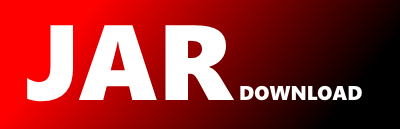
juliac.ComponentImpl Maven / Gradle / Ivy
The newest version!
package juliac;
/**
*
*
* @see org.objectweb.fractal.julia.BasicControllerMixin
* @see org.objectweb.fractal.julia.BasicComponentMixin
* @see org.objectweb.fractal.julia.TypeComponentMixin
* @see org.objectweb.fractal.koch.control.interceptor.CollectionItfComponentMixin
*/
public class ComponentImpl implements org.objectweb.fractal.julia.loader.Generated , org.objectweb.fractal.julia.Controller , org.objectweb.fractal.api.Component {
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* Does nothing.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
* @see org.objectweb.fractal.julia.BasicControllerMixin#initFcController(org.objectweb.fractal.julia.InitializationContext)
*/
private void initFcController$0(final org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* The type of this component.
*
* @see org.objectweb.fractal.julia.BasicComponentMixin#fcType
*/
public org.objectweb.fractal.api.Type fcType;
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
*
*
* @see org.objectweb.fractal.koch.control.interceptor.CollectionItfComponentMixin#getFcInterface(java.lang.String)
*/
public java.lang.Object getFcInterface(final java.lang.String interfaceName) throws org.objectweb.fractal.api.NoSuchInterfaceException {
if (interfaceName.startsWith("/koch/collection/")) {
return fcInterfaces.get(interfaceName);
}
return getFcInterface$0(interfaceName);
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* Checks the interface name against the component's type and then calls the
* overriden method. This method also creates the collection interfaces
* when needed, and puts them in the {@link #_this_fcInterfaces} map.
*
* @param interfaceName
* the name of the external interface that must be
* returned.
* @return the external interface of the component to which this interface
belongs, whose name is equal to the given name.
* @throws NoSuchInterfaceException
* if there is no such interface.
* @see org.objectweb.fractal.julia.TypeComponentMixin#getFcInterface(java.lang.String)
*/
private java.lang.Object getFcInterface$0(final java.lang.String interfaceName) throws org.objectweb.fractal.api.NoSuchInterfaceException {
if (interfaceName.indexOf('/') == 0) {
return getFcInterface$1(interfaceName);
}
org.objectweb.fractal.api.type.ComponentType compType = ((org.objectweb.fractal.api.type.ComponentType) (getFcType()));
org.objectweb.fractal.api.type.InterfaceType itfType;
try {
itfType = compType.getFcInterfaceType(interfaceName);
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
throw new org.objectweb.fractal.julia.ChainedNoSuchInterfaceException(null, this, interfaceName);
}
java.lang.Object result;
try {
result = getFcInterface$1(interfaceName);
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
if (itfType.isFcCollectionItf()) {
result = getFcInterface$1("/collection/" + itfType.getFcItfName());
result = ((org.objectweb.fractal.julia.ComponentInterface) (result)).clone();
((org.objectweb.fractal.julia.ComponentInterface) (result)).setFcItfName(interfaceName);
fcInterfaces.put(interfaceName, result);
} else {
throw e;
}
}
return result;
}
/**
* The interfaces of this component. This map associates each interface to its
* name.
*
* @see org.objectweb.fractal.julia.BasicComponentMixin#fcInterfaces
*/
public java.util.Map fcInterfaces;
/**
* Initializes the fields of this mixin from the given context, and then calls
* the overriden method.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
* @see org.objectweb.fractal.julia.BasicComponentMixin#initFcController(org.objectweb.fractal.julia.InitializationContext)
*/
public void initFcController(final org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
this.fcType = ic.type;
this.fcInterfaces = ic.interfaces;
initFcController$0(ic);
}
/**
*
*
* @see org.objectweb.fractal.julia.BasicComponentMixin#getFcType()
*/
public org.objectweb.fractal.api.Type getFcType() {
return fcType;
}
/**
*
*
* @see org.objectweb.fractal.julia.BasicComponentMixin#getFcInterfaces()
*/
public java.lang.Object[] getFcInterfaces() {
if (fcInterfaces == null) {
return new java.lang.Object[0];
}
// returns the names of all the public interfaces in fcInterfaces
// interfaces whose name begins with '/' are private
int size = 0;
java.lang.String[] names = new java.lang.String[fcInterfaces.size()];
names = ((java.lang.String[]) (fcInterfaces.keySet().toArray(names)));
for (int i = 0; i < names.length; ++i) {
if (names[i].charAt(0) != '/') {
++size;
}
}
int index = 0;
java.lang.Object[] itfs = new java.lang.Object[size];
for (int i = 0; i < names.length; ++i) {
if (names[i].charAt(0) != '/') {
itfs[index++] = fcInterfaces.get(names[i]);
}
}
return itfs;
}
/**
*
*
* @see org.objectweb.fractal.julia.BasicComponentMixin#getFcInterface(java.lang.String)
*/
private java.lang.Object getFcInterface$1(final java.lang.String interfaceName) throws org.objectweb.fractal.api.NoSuchInterfaceException {
java.lang.Object itf = (fcInterfaces == null) ? null : fcInterfaces.get(interfaceName);
if (itf == null) {
throw new org.objectweb.fractal.julia.ChainedNoSuchInterfaceException(null, this, interfaceName);
}
return itf;
}
// -------------------------------------------------------------------------
// Fields and methods required by the mixin class in the base class
// -------------------------------------------------------------------------
/**
* The {@link Controller#initFcController initFcController} method overriden
* by this mixin.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
*/
private void initFcController$1(org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
}
/**
* The {@link Component#getFcInterface getFcInterface} method overriden by
* this mixin.
*
* @param interfaceName
* the name of the external interface that must be
* returned.
* @return the external interface of the component to which this interface
belongs, whose name is equal to the given name.
* @throws NoSuchInterfaceException
* if there is no such interface.
*/
private java.lang.Object getFcInterface$2(java.lang.String interfaceName) throws org.objectweb.fractal.api.NoSuchInterfaceException {
return null;
}
public java.lang.String getFcGeneratorParameters() {
return "(org.objectweb.fractal.juliac.spoon.MixinClassGenerator juliac.ComponentImpl org.objectweb.fractal.julia.BasicControllerMixin org.objectweb.fractal.julia.BasicComponentMixin org.objectweb.fractal.julia.TypeComponentMixin org.objectweb.fractal.koch.control.interceptor.CollectionItfComponentMixin)";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy