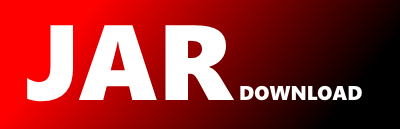
org.objectweb.fractal.koch.factory.ControlComponentBoundMap Maven / Gradle / Ivy
The newest version!
/***
* Julia
* Copyright (C) 2005-2006 INRIA, France Telecom, USTL
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Contact: [email protected]
*
* Author: Lionel Seinturier
*/
package org.objectweb.fractal.koch.factory;
import org.objectweb.fractal.api.Component;
import org.objectweb.fractal.api.NoSuchInterfaceException;
/**
* An implementation of a {@link java.util.Map} where the get method returns the
* interface associated to a control component.
*
* @author Lionel Seinturier
* @since 2.5
*/
public class ControlComponentBoundMap extends AbstractMap {
/** The component controller. */
protected Component ctrlcomp;
public ControlComponentBoundMap( Component ctrlcomp ) {
this.ctrlcomp = ctrlcomp;
}
/**
* Return the interface associated to the current control component.
*
* This method is typically used by controllers which request the control
* interface exported by one their peers.
*
* @param key the interface name
*/
public Object get(Object key) {
if( !(key instanceof String) ) {
final String msg = "Key "+key+" should be a String";
throw new IllegalArgumentException(msg);
}
/*
* Twisting interface names between //foo and foo.
*
* Control components are regular Fractal component in the sense that
* they can be managed with the Fractal API. A control component
* provides, as any other Fractal component, a component interface, a
* name-controller interface, a binding-controller, etc.
*
* However, a control component also implements a control function (for
* example, that of a binding controller) for a base level component.
* There is then a name clash between the control interface names
* corresponding to the component personality of the controller and the
* control service provided to a base level component. To solve this
* clash, the interface names of these last services are prefixed with
* two slash characters. Hence, a control component which requires the
* services by the binding controller should request the
* //binding-controller interface.
*
* However, the existing Julia code does not do that and simply
* retrieves the binding-controller interface (the clash is meaningless
* with Julia as the notion of a control component does not exist).
* Hence to preserve a ascending compatibility, when a controller
* requests interface foo, we append the // prefix to provide a
* consistent behavior with the architecture of the membrane.
*
* However, by doing so, we prevent a controller from accessing its
* control interfaces (component, name-controller, etc.). To solve the
* issue, we adopt the convention, that, if the requested interface
* name starts with //, we consider that the request concerns a control
* interface (of the control component), and we remove the // prefix.
*
* Hence the twisting between //foo and foo.
*/
String itfName = (String) key;
if( itfName.startsWith("//") ) {
itfName = itfName.substring(2);
}
else {
itfName = "//" + itfName;
}
try {
return ctrlcomp.getFcInterface(itfName);
}
catch( NoSuchInterfaceException nsie ) {
return null;
}
}
/**
* Return true if the current control component defines the given interface.
*
* This method is typically used by controllers which request the control
* interface exported by one their peers.
*
* @param key the interface name
* @return true if the interface exists
*/
public boolean containsKey(Object key) {
if( !(key instanceof String) ) {
final String msg = "Key "+key+" should be a String";
throw new IllegalArgumentException(msg);
}
/*
* When the controller requests interface foo, its component personality
* defines this interface as //foo.
*/
String itfName = "//" + key;
try {
ctrlcomp.getFcInterface(itfName);
return true;
}
catch( NoSuchInterfaceException nsie ) {
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy