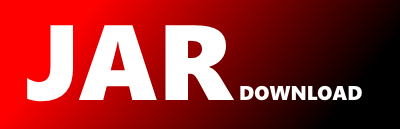
org.ow2.easybeans.console.registry.service.RegistryService Maven / Gradle / Ivy
/**
* EasyBeans
* Copyright (C) 2008-2009 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id$
* --------------------------------------------------------------------------
*/
package org.ow2.easybeans.console.registry.service;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
import javax.management.AttributeNotFoundException;
import javax.management.InstanceNotFoundException;
import javax.management.MBeanException;
import javax.management.MBeanServer;
import javax.management.MBeanServerConnection;
import javax.management.MBeanServerFactory;
import javax.management.MalformedObjectNameException;
import javax.management.ObjectName;
import javax.management.ReflectionException;
import javax.management.openmbean.CompositeData;
import org.apache.felix.ipojo.annotations.Component;
import org.apache.felix.ipojo.annotations.Instantiate;
import org.apache.felix.ipojo.annotations.Invalidate;
import org.apache.felix.ipojo.annotations.Provides;
import org.apache.felix.ipojo.annotations.ServiceProperty;
import org.apache.felix.ipojo.annotations.Validate;
import org.ow2.kerneos.core.service.KerneosSimpleService;
import org.ow2.util.log.Log;
import org.ow2.util.log.LogFactory;
/**
* Manages the POJO service used by flex clients.
* @author Florent Benoit
*/
@Component
@Instantiate
@Provides
public class RegistryService implements KerneosSimpleService {
@ServiceProperty(name = KerneosSimpleService.ID, value = "RegistryService")
private String id;
/**
* Connection to the MBean server
*/
private transient MBeanServerConnection mbeanServerConnection;
/**
* The logger
*/
public static Log logger = LogFactory.getLog(RegistryService.class);
/**
* Default constructor
*/
public RegistryService() {
}
@Validate
private void start() {
logger.debug("");
try {
this.mbeanServerConnection = getMBeanServerConnection();
} catch (Exception e) {
logger.error("Cannot get a connection to the MBean server");
}
}
@Invalidate
private void stop() {
logger.debug("");
}
/**
* @param oNameStr A string representation of the object name.
* @return an object name from the given string.
*/
protected ObjectName getObjectName(final String oNameStr) {
ObjectName oName = null;
try {
oName = new ObjectName(oNameStr);
} catch (MalformedObjectNameException e) {
logger.error("Cannot create a new ObjectName object. The format of the string does not correspond to a valid ObjectName.", e);
} catch (NullPointerException e) {
logger.error("Cannot create a new ObjectName object. The name is null", e);
}
return oName;
}
/**
* @return the list of {@link RegistryProtocol} objects
*/
public List getRegistryProtocols() {
logger.debug("");
// ObjectName
String pattern = "*:j2eeType=JNDIResource,name=jrmp,*";
ObjectName oName = getObjectName(pattern);
Set objectNames = null;
try {
objectNames = getMBeanServerConnection().queryNames(oName, null);
} catch (IOException e) {
logger.error("Cannot get a connection to the MBean server", e);
} catch (Exception e) {
logger.error("Cannot get a connection to the MBean server", e);
}
List list = new ArrayList();
// For each ObjectName, get data
if (objectNames != null) {
for (ObjectName objectName : objectNames) {
RegistryProtocol registryProtocol = new RegistryProtocol();
list.add(registryProtocol);
// Provider URL
String providerURL = null;
try {
providerURL = (String) this.mbeanServerConnection.getAttribute(objectName, "ProviderURL");
} catch (MBeanException e) {
logger.error("Could not found a value of the attribute 'ProviderURL' of the objectName" + objectName, e);
} catch (AttributeNotFoundException e) {
logger.error("Could not found a value of the attribute 'ProviderURL' of the objectName" + objectName, e);
} catch (InstanceNotFoundException e) {
logger.error("Could not found a value of the attribute 'ProviderURL' of the objectName" + objectName, e);
} catch (ReflectionException e) {
logger.error("Could not found a value of the attribute 'ProviderURL' of the objectName" + objectName, e);
} catch (IOException e) {
logger.error("Could not found a value of the attribute 'ProviderURL' of the objectName" + objectName, e);
}
registryProtocol.setProviderURL(providerURL);
// Name
String name = null;
try {
name = (String) this.mbeanServerConnection.getAttribute(objectName, "Name");
} catch (MBeanException e) {
logger.error("Could not found a value of the attribute 'Name' of the objectName" + objectName, e);
} catch (AttributeNotFoundException e) {
logger.error("Could not found a value of the attribute 'Name' of the objectName" + objectName, e);
} catch (InstanceNotFoundException e) {
logger.error("Could not found a value of the attribute 'Name' of the objectName" + objectName, e);
} catch (ReflectionException e) {
logger.error("Could not found a value of the attribute 'Name' of the objectName" + objectName, e);
} catch (IOException e) {
logger.error("Could not found a value of the attribute 'Name' of the objectName" + objectName, e);
}
registryProtocol.setName(name);
//retrieve the list of pair name/value of each JNDI entry
CompositeData compositeDatas[] = null;
try {
compositeDatas = (CompositeData[]) getMBeanServerConnection().invoke(objectName, "listJNDIENtries", null, null);
} catch (InstanceNotFoundException e) {
logger.error("Could not get back data of the 'listJNDIENtries' method of the objectName" + objectName, e);
} catch (MBeanException e) {
logger.error("Could not get back data of the 'listJNDIENtries' method of the objectName" + objectName, e);
} catch (ReflectionException e) {
logger.error("Could not get back data of the 'listJNDIENtries' method of the objectName" + objectName, e);
} catch (IOException e) {
logger.error("Could not get back data of the 'listJNDIENtries' method of the objectName" + objectName, e);
} catch (Exception e) {
logger.error("Could not get back data of the 'listJNDIENtries' method of the objectName" + objectName, e);
}
List jndiNames = new ArrayList();
List jndiValues = new ArrayList();
List jndiTypes = new ArrayList();
if (compositeDatas != null) {
for (CompositeData compositeData: compositeDatas) {
jndiNames.add(String.class.cast(compositeData.get("name")));
jndiValues.add(String.class.cast(compositeData.get("value")));
jndiTypes.add(String.class.cast(compositeData.get("type")));
}
}
registryProtocol.setJndiNames(jndiNames);
registryProtocol.setJndiValues(jndiValues);
}
}
return list;
}
/**
*
* @return a connection to a MBean Server
* @throws Exception
*/
private MBeanServerConnection getMBeanServerConnection() throws Exception {
MBeanServer mBeanServer = MBeanServer.class.cast(MBeanServerFactory.findMBeanServer(null).get(0));
return mBeanServer;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy