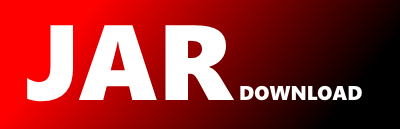
org.ow2.cmi.lb.strategy.LocalPreference Maven / Gradle / Ivy
/**
* CMI : Cluster Method Invocation
* Copyright (C) 2007,2008 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA
* --------------------------------------------------------------------------
* $Id:LocalPreference.java 1124 2007-07-27 16:38:35Z loris $
* --------------------------------------------------------------------------
*/
package org.ow2.cmi.lb.strategy;
import java.net.InetAddress;
import java.net.NetworkInterface;
import java.net.SocketException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import net.jcip.annotations.Immutable;
import org.ow2.cmi.controller.client.ClientClusterViewManager;
import org.ow2.cmi.controller.common.ClusterViewManager;
import org.ow2.cmi.controller.server.ServerClusterViewManager;
import org.ow2.cmi.lb.LoadBalanceable;
import org.ow2.cmi.reference.ServerRef;
import org.ow2.util.log.Log;
import org.ow2.util.log.LogFactory;
/**
* Defines a strategy that enable the local preference.
* @param The type of objects that are load-balanced
* @author The new CMI team
*/
@Immutable
public final class LocalPreference implements IStrategy {
/**
* Logger.
*/
private static final Log LOGGER = LogFactory.getLog(LocalPreference.class);
/**
* The manager of the cluster view.
*/
private final ClusterViewManager clusterViewManager;
/**
* Constructs a strategy for load-factor.
* @param clusterViewManager the manager of the cluster view
*/
public LocalPreference(final ClusterViewManager clusterViewManager) {
this.clusterViewManager = clusterViewManager;
}
/**
* Returns a list of CMIReference that references the local servers.
* @param cmiRefs a list of CMIReference
* @return a list of CMIReference that references the local servers
*/
public List choose(final Collection cmiRefs) {
List localServers = new ArrayList();
for(T cmiRef : cmiRefs) {
// Gets the reference of server that have deployed the object
ServerRef serverRef = cmiRef.getServerRef();
// Gets its address
InetAddress inetAddress = serverRef.getInetAddress();
try {
// Checks if the addresses match
if(isLocal(inetAddress)) {
// Local address: adds reference in the first position
localServers.add(cmiRef);
}
} catch (SocketException e) {
LOGGER.error("Cannot know if is local or not", e);
throw new RuntimeException("Cannot know if is local or not", e);
}
}
return localServers;
}
/**
* Tests if an address is local.
* @param inetAddress an address
* @return true if the given address is local
* @throws SocketException if an I/O error occurs
*/
private boolean isLocal(final InetAddress inetAddress) throws SocketException {
if(clusterViewManager instanceof ClientClusterViewManager) {
if(NetworkInterface.getByInetAddress(inetAddress)!=null) {
return true;
}
} else if(clusterViewManager instanceof ServerClusterViewManager) {
if(inetAddress.equals(((ServerClusterViewManager) clusterViewManager).getInetAddress())) {
return true;
}
}
return false;
}
@Override
public String toString() {
return "LocalPreference";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy