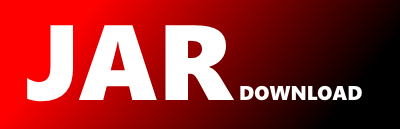
org.ow2.easywsdl.extensions.multiple.impl.MultipleExtReaderImpl Maven / Gradle / Ivy
/**
* Copyright (c) 2008-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the New BSD License (3-clause license).
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the New BSD License (3-clause license)
* for more details.
*
* You should have received a copy of the New BSD License (3-clause license)
* along with this program/library; If not, see http://directory.fsf.org/wiki/License:BSD_3Clause/
* for the New BSD License (3-clause license).
*/
package org.ow2.easywsdl.extensions.multiple.impl;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URISyntaxException;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerException;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import org.ow2.easywsdl.extensions.multiple.MultipleExtFactory;
import org.ow2.easywsdl.extensions.multiple.api.Description;
import org.ow2.easywsdl.extensions.multiple.api.MultipleExtException;
import org.ow2.easywsdl.extensions.multiple.api.MultipleExtReader;
import org.ow2.easywsdl.wsdl.ExtensionFactory;
import org.ow2.easywsdl.wsdl.WSDLFactory;
import org.ow2.easywsdl.wsdl.api.WSDLException;
import org.ow2.easywsdl.wsdl.api.WSDLReader;
import org.ow2.easywsdl.wsdl.api.WSDLReader.FeatureConstants;
import org.ow2.easywsdl.wsdl.api.abstractElmt.AbstractWSDLReaderImpl;
import org.w3c.dom.Document;
import org.xml.sax.InputSource;
import com.ebmwebsourcing.easycommons.xml.Transformers;
/**
* @author Nicolas Boissel-Dallier - EBM WebSourcing
*/
public class MultipleExtReaderImpl implements MultipleExtReader {
private WSDLReader reader = null;
public MultipleExtReaderImpl() throws MultipleExtException {
try {
WSDLFactory factory = WSDLFactory.newInstance();
this.reader = factory.newWSDLReader();
} catch (final WSDLException e) {
throw new MultipleExtException(e);
}
}
public Object getFeature(final FeatureConstants name) {
return this.reader.getFeature(name);
}
public Map getFeatures() {
return this.reader.getFeatures();
}
public void setFeatures(final Map features) {
((AbstractWSDLReaderImpl) this.reader).setFeatures(features);
}
/**
* {@inheritDoc}
*
*/
public Description read(final URL wsdlURL) throws MultipleExtException, IOException, URISyntaxException {
List emptyList = new ArrayList();
return read(emptyList, wsdlURL);
}
/**
* {@inheritDoc}
*
*/
public Description read(final List extFactories, final URL wsdlURL) throws MultipleExtException, IOException, URISyntaxException {
try {
InputSource inputSource = new InputSource(wsdlURL.openStream());
inputSource.setSystemId(wsdlURL.toString());
return this.read(extFactories, inputSource);
} catch (final MalformedURLException e) {
throw new RuntimeException("The provided well-formed URL has been detected as malformed !!");
} catch (final WSDLException e) {
throw new MultipleExtException(e);
}
}
/**
* {@inheritDoc}
*
*/
public Description read(final List extFactories, final Document wsdlDocument) throws MultipleExtException, URISyntaxException {
// The DOM Document needs to be converted into an InputStource
final ByteArrayOutputStream baos = new ByteArrayOutputStream();
final StreamResult streamResult = new StreamResult(baos);
// FIXME: The Transformer creation is not thread-safe
Transformer transformer = null;
try {
transformer = Transformers.takeTransformer();
transformer.transform(new DOMSource(wsdlDocument), streamResult);
baos.flush();
baos.close();
final InputSource documentInputSource = new InputSource(new ByteArrayInputStream(
baos.toByteArray()));
documentInputSource.setSystemId(wsdlDocument.getBaseURI());
return this.read(extFactories, documentInputSource);
} catch (final TransformerException e) {
throw new MultipleExtException(e);
} catch (final IOException e) {
throw new MultipleExtException(e);
} finally {
Transformers.releaseTransformer(transformer);
}
}
/**
* {@inheritDoc}
*
*/
public Description read(final List extFactories, final InputSource wsdlInputSource) throws MultipleExtException, MalformedURLException, URISyntaxException {
try {
final org.ow2.easywsdl.wsdl.api.Description desc = this.reader.read(wsdlInputSource);
return MultipleExtFactory.newInstance().addMultipleExtElmt2Description(extFactories, desc);
} catch (final WSDLException e) {
throw new MultipleExtException(e);
}
}
public void setFeature(final FeatureConstants name, final Object value) throws WSDLException {
this.reader.setFeature(name, value);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy