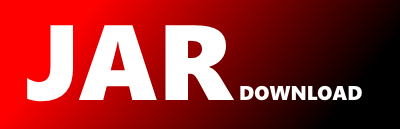
org.ow2.cmi.loader.LoaderUtil Maven / Gradle / Ivy
The newest version!
/**
* CMI : Cluster Method Invocation
* Copyright (C) 2008 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA
* --------------------------------------------------------------------------
* $Id: LoaderUtil.java 1801 2008-05-19 17:22:05Z eyindanga $
* --------------------------------------------------------------------------
*/
package org.ow2.cmi.loader;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
/**
* Helper for default archive Loader.
*
* @author The new CMI team
*
*/
public class LoaderUtil {
/**
* Gets an URL from a given file.
*
* @param file
* the given file
* @return the URL that has been built
* @throws Exception any.
*/
public static URL fileToURL2(final File file) throws Exception {
// check
if (file == null) {
throw new Exception("Invalid File. It is null");
}
// transform
try {
return file.toURI().toURL();
} catch (MalformedURLException e) {
throw new Exception("Cannot get URL from the given file '" + file
+ "'.", e);
}
}
/**
* Gets the URL of the archive.
*
* @param file
* the archive.
* @return URL of archive
*/
public static URL[] getArchiveUrls(final File file) {
List clUser = new ArrayList();
try {
clUser.add(fileToURL2(file));
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return clUser.toArray(new URL[0]);
}
/**
* Create temporary jar with content of archiveData
.
*
* @param archiveName Name of the archive.
* @param archiveData Byte array.
* @return Temporary file containing the byte array.
* @throws IOException if an exception of this type occurs.
*/
public static File createTempJarFile(final String archiveName,
final byte[] archiveData) throws IOException {
File tempFile = File.createTempFile(archiveName, ".jar");
tempFile.deleteOnExit();
FileOutputStream tempFos = new FileOutputStream(tempFile);
tempFos.write(archiveData);
tempFos.close();
return tempFile;
}
/**
* Build an Id for the archive before sharing it to all the cluster.
*
* @param archiveHost
* owner of the archive.
* @param location location of the archive.
* @return The archive Id.
*/
public static ArchiveId buildArchiveId(final String archiveHost,
final String location) {
return new ArchiveId(archiveHost, location);
}
/**
* Build an archiveId
> from string fomatted like
* host_address+archive_location
.
*
* @param stringArchiveId
* The formatted String for given archiveId
* @return ArchiveId
*/
public static ArchiveId buildArchiveIdFromFormattedId(
final String stringArchiveId) {
String archiveHost = stringArchiveId.substring(0, stringArchiveId
.indexOf(ArchiveId.SEPARATOR));
String archiveLocation = stringArchiveId.substring(stringArchiveId
.indexOf(ArchiveId.SEPARATOR) + 1, stringArchiveId.length());
ArchiveId ret = buildArchiveId(archiveHost, archiveLocation);
ret.setName(getNameFromLocation(ret.getLocation()));
return ret;
}
/**
* Gets the archive name from location
.
* @param location archive location.
* @return The canonical name of the archive. /toto.jar
will return toto
*/
private static String getNameFromLocation(final String location) {
if (location == null) {
return ArchiveId.DEFAULT_NAME;
}
int ind = location.lastIndexOf("/");
if (ind != -1) {
return location.substring(ind + 1, location.length() - 4);
}
return location.substring(0, location.length() - 4);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy