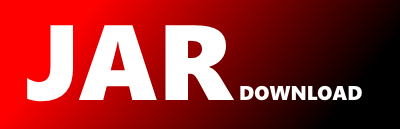
juliac.CompositeLifeCycleControllerImpl Maven / Gradle / Ivy
The newest version!
package juliac;
/**
*
*
* @see org.objectweb.fractal.julia.BasicControllerMixin
* @see org.objectweb.fractal.julia.UseComponentMixin
* @see org.objectweb.fractal.julia.control.lifecycle.BasicLifeCycleCoordinatorMixin
* @see org.objectweb.fractal.julia.control.lifecycle.OptimizedLifeCycleControllerMixin
* @see org.objectweb.fractal.julia.control.lifecycle.TypeLifeCycleMixin
*/
public class CompositeLifeCycleControllerImpl implements org.objectweb.fractal.julia.loader.Generated , org.objectweb.fractal.julia.Controller , org.objectweb.fractal.api.control.LifeCycleController , org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator {
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* Does nothing.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
* @see org.objectweb.fractal.julia.BasicControllerMixin#initFcController(org.objectweb.fractal.julia.InitializationContext)
*/
private void initFcController$0(final org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* The {@link Component} interface of the component to which this controller
* object belongs.
*
* @see org.objectweb.fractal.julia.UseComponentMixin#weaveableC
*/
public org.objectweb.fractal.api.Component weaveableC;
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* The components that are currently active.
*
* @see org.objectweb.fractal.julia.control.lifecycle.BasicLifeCycleCoordinatorMixin#fcActive
*/
public java.util.List fcActive;
/**
* The {@link Component} interface of the component to which this controller
* object belongs.
*
* @see org.objectweb.fractal.julia.UseComponentMixin#weaveableOptC
*/
public org.objectweb.fractal.api.Component weaveableOptC;
/**
* Initializes the fields of this mixin and then calls the overriden method.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
* @see org.objectweb.fractal.julia.UseComponentMixin#initFcController(org.objectweb.fractal.julia.InitializationContext)
*/
public void initFcController(final org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
weaveableC = ((org.objectweb.fractal.api.Component) (ic.getInterface("component")));
weaveableOptC = weaveableC;
initFcController$0(ic);
}
/**
* Sets the lifecycle state of this component and of all its direct and
* indirect sub components that have a {@link LifeCycleCoordinator} interface.
*
* @param started
* true to set the lifecycle state of the components
* to {@link #STARTED STARTED}, or false to set this state to
* {@link #STOPPED STOPPED}.
* @throws IllegalLifeCycleException
* if a problem occurs.
* @see org.objectweb.fractal.julia.control.lifecycle.BasicLifeCycleCoordinatorMixin#setFcState(boolean)
*/
public void setFcState(final boolean started) throws org.objectweb.fractal.api.control.IllegalLifeCycleException {
// finds all the direct and indirect sub components of this component
org.objectweb.fractal.api.Component thisComponent;
try {
thisComponent = ((org.objectweb.fractal.api.Component) (weaveableC.getFcInterface("component")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
throw new org.objectweb.fractal.julia.control.lifecycle.ChainedIllegalLifeCycleException(e, weaveableC, "Cannot set the lifecycle state");
}
java.util.List allSubComponents = org.objectweb.fractal.julia.control.content.Util.getAllSubComponents(thisComponent);
// sets the state of these components
for (int i = 0; i < allSubComponents.size(); ++i) {
org.objectweb.fractal.api.Component c = ((org.objectweb.fractal.api.Component) (allSubComponents.get(i)));
org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator lc;
try {
lc = ((org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator) (c.getFcInterface("lifecycle-controller")));
} catch (java.lang.Exception e) {
try {
lc = ((org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator) (c.getFcInterface("/lifecycle-coordinator")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException f) {
continue;
}
}
if (started) {
lc.setFcStarted();
} else {
lc.setFcStopped();
}
}
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* Checks the mandatory client interfaces of the component (and of all its sub
* components) and then calls the overriden method.
*
* @throws IllegalLifeCycleException
* if a problem occurs.
* @see org.objectweb.fractal.julia.control.lifecycle.TypeLifeCycleMixin#startFc()
*/
public void startFc() throws org.objectweb.fractal.api.control.IllegalLifeCycleException {
// finds all the direct and indirect sub components of this component
org.objectweb.fractal.api.Component thisComponent;
try {
thisComponent = ((org.objectweb.fractal.api.Component) (weaveableC.getFcInterface("component")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
throw new org.objectweb.fractal.julia.control.lifecycle.ChainedIllegalLifeCycleException(e, weaveableC, "Cannot start the component");
}
java.util.List allSubComponents = org.objectweb.fractal.julia.control.content.Util.getAllSubComponents(thisComponent);
// checks that the mandatory client interfaces of these components are bound
for (int i = 0; i < allSubComponents.size(); ++i) {
org.objectweb.fractal.api.Component subComponent = ((org.objectweb.fractal.api.Component) (allSubComponents.get(i)));
try {
checkFcMandatoryInterfaces(subComponent);
} catch (org.objectweb.fractal.api.control.IllegalBindingException e) {
throw new org.objectweb.fractal.julia.control.lifecycle.ChainedIllegalLifeCycleException(e, weaveableC, "Cannot start the component");
}
}
// calls the overriden method
startFc$0();
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* Indicates if this component is started or not.
*
* @see org.objectweb.fractal.julia.control.lifecycle.OptimizedLifeCycleControllerMixin#fcStarted
*/
public boolean fcStarted;
// -------------------------------------------------------------------------
// Fields and methods required by the mixin class in the base class
// -------------------------------------------------------------------------
/**
* The {@link Controller#initFcController initFcController} method overriden
* by this mixin.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
*/
private void initFcController$1(org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
}
// -------------------------------------------------------------------------
// Implementation of the LifeCycleController interface
// -------------------------------------------------------------------------
/**
*
*
* @see org.objectweb.fractal.julia.control.lifecycle.OptimizedLifeCycleControllerMixin#getFcState()
*/
public java.lang.String getFcState() {
return fcStarted ? org.objectweb.fractal.api.control.LifeCycleController.STARTED : org.objectweb.fractal.api.control.LifeCycleController.STOPPED;
}
/**
*
*
* @see org.objectweb.fractal.julia.control.lifecycle.OptimizedLifeCycleControllerMixin#startFc()
*/
private void startFc$0() throws org.objectweb.fractal.api.control.IllegalLifeCycleException {
org.objectweb.fractal.api.Component id;
try {
id = ((org.objectweb.fractal.api.Component) (weaveableC.getFcInterface("component")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
throw new org.objectweb.fractal.julia.control.lifecycle.ChainedIllegalLifeCycleException(e, weaveableC, "Cannot start component");
}
org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator[] clccs = getFcLifeCycleControllers(id);
for (int i = 0; i < clccs.length; ++i) {
clccs[i].setFcStarted();
}
setFcState(true);
}
/**
*
*
* @see org.objectweb.fractal.julia.control.lifecycle.OptimizedLifeCycleControllerMixin#stopFc()
*/
public void stopFc() throws org.objectweb.fractal.api.control.IllegalLifeCycleException {
org.objectweb.fractal.api.Component id;
try {
id = ((org.objectweb.fractal.api.Component) (weaveableC.getFcInterface("component")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
throw new org.objectweb.fractal.julia.control.lifecycle.ChainedIllegalLifeCycleException(e, weaveableC, "Cannot stop component");
}
org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator[] clccs = getFcLifeCycleControllers(id);
stopFc(clccs);
setFcState(false);
}
/**
* Checks that all the mandatory client interface of the given component are
* bound.
*
* @param c
* a component.
* @throws IllegalBindingException
* if a mandatory client interface of the
* given component is not bound.
* @see org.objectweb.fractal.julia.control.lifecycle.TypeLifeCycleMixin#checkFcMandatoryInterfaces(org.objectweb.fractal.api.Component)
*/
public void checkFcMandatoryInterfaces(final org.objectweb.fractal.api.Component c) throws org.objectweb.fractal.api.control.IllegalBindingException {
org.objectweb.fractal.api.control.BindingController bc;
try {
bc = ((org.objectweb.fractal.api.control.BindingController) (c.getFcInterface("binding-controller")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
return;
}
org.objectweb.fractal.api.type.ComponentType compType = ((org.objectweb.fractal.api.type.ComponentType) (c.getFcType()));
java.lang.String[] names = bc.listFc();
for (int i = 0; i < names.length; ++i) {
org.objectweb.fractal.api.type.InterfaceType itfType;
try {
itfType = compType.getFcInterfaceType(names[i]);
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
continue;
}
if (itfType.isFcClientItf() && (!itfType.isFcOptionalItf())) {
java.lang.Object sItf;
try {
sItf = bc.lookupFc(names[i]);
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
continue;
}
if (sItf == null) {
throw new org.objectweb.fractal.julia.control.binding.ChainedIllegalBindingException(null, c, null, names[i], null, "Mandatory client interface unbound");
}
}
}
}
/**
*
*
* @see org.objectweb.fractal.julia.control.lifecycle.BasicLifeCycleCoordinatorMixin#fcActivated(org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator)
*/
public boolean fcActivated(final org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator component) {
synchronized(fcActive) {
// a component can become active iff another component is already active
if (fcActive.size() > 0) {
if (!fcActive.contains(component)) {
fcActive.add(component);
}
return true;
}
return false;
}
}
// -------------------------------------------------------------------------
// Implementation of the CoordinatorLifeCycleController interface
// -------------------------------------------------------------------------
/**
*
*
* @see org.objectweb.fractal.julia.control.lifecycle.OptimizedLifeCycleControllerMixin#setFcStarted()
*/
public boolean setFcStarted() {
if (!fcStarted) {
fcStarted = true;
return true;
}
return false;
}
/**
*
*
* @see org.objectweb.fractal.julia.control.lifecycle.BasicLifeCycleCoordinatorMixin#fcInactivated(org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator)
*/
public void fcInactivated(final org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator component) {
synchronized(fcActive) {
fcActive.remove(component);
// notifies the thread that may be blocked in stopFc
fcActive.notifyAll();
}
}
/**
* Stops the given components simultaneously. This method sets the state of
* the components to "STOPPING", waits until all the components
* are simultaneoulsy inactive (their state is known thanks to the {@link #fcActivated fcActivated} and {@link #fcInactivated fcInactivated} callback
* methods), and then sets the state of the components to {@link #STOPPED
* STOPPED}.
*
* @param components
* the {@link LifeCycleCoordinator} interface of the
* components to be stopped.
* @throws IllegalLifeCycleException
* if a problem occurs.
* @see org.objectweb.fractal.julia.control.lifecycle.BasicLifeCycleCoordinatorMixin#stopFc(org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator[])
*/
public void stopFc(final org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator[] components) throws org.objectweb.fractal.api.control.IllegalLifeCycleException {
// initializes the fcActive list
fcActive = new java.util.ArrayList();
for (int i = 0; i < components.length; ++i) {
if (components[i].getFcState().equals(org.objectweb.fractal.api.control.LifeCycleController.STARTED)) {
fcActive.add(components[i]);
}
}
// sets the state of the components to STOPPING
org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator c;
try {
c = ((org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator) (weaveableC.getFcInterface("lifecycle-controller")));
} catch (java.lang.Exception e) {
try {
c = ((org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator) (weaveableC.getFcInterface("/lifecycle-coordinator")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException f) {
throw new org.objectweb.fractal.julia.control.lifecycle.ChainedIllegalLifeCycleException(f, weaveableC, "Cannot stop components");
}
}
for (int i = 0; i < components.length; ++i) {
if (components[i].getFcState().equals(org.objectweb.fractal.api.control.LifeCycleController.STARTED)) {
components[i].setFcStopping(c);
}
}
// waits until all the components are simultaneously inactive
synchronized(fcActive) {
while (fcActive.size() > 0) {
try {
fcActive.wait();
} catch (java.lang.InterruptedException e) {
}
}
}
// sets the state of the components to STOPPED
for (int i = 0; i < components.length; ++i) {
if (components[i].getFcState().equals(org.objectweb.fractal.api.control.LifeCycleController.STARTED)) {
components[i].setFcStopped();
}
}
fcActive = null;
}
/**
*
*
* @see org.objectweb.fractal.julia.control.lifecycle.OptimizedLifeCycleControllerMixin#setFcStopping(org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator)
*/
public void setFcStopping(final org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator coordinator) throws org.objectweb.fractal.api.control.IllegalLifeCycleException {
// this method should never be called,
// as this component is stopped by stopping its sub components
throw new java.lang.Error("Internal error");
}
/**
*
*
* @see org.objectweb.fractal.julia.control.lifecycle.OptimizedLifeCycleControllerMixin#setFcStopped()
*/
public boolean setFcStopped() {
if (fcStarted) {
fcStarted = false;
return true;
}
return false;
}
// -------------------------------------------------------------------------
// Utility methods
// -------------------------------------------------------------------------
/**
* Returns the components that must be stopped in order to stop the given
* component. These components are the direct or indirect primitive sub
* components of this component that provide a {@link LifeCycleCoordinator}
* interface, as well as the primitive client components of these components.
*
* @param id
* a composite component.
* @return the components that must be stopped in order to stop the given
component.
* @throws IllegalLifeCycleException
* if a problem occurs.
* @see org.objectweb.fractal.julia.control.lifecycle.OptimizedLifeCycleControllerMixin#getFcLifeCycleControllers(org.objectweb.fractal.api.Component)
*/
public org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator[] getFcLifeCycleControllers(final org.objectweb.fractal.api.Component id) throws org.objectweb.fractal.api.control.IllegalLifeCycleException {
java.util.List clccList = getFcInternalLifeCycleControllers();
java.lang.Object[] sItfs = id.getFcInterfaces();
java.util.Set visited = new java.util.HashSet();
for (int i = 0; i < sItfs.length; ++i) {
org.objectweb.fractal.api.Interface sItf = ((org.objectweb.fractal.api.Interface) (sItfs[i]));
if (!((org.objectweb.fractal.api.type.InterfaceType) (sItf.getFcItfType())).isFcClientItf()) {
getSExtLifeCycleControllers(sItf, clccList, visited);
}
}
org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator[] clccs;
clccs = new org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator[clccList.size()];
return ((org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator[]) (clccList.toArray(clccs)));
}
/**
* The {@link LifeCycleController#startFc startFc} method overriden by this
* mixin.
*
* @throws IllegalLifeCycleException
* if a problem occurs.
*/
private void startFc$1() throws org.objectweb.fractal.api.control.IllegalLifeCycleException {
}
/**
* Finds the primitive client components that are bound to the given server
* interface.
*
* @param serverItf
* a server interface.
* @param clccList
* where to put the {@link LifeCycleCoordinator}
* interfaces of the primitive client components that are found.
* @param visited
* the already visited interfaces.
* @throws IllegalLifeCycleException
* if a problem occurs.
* @see org.objectweb.fractal.julia.control.lifecycle.OptimizedLifeCycleControllerMixin#getSExtLifeCycleControllers(org.objectweb.fractal.api.Interface,java.util.List,java.util.Set)
*/
private void getSExtLifeCycleControllers(final org.objectweb.fractal.api.Interface serverItf, final java.util.List clccList, final java.util.Set visited) throws org.objectweb.fractal.api.control.IllegalLifeCycleException {
java.lang.Object[] comps;
try {
comps = org.objectweb.fractal.julia.control.binding.Util.getFcPotentialClientsOf(serverItf).toArray();
} catch (java.lang.Exception e) {
throw new org.objectweb.fractal.julia.control.lifecycle.ChainedIllegalLifeCycleException(e, serverItf.getFcItfOwner(), "Cannot get the LifeCycleCoordinator interfaces");
}
for (int i = 0; i < comps.length; ++i) {
org.objectweb.fractal.api.Component comp = ((org.objectweb.fractal.api.Component) (comps[i]));
org.objectweb.fractal.api.Interface[] clientItfs;
try {
java.util.List l = org.objectweb.fractal.julia.control.binding.Util.getFcClientItfsBoundTo(comp, serverItf);
clientItfs = ((org.objectweb.fractal.api.Interface[]) (l.toArray(new org.objectweb.fractal.api.Interface[l.size()])));
} catch (java.lang.Exception e) {
throw new org.objectweb.fractal.julia.control.lifecycle.ChainedIllegalLifeCycleException(e, serverItf.getFcItfOwner(), "Cannot get the LifeCycleCoordinator interfaces");
}
for (int j = 0; j < clientItfs.length; ++j) {
getCExtLifeCycleControllers(clientItfs[j], clccList, visited);
}
}
}
/**
* Finds the primitive client components that are bound to the given client
* interface.
*
* @param clientItf
* a client interface.
* @param clccList
* where to put the {@link LifeCycleCoordinator} interfaces of
* the primitive client components that are found.
* @param visited
* the already visited interfaces.
* @throws IllegalLifeCycleException
* if a problem occurs.
* @see org.objectweb.fractal.julia.control.lifecycle.OptimizedLifeCycleControllerMixin#getCExtLifeCycleControllers(org.objectweb.fractal.api.Interface,java.util.List,java.util.Set)
*/
private void getCExtLifeCycleControllers(final org.objectweb.fractal.api.Interface clientItf, final java.util.List clccList, final java.util.Set visited) throws org.objectweb.fractal.api.control.IllegalLifeCycleException {
org.objectweb.fractal.api.Component component = clientItf.getFcItfOwner();
org.objectweb.fractal.api.control.ContentController cc = null;
try {
cc = ((org.objectweb.fractal.api.control.ContentController) (component.getFcInterface("content-controller")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
}
if (cc != null) {
org.objectweb.fractal.api.Interface itf;
java.lang.String name = clientItf.getFcItfName();
try {
if (!clientItf.isFcInternalItf()) {
itf = ((org.objectweb.fractal.api.Interface) (cc.getFcInternalInterface(name)));
} else {
itf = ((org.objectweb.fractal.api.Interface) (component.getFcInterface(name)));
}
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
throw new org.objectweb.fractal.julia.control.lifecycle.ChainedIllegalLifeCycleException(e, component, "Cannot find the LifeCycleCoordinator interfaces");
}
if (!visited.contains(itf)) {
visited.add(itf);
getSExtLifeCycleControllers(itf, clccList, visited);
}
} else if (!visited.contains(clientItf)) {
visited.add(clientItf);
org.objectweb.fractal.api.Component c = clientItf.getFcItfOwner();
org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator lcc;
try {
lcc = ((org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator) (c.getFcInterface("lifecycle-controller")));
} catch (java.lang.Exception e) {
try {
lcc = ((org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator) (c.getFcInterface("/lifecycle-coordinator")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException f) {
throw new org.objectweb.fractal.julia.control.lifecycle.ChainedIllegalLifeCycleException(f, component, "Primitive client without a LifeCycleCoordinator");
}
}
if (!clccList.contains(lcc)) {
clccList.add(lcc);
}
}
}
/**
*
*
* @see org.objectweb.fractal.julia.control.lifecycle.OptimizedLifeCycleControllerMixin#getFcInternalLifeCycleControllers()
*/
public java.util.List getFcInternalLifeCycleControllers() throws org.objectweb.fractal.api.control.IllegalLifeCycleException {
// finds all the direct and indirect sub components of this component
org.objectweb.fractal.api.Component thisComponent;
try {
thisComponent = ((org.objectweb.fractal.api.Component) (weaveableC.getFcInterface("component")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
throw new org.objectweb.fractal.julia.control.lifecycle.ChainedIllegalLifeCycleException(e, weaveableC, "The OptimizedLifeCycleControllerMixin requires " + "components to provide the Component interface");
}
java.util.List allSubComponents = org.objectweb.fractal.julia.control.content.Util.getAllSubComponents(thisComponent);
java.util.List result = new java.util.ArrayList();
for (int i = 0; i < allSubComponents.size(); ++i) {
org.objectweb.fractal.api.Component c = ((org.objectweb.fractal.api.Component) (allSubComponents.get(i)));
try {
c.getFcInterface("content-controller");
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
try {
// do not remove the cast!
result.add(((org.objectweb.fractal.julia.control.lifecycle.LifeCycleCoordinator) (c.getFcInterface("lifecycle-controller"))));
} catch (java.lang.Exception f) {
try {
result.add(c.getFcInterface("/lifecycle-coordinator"));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException ignored) {
}
}
}
}
return result;
}
public java.lang.String getFcGeneratorParameters() {
return "(org.objectweb.fractal.juliac.spoon.MixinClassGenerator juliac.CompositeLifeCycleControllerImpl org.objectweb.fractal.julia.BasicControllerMixin org.objectweb.fractal.julia.UseComponentMixin org.objectweb.fractal.julia.control.lifecycle.BasicLifeCycleCoordinatorMixin org.objectweb.fractal.julia.control.lifecycle.OptimizedLifeCycleControllerMixin org.objectweb.fractal.julia.control.lifecycle.TypeLifeCycleMixin)";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy