoadBalanceableException{
if (loadBalanceables == null || loadBalanceables.isEmpty()) {
LOGGER.error("The given list is null or empty: " + loadBalanceables);
throw new NoLoadBalanceableException("The given list is null or empty: " + loadBalanceables);
}
List cmiRefsWithStrategy;
IStrategy strategy = getStrategy();
if(strategy != null) {
cmiRefsWithStrategy = strategy.choose(loadBalanceables);
// If no server corresponds at this strategy, we don't use it
if(cmiRefsWithStrategy.isEmpty()) {
cmiRefsWithStrategy = new ArrayList(loadBalanceables);
}
} else {
cmiRefsWithStrategy = new ArrayList(loadBalanceables);
}
int index = random.nextInt(cmiRefsWithStrategy.size());
return cmiRefsWithStrategy.get(index);
}
@Override
public String toString() {
return "Random[strategy: " + getStrategy() + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy