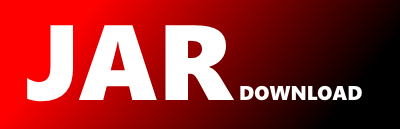
org.objectweb.fractal.koch.loader.TreeParser Maven / Gradle / Ivy
The newest version!
/***
* Julia
* Copyright (C) 2005-2006 INRIA, France Telecom, USTL
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Contact: [email protected]
*
* Author: Lionel Seinturier
*/
package org.objectweb.fractal.koch.loader;
import java.util.ArrayList;
import java.util.List;
import org.objectweb.fractal.julia.loader.Tree;
/**
* A class for parsing string representations of {@link Tree} structures.
* These string representations follow a LISP like syntax such as
* "(foo (bar foo))".
*
* @author Lionel Seinturier
* @since 2.5
*/
public class TreeParser {
/** The string to parse. */
private String str;
public TreeParser( String str ) {
this.str = str;
read();
}
/**
* Copy/paste from
* {@link org.objectweb.fractal.julia.loader.BasicLoader#parseTree()}.
*
* Recursive method to parse a tree. The first character of the tree to be
* parsed is supposed to have already been read, and available in {@link #car
* car}. After parsing, the character immediately following the parsed tree
* is also supposed to have already been parsed, and available in {@link #car
* car}.
*
* @return a tree parsed from {@link #str}.
* @throws TreeParserException if a syntax error is found.
*/
public Tree parseTree() throws TreeParserException {
int c = car;
if (c == -1) {
throw new TreeParserException("Unexpected end of string");
} else if (c == ')') {
throw new TreeParserException("Unmatched closing parenthesis");
} else if (c == '(') {
// parses a tree of the form "(subTree1 ... subTreeN)"
read();
List subTrees = new ArrayList();
while (true) {
c = parseSpaces();
if (c == ')') {
read();
return new Tree((Tree[])subTrees.toArray(new Tree[subTrees.size()]));
} else {
subTrees.add(parseTree());
}
}
} else {
// parses a tree of the form "tree"
StringBuffer buf = new StringBuffer();
while (true) {
buf.append((char)c);
c = read();
if (c == -1 || c == ' ' || c == '\t' || c == '\n' ||
c == '\r' || c == '#' || c == '(' || c == ')')
{
car = c;
return new Tree(buf.toString());
}
}
}
}
/**
* Copy/paste from
* {@link org.objectweb.fractal.julia.loader.BasicLoader#parseTree()}.
*
* Parses spaces and comments until a non space character is found.
*
* @return the first non space character found, which is also stored in
* {@link #car car}.
*/
private int parseSpaces() {
int c = car;
while (c == ' ' || c == '\t' || c == '\n' || c == '\r' || c == '#') {
if (c == '#') {
// parses a single line comment
do {
c = read();
} while (c != '\n' && c != '\r');
}
c = read();
}
return car = c;
}
private int read() {
car = (idx>=str.length()) ? -1 : str.charAt(idx);
idx++;
return car;
}
/** Last character read from {@link #str}. */
private int car;
/** Index of the next character to be read from {@link #str}. */
private int idx = 0;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy