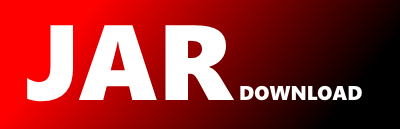
org.objectweb.fractal.koch.Koch Maven / Gradle / Ivy
The newest version!
/***
* Julia
* Copyright (C) 2005-2006 INRIA, France Telecom, USTL
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Contact: [email protected]
*
* Author: Lionel Seinturier
*/
package org.objectweb.fractal.koch;
import java.util.Map;
import org.objectweb.fractal.api.Component;
import org.objectweb.fractal.api.NoSuchInterfaceException;
import org.objectweb.fractal.api.Type;
import org.objectweb.fractal.api.control.BindingController;
import org.objectweb.fractal.api.factory.Factory;
import org.objectweb.fractal.api.factory.GenericFactory;
import org.objectweb.fractal.api.factory.InstantiationException;
import org.objectweb.fractal.api.type.ComponentType;
import org.objectweb.fractal.api.type.InterfaceType;
import org.objectweb.fractal.julia.factory.ChainedInstantiationException;
import org.objectweb.fractal.julia.loader.Loader;
import org.objectweb.fractal.julia.type.BasicComponentType;
import org.objectweb.fractal.koch.control.membrane.MembraneController;
import org.objectweb.fractal.koch.factory.BasicGenericFactoryImpl;
import org.objectweb.fractal.koch.factory.MembraneFactoryImpl;
import org.objectweb.fractal.koch.factory.TypeFactoryImpl;
import org.objectweb.fractal.koch.loader.KochDynamicLoader;
import org.objectweb.fractal.koch.loader.KochDynamicLoaderAttributes;
/**
* The Fractal provider class for managing component-based control membranes.
* This class provides the {@link #newFcInstance()} method for retrieving an
* instance of the Koch bootstrap component.
*
* @author Lionel Seinturier
* @since 2.5
*/
public class Koch implements Factory, GenericFactory {
/** The name of the default Koch configuration file. */
final public static String DEFAULT_CONFIGURATION = "koch-bundled.cfg";
public Koch() {}
/** Return the Fractal type of this component. */
public Type getFcInstanceType() {
return TYPE;
}
/** Return the Fractal controller description of this component. */
public Object getFcControllerDesc() {
return CONTROLLER_DESC;
}
/** Return the Fractal content description of this component. */
public Object getFcContentDesc() {
return null;
}
/**
* Return the reference of the bootstrap component.
*/
public Component newFcInstance() throws InstantiationException {
return newFcInstance(null,null,null);
}
/**
* Return the reference of the bootstrap component.
*/
public Component newFcInstance(
Type type, Object controllerDesc, Object contentDesc )
throws InstantiationException {
if( bootstrap == null ) {
// Get an object-based version of the generic factory
GenericFactory gf = getFcGenericFactory(contentDesc);
// Create the Juliak bootstrap component
bootstrap =
gf.newFcInstance(
getFcInstanceType(),
getFcControllerDesc(),
getFcContentDesc() );
/*
* The contentDesc argument of this method may contain a
* julia.config property which must be transmitted to the loader
* component. This component is a subcomponent of the bootstrap
* (which is a composite). The loader component provides an
* attribute-controller interface which is exported by the
* bootstrap component. This interface allows initializing the
* loader component.
*/
try {
MembraneController mc = (MembraneController)
bootstrap.getFcInterface("/membrane-controller");
Component membrane = mc.getFcMembrane();
KochDynamicLoaderAttributes attrItf =
(KochDynamicLoaderAttributes)
membrane.getFcInterface("attribute-controller");
setConfigFileName(attrItf,contentDesc);
}
catch (NoSuchInterfaceException e) {
String msg = "NoSuchInterfaceException: "+e.getMessage();
throw new ChainedInstantiationException(e,null,msg);
}
}
return bootstrap;
}
private static ComponentType TYPE = null;
final private static Object CONTROLLER_DESC = "bootstrap";
private static Component bootstrap = null;
static {
try {
TYPE = new BasicComponentType(new InterfaceType[0]);
}
catch (InstantiationException e) {
throw new RuntimeException(e);
}
}
// ----------------------------------------------------------
// Implementation specific
// ----------------------------------------------------------
private static GenericFactory gf;
private static Loader kochLoader;
private GenericFactory getFcGenericFactory( Object hints ) throws InstantiationException {
if( gf == null ) {
// Juliak generic factory
BasicGenericFactoryImpl genericFactory = getGenericFactory();
TypeFactoryImpl typeFactory = new TypeFactoryImpl();
MembraneFactoryImpl membraneFactory = new MembraneFactoryImpl();
try {
BindingController bootstrapMembrane = (BindingController)
getFcKochLoader(hints).loadClass(getBootstrapMembrane(), null).
newInstance();
genericFactory.bindFc("loader", getFcKochLoader(hints));
genericFactory.bindFc("membrane-factory", membraneFactory);
typeFactory.bindFc("loader", getFcKochLoader(hints));
bootstrapMembrane.bindFc("type-factory", typeFactory);
bootstrapMembrane.bindFc("generic-factory", genericFactory);
membraneFactory.bindFc("membranes-bootstrap", bootstrapMembrane);
membraneFactory.bindFc("generic-factory", genericFactory);
membraneFactory.bindFc("type-factory", typeFactory);
membraneFactory.bindFc("loader", getFcKochLoader(hints));
}
catch( Exception e ) {
String msg = e.getClass()+": "+e.getMessage();
throw new ChainedInstantiationException(e,null,msg);
}
gf = genericFactory;
}
return gf;
}
/**
* @return the concrete generic factory.
*/
protected BasicGenericFactoryImpl getGenericFactory() {
BasicGenericFactoryImpl genericFactory = new BasicGenericFactoryImpl();
return genericFactory;
}
/**
* Return the fully-qualified name of the Bootstrap component.
*/
protected String getBootstrapMembrane() {
return "org.objectweb.fractal.koch.membrane.Bootstrap";
}
private Loader getFcKochLoader( Object hints ) {
if( kochLoader == null ) {
KochDynamicLoader loader = new KochDynamicLoader();
loader.setConfigFileName(DEFAULT_CONFIGURATION);
setConfigFileName(loader, hints);
kochLoader = loader;
}
return kochLoader;
}
private void setConfigFileName(
KochDynamicLoaderAttributes loaderAttrItf, Object hints ) {
String configFileName = null;
if( hints instanceof Map ) {
configFileName = (String) ((Map)hints).get("julia.config");
}
else if( System.getProperty("julia.config") != null ) {
configFileName = System.getProperty("julia.config");
}
if( configFileName != null ) {
loaderAttrItf.setConfigFileName(configFileName);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy