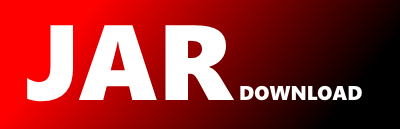
juliac.ContentControllerImpl Maven / Gradle / Ivy
The newest version!
package juliac;
/**
*
*
* @see org.objectweb.fractal.julia.BasicControllerMixin
* @see org.objectweb.fractal.julia.UseComponentMixin
* @see org.objectweb.fractal.julia.control.content.BasicContentControllerMixin
* @see org.objectweb.fractal.julia.control.content.CheckContentMixin
* @see org.objectweb.fractal.julia.control.content.TypeContentMixin
* @see org.objectweb.fractal.koch.control.interceptor.CollectionItfContentControllerMixin
* @see org.objectweb.fractal.julia.control.content.BindingContentMixin
* @see org.objectweb.fractal.julia.control.lifecycle.UseLifeCycleControllerMixin
* @see org.objectweb.fractal.julia.control.content.LifeCycleContentMixin
* @see org.objectweb.fractal.julia.control.content.SuperContentMixin
*/
public class ContentControllerImpl implements org.objectweb.fractal.julia.loader.Generated , org.objectweb.fractal.julia.Controller , org.objectweb.fractal.api.control.ContentController {
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* Does nothing.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
* @see org.objectweb.fractal.julia.BasicControllerMixin#initFcController(org.objectweb.fractal.julia.InitializationContext)
*/
private void initFcController$2(final org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* The {@link Component} interface of the component to which this controller
* object belongs.
*
* @see org.objectweb.fractal.julia.UseComponentMixin#weaveableC
*/
public org.objectweb.fractal.api.Component weaveableC;
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
*
*
* @see org.objectweb.fractal.koch.control.interceptor.CollectionItfContentControllerMixin#getFcInternalInterface(java.lang.String)
*/
public java.lang.Object getFcInternalInterface(final java.lang.String interfaceName) throws org.objectweb.fractal.api.NoSuchInterfaceException {
if (interfaceName.startsWith("/koch/collection/")) {
return fcInternalInterfaces.get(interfaceName);
}
return getFcInternalInterface$0(interfaceName);
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* The {@link LifeCycleController} interface of the component to which this
* controller object belongs.
*
* @see org.objectweb.fractal.julia.control.lifecycle.UseLifeCycleControllerMixin#weaveableOptLC
*/
public org.objectweb.fractal.api.control.LifeCycleController weaveableOptLC;
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* Checks that the given component is not already a sub component, and then
* calls the overriden method. This method also checks that the addition of
* this sub component will not create a cycle in the component hierarchy.
*
* @param subComponent
* the component to be added inside this component.
* @throws IllegalContentException
* if the given component cannot be added
* inside this component.
* @throws IllegalLifeCycleException
* if this component has a {@link org.objectweb.fractal.api.control.LifeCycleController} interface, but it is not in an appropriate state
* to perform this operation.
* @see org.objectweb.fractal.julia.control.content.CheckContentMixin#addFcSubComponent(org.objectweb.fractal.api.Component)
*/
private void addFcSubComponent$0(final org.objectweb.fractal.api.Component subComponent) throws org.objectweb.fractal.api.control.IllegalContentException, org.objectweb.fractal.api.control.IllegalLifeCycleException {
if (containsFcSubComponent(subComponent)) {
throw new org.objectweb.fractal.julia.control.content.ChainedIllegalContentException(null, weaveableC, subComponent, "Already a sub component");
}
// gets the Component interface of this component,
// and checks that it is not equal to 'subComponent'
org.objectweb.fractal.api.Component thisComponent;
try {
thisComponent = ((org.objectweb.fractal.api.Component) (weaveableC.getFcInterface("component")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
throw new org.objectweb.fractal.julia.control.content.ChainedIllegalContentException(e, weaveableC, subComponent, "Cannot check this operation");
}
if (subComponent.equals(thisComponent)) {
throw new org.objectweb.fractal.julia.control.content.ChainedIllegalContentException(null, weaveableC, subComponent, "A component cannot be a sub component of itself");
}
// finds all the direct and indirect sub components of 'subComponent' and,
// for each sub component checks that it is not equal to 'thisComponent'
java.util.List allSubComponents = org.objectweb.fractal.julia.control.content.Util.getAllSubComponents(subComponent);
for (int i = 0; i < allSubComponents.size(); ++i) {
if (allSubComponents.get(i).equals(thisComponent)) {
throw new org.objectweb.fractal.julia.control.content.ChainedIllegalContentException(null, weaveableC, subComponent, "Would create a cycle in the component hierarchy");
}
}
// calls the overriden method
addFcSubComponent$1(subComponent);
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* Calls the overriden method and then notifies the given component it has
* been added in this component. This method does nothing if the given sub
* component does not provide the {@link SuperControllerNotifier} interface.
*
* @param subComponent
* the component to be added inside this component.
* @throws IllegalContentException
* if the given component cannot be added
* inside this component.
* @throws IllegalLifeCycleException
* if this component has a {@link org.objectweb.fractal.api.control.LifeCycleController} interface, but it is not in an appropriate state
* to perform this operation.
* @see org.objectweb.fractal.julia.control.content.SuperContentMixin#addFcSubComponent(org.objectweb.fractal.api.Component)
*/
public void addFcSubComponent(final org.objectweb.fractal.api.Component subComponent) throws org.objectweb.fractal.api.control.IllegalContentException, org.objectweb.fractal.api.control.IllegalLifeCycleException {
addFcSubComponent$0(subComponent);
org.objectweb.fractal.julia.control.content.SuperControllerNotifier scn = getFcSuperControllerNotifier(subComponent);
if (scn != null) {
try {
org.objectweb.fractal.api.Component c = ((org.objectweb.fractal.api.Component) (weaveableC.getFcInterface("component")));
scn.addedToFc(c);
} catch (org.objectweb.fractal.api.NoSuchInterfaceException ignored) {
}
}
}
/**
* The {@link Component} interface of the component to which this controller
* object belongs.
*
* @see org.objectweb.fractal.julia.UseComponentMixin#weaveableOptC
*/
public org.objectweb.fractal.api.Component weaveableOptC;
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* Checks that the component is stopped and then calls the overriden method.
*
* @param subComponent
* the component to be removed from this component.
* @throws IllegalContentException
* if the given component cannot be removed
* from this component.
* @throws IllegalLifeCycleException
* if this component has a {@link LifeCycleController} interface, but it is not in an appropriate state
* to perform this operation.
* @see org.objectweb.fractal.julia.control.content.LifeCycleContentMixin#removeFcSubComponent(org.objectweb.fractal.api.Component)
*/
private void removeFcSubComponent$0(final org.objectweb.fractal.api.Component subComponent) throws org.objectweb.fractal.api.control.IllegalContentException, org.objectweb.fractal.api.control.IllegalLifeCycleException {
if (weaveableOptLC != null) {
java.lang.String state = weaveableOptLC.getFcState();
if (!org.objectweb.fractal.api.control.LifeCycleController.STOPPED.equals(state)) {
throw new org.objectweb.fractal.julia.control.lifecycle.ChainedIllegalLifeCycleException(null, weaveableOptC, "The component is not stopped");
}
}
removeFcSubComponent$1(subComponent);
}
/**
* Initializes the fields of this mixin and then calls the overriden method.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
* @see org.objectweb.fractal.julia.UseComponentMixin#initFcController(org.objectweb.fractal.julia.InitializationContext)
*/
private void initFcController$1(final org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
weaveableC = ((org.objectweb.fractal.api.Component) (ic.getInterface("component")));
weaveableOptC = weaveableC;
initFcController$2(ic);
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* Checks the interface name against the component's type and then calls the
* overriden method. This method also creates the collection interfaces
* when needed, and puts them in the {@link #_this_fcInternalInterfaces} map.
*
* @param interfaceName
* the name of the internal interface that must be
* returned.
* @return the internal interface of the component to which this interface
belongs, whose name is equal to the given name.
* @throws NoSuchInterfaceException
* if there is no such interface.
* @see org.objectweb.fractal.julia.control.content.TypeContentMixin#getFcInternalInterface(java.lang.String)
*/
private java.lang.Object getFcInternalInterface$0(final java.lang.String interfaceName) throws org.objectweb.fractal.api.NoSuchInterfaceException {
org.objectweb.fractal.api.type.ComponentType compType = ((org.objectweb.fractal.api.type.ComponentType) (weaveableC.getFcType()));
org.objectweb.fractal.api.type.InterfaceType itfType;
try {
itfType = compType.getFcInterfaceType(interfaceName);
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
throw new org.objectweb.fractal.julia.ChainedNoSuchInterfaceException(null, weaveableC, interfaceName);
}
java.lang.Object result;
try {
result = getFcInternalInterface$1(interfaceName);
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
if (itfType.isFcCollectionItf()) {
java.lang.String collectionName = "/collection/" + itfType.getFcItfName();
result = getFcInternalInterface$1(collectionName);
result = ((org.objectweb.fractal.julia.ComponentInterface) (result)).clone();
((org.objectweb.fractal.julia.ComponentInterface) (result)).setFcItfName(interfaceName);
fcInternalInterfaces.put(interfaceName, result);
} else {
throw e;
}
}
return result;
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* The internal interfaces of the component to which this controller object
* belongs.
*
* @see org.objectweb.fractal.julia.control.content.BasicContentControllerMixin#fcInternalInterfaces
*/
public java.util.Map fcInternalInterfaces;
/**
* Initializes the fields of this mixin and then calls the overriden method.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
* @see org.objectweb.fractal.julia.control.lifecycle.UseLifeCycleControllerMixin#initFcController(org.objectweb.fractal.julia.InitializationContext)
*/
public void initFcController(final org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
weaveableOptLC = ((org.objectweb.fractal.api.control.LifeCycleController) (ic.getOptionalInterface("lifecycle-controller")));
initFcController$0(ic);
}
/**
* The sub components of the component to which this controller object
* belongs.
*
* @see org.objectweb.fractal.julia.control.content.BasicContentControllerMixin#fcSubComponents
*/
public java.util.List fcSubComponents;
// -------------------------------------------------------------------------
// Fields and methods required by the mixin class in the base class
// -------------------------------------------------------------------------
/**
* The {@link Controller#initFcController initFcController} method overriden
* by this mixin.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
*/
private void initFcController$3(org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* Checks that this operation will not create non local bindings, and then
* calls the overriden method.
*
* @param subComponent
* the component to be removed from this component.
* @throws IllegalContentException
* if the given component cannot be removed
* from this component.
* @throws IllegalLifeCycleException
* if this component has a {@link org.objectweb.fractal.api.control.LifeCycleController} interface, but it is not in an appropriate state
* to perform this operation.
* @see org.objectweb.fractal.julia.control.content.BindingContentMixin#removeFcSubComponent(org.objectweb.fractal.api.Component)
*/
private void removeFcSubComponent$1(final org.objectweb.fractal.api.Component subComponent) throws org.objectweb.fractal.api.control.IllegalContentException, org.objectweb.fractal.api.control.IllegalLifeCycleException {
try {
checkFcRemoveSubComponent(subComponent);
} catch (org.objectweb.fractal.api.control.IllegalBindingException e) {
throw new org.objectweb.fractal.julia.control.content.ChainedIllegalContentException(e, weaveableOptC, subComponent, "Would create non local bindings");
}
removeFcSubComponent$2(subComponent);
}
/**
* Initializes the fields of this mixin and then calls the overriden method.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
* @see org.objectweb.fractal.julia.control.content.BasicContentControllerMixin#initFcController(org.objectweb.fractal.julia.InitializationContext)
*/
private void initFcController$0(final org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
fcInternalInterfaces = ic.internalInterfaces;
initFcController$1(ic);
}
/**
*
*
* @see org.objectweb.fractal.julia.control.content.BasicContentControllerMixin#getFcInternalInterfaces()
*/
public java.lang.Object[] getFcInternalInterfaces() {
if (fcInternalInterfaces == null) {
return new java.lang.Object[0];
}
// returns the names of all the public interfaces in fcInternalInterfaces
// interfaces whose name begins with '/' are private
int size = 0;
java.lang.String[] names = new java.lang.String[fcInternalInterfaces.size()];
names = ((java.lang.String[]) (fcInternalInterfaces.keySet().toArray(names)));
for (int i = 0; i < names.length; ++i) {
if (names[i].charAt(0) != '/') {
++size;
}
}
int index = 0;
java.lang.Object[] itfs = new java.lang.Object[size];
for (int i = 0; i < names.length; ++i) {
if (names[i].charAt(0) != '/') {
itfs[index++] = fcInternalInterfaces.get(names[i]);
}
}
return itfs;
}
/**
* Calls the overriden method and then notifies the given component it has
* been removed from in this component. This method does nothing if the given
* sub component does not provide the {@link SuperControllerNotifier}
* interface.
*
* @param subComponent
* the component to be removed from this component.
* @throws IllegalContentException
* if the given component cannot be removed
* from this component.
* @throws IllegalLifeCycleException
* if this component has a {@link org.objectweb.fractal.api.control.LifeCycleController} interface, but it is not in an appropriate state
* to perform this operation.
* @see org.objectweb.fractal.julia.control.content.SuperContentMixin#removeFcSubComponent(org.objectweb.fractal.api.Component)
*/
public void removeFcSubComponent(final org.objectweb.fractal.api.Component subComponent) throws org.objectweb.fractal.api.control.IllegalContentException, org.objectweb.fractal.api.control.IllegalLifeCycleException {
removeFcSubComponent$0(subComponent);
org.objectweb.fractal.julia.control.content.SuperControllerNotifier scn = getFcSuperControllerNotifier(subComponent);
if (scn != null) {
try {
org.objectweb.fractal.api.Component c = ((org.objectweb.fractal.api.Component) (weaveableC.getFcInterface("component")));
scn.removedFromFc(c);
} catch (org.objectweb.fractal.api.NoSuchInterfaceException ignored) {
}
}
}
/**
* Checks that the removal of the given sub component will not create non
* local bindings.
*
* @param subComponent
* a sub component that will be removed from this
* component.
* @throws IllegalBindingException
* if the removal of the given sub component
* would create non local bindings.
* @see org.objectweb.fractal.julia.control.content.BindingContentMixin#checkFcRemoveSubComponent(org.objectweb.fractal.api.Component)
*/
public void checkFcRemoveSubComponent(final org.objectweb.fractal.api.Component subComponent) throws org.objectweb.fractal.api.control.IllegalBindingException {
org.objectweb.fractal.api.Component parent;
try {
parent = ((org.objectweb.fractal.api.Component) (weaveableOptC.getFcInterface("component")));
if (parent == null) {
return;
}
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
return;
}
org.objectweb.fractal.api.control.BindingController bc;
try {
bc = ((org.objectweb.fractal.api.control.BindingController) (subComponent.getFcInterface("binding-controller")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
bc = null;
}
java.lang.Object[] itfs = subComponent.getFcInterfaces();
for (int i = 0; i < itfs.length; ++i) {
org.objectweb.fractal.api.Interface itf;
org.objectweb.fractal.api.type.InterfaceType itfType;
try {
itf = ((org.objectweb.fractal.api.Interface) (itfs[i]));
itfType = ((org.objectweb.fractal.api.type.InterfaceType) (itf.getFcItfType()));
} catch (java.lang.ClassCastException e) {
continue;
}
if (itfType.isFcClientItf()) {
if (bc != null) {
org.objectweb.fractal.api.Interface sItf;
try {
sItf = ((org.objectweb.fractal.api.Interface) (bc.lookupFc(itf.getFcItfName())));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
continue;
} catch (java.lang.ClassCastException e) {
continue;
}
if (sItf != null) {
checkFcLocalBinding(itf, parent, sItf, null);
}
}
} else {
java.lang.Object[] potentialClients;
try {
potentialClients = org.objectweb.fractal.julia.control.binding.Util.getFcPotentialClientsOf(itf).toArray();
} catch (java.lang.Exception e) {
continue;
}
for (int j = 0; j < potentialClients.length; ++j) {
org.objectweb.fractal.api.Component c = ((org.objectweb.fractal.api.Component) (potentialClients[j]));
java.util.List clientItfs;
try {
clientItfs = org.objectweb.fractal.julia.control.binding.Util.getFcClientItfsBoundTo(c, itf);
} catch (java.lang.Exception e) {
continue;
}
if (clientItfs.size() > 0) {
checkFcLocalBinding(((org.objectweb.fractal.api.Interface) (clientItfs.get(0))), null, itf, parent);
}
}
}
}
}
/**
*
*
* @see org.objectweb.fractal.julia.control.content.BasicContentControllerMixin#getFcInternalInterface(java.lang.String)
*/
private java.lang.Object getFcInternalInterface$1(final java.lang.String interfaceName) throws org.objectweb.fractal.api.NoSuchInterfaceException {
java.lang.Object itf;
if (fcInternalInterfaces == null) {
itf = null;
} else {
itf = fcInternalInterfaces.get(interfaceName);
}
if (itf == null) {
throw new org.objectweb.fractal.julia.ChainedNoSuchInterfaceException(null, weaveableOptC, interfaceName);
}
return itf;
}
/**
*
*
* @see org.objectweb.fractal.julia.control.content.BasicContentControllerMixin#getFcSubComponents()
*/
public org.objectweb.fractal.api.Component[] getFcSubComponents() {
if (fcSubComponents == null) {
return new org.objectweb.fractal.api.Component[0];
}
return ((org.objectweb.fractal.api.Component[]) (fcSubComponents.toArray(new org.objectweb.fractal.api.Component[fcSubComponents.size()])));
}
/**
* Checks that the given component is really a sub component, and then
* calls the overriden method.
*
* @param subComponent
* the component to be removed from this component.
* @throws IllegalContentException
* if the given component cannot be removed
* from this component.
* @throws IllegalLifeCycleException
* if this component has a {@link org.objectweb.fractal.api.control.LifeCycleController} interface, but it is not in an appropriate state
* to perform this operation.
* @see org.objectweb.fractal.julia.control.content.CheckContentMixin#removeFcSubComponent(org.objectweb.fractal.api.Component)
*/
private void removeFcSubComponent$2(final org.objectweb.fractal.api.Component subComponent) throws org.objectweb.fractal.api.control.IllegalContentException, org.objectweb.fractal.api.control.IllegalLifeCycleException {
if (!containsFcSubComponent(subComponent)) {
throw new org.objectweb.fractal.julia.control.content.ChainedIllegalContentException(null, weaveableC, subComponent, "Not a sub component");
}
removeFcSubComponent$3(subComponent);
}
/**
* Returns the {@link SuperControllerNotifier} interface of the given
* component.
*
* @param c
* a component.
* @return the {@link SuperControllerNotifier} interface of the given
component, or null.
* @see org.objectweb.fractal.julia.control.content.SuperContentMixin#getFcSuperControllerNotifier(org.objectweb.fractal.api.Component)
*/
private org.objectweb.fractal.julia.control.content.SuperControllerNotifier getFcSuperControllerNotifier(final org.objectweb.fractal.api.Component c) {
try {
return ((org.objectweb.fractal.julia.control.content.SuperControllerNotifier) (c.getFcInterface("super-controller")));
} catch (java.lang.Exception e) {
try {
return ((org.objectweb.fractal.julia.control.content.SuperControllerNotifier) (c.getFcInterface("/super-controller-notifier")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException ignored) {
return null;
}
}
}
/**
*
*
* @see org.objectweb.fractal.julia.control.content.BasicContentControllerMixin#addFcSubComponent(org.objectweb.fractal.api.Component)
*/
private void addFcSubComponent$1(final org.objectweb.fractal.api.Component subComponent) throws org.objectweb.fractal.api.control.IllegalContentException, org.objectweb.fractal.api.control.IllegalLifeCycleException {
if (fcSubComponents == null) {
fcSubComponents = new java.util.ArrayList();
}
fcSubComponents.add(subComponent);
}
/**
* The {@link ContentController#getFcInternalInterface getFcInternalInterface}
* method overriden by this mixin.
*
* @param interfaceName
* the name of the internal interface that must be
* returned.
* @return the internal interface of the component to which this interface
belongs, whose name is equal to the given name.
* @throws NoSuchInterfaceException
* if there is no such interface.
*/
private java.lang.Object getFcInternalInterface$2(java.lang.String interfaceName) throws org.objectweb.fractal.api.NoSuchInterfaceException {
return null;
}
/**
*
*
* @see org.objectweb.fractal.julia.control.content.BasicContentControllerMixin#removeFcSubComponent(org.objectweb.fractal.api.Component)
*/
private void removeFcSubComponent$3(final org.objectweb.fractal.api.Component subComponent) throws org.objectweb.fractal.api.control.IllegalContentException, org.objectweb.fractal.api.control.IllegalLifeCycleException {
if (fcSubComponents != null) {
fcSubComponents.remove(subComponent);
}
}
/**
* Tests if this component contains the given sub component.
*
* @param subComponent
* a component.
* @return true if this component contains the given sub component,
or false otherwise.
* @see org.objectweb.fractal.julia.control.content.CheckContentMixin#containsFcSubComponent(org.objectweb.fractal.api.Component)
*/
public boolean containsFcSubComponent(final org.objectweb.fractal.api.Component subComponent) {
org.objectweb.fractal.api.Component[] subComponents = getFcSubComponents();
for (int i = 0; i < subComponents.length; ++i) {
if (subComponents[i].equals(subComponent)) {
return true;
}
}
return false;
}
/**
* Checks that a given binding is a local binding.
*
* @param cItf
* a client interface.
* @param cId
* the parent from which the client component has been removed, or
* null if the client component has not been removed from a
* parent component.
* @param sItf
* the server interface to which the client interface is bound.
* @param sId
* the parent from which the server component has been removed, or
* null if the server component has not been removed from a
* parent component.
* @throws IllegalBindingException
* if the given binding is not a local
* binding.
* @see org.objectweb.fractal.julia.control.content.BindingContentMixin#checkFcLocalBinding(org.objectweb.fractal.api.Interface,org.objectweb.fractal.api.Component,org.objectweb.fractal.api.Interface,org.objectweb.fractal.api.Component)
*/
private void checkFcLocalBinding(final org.objectweb.fractal.api.Interface cItf, final org.objectweb.fractal.api.Component cId, final org.objectweb.fractal.api.Interface sItf, final org.objectweb.fractal.api.Component sId) throws org.objectweb.fractal.api.control.IllegalBindingException {
org.objectweb.fractal.api.Component client = cItf.getFcItfOwner();
org.objectweb.fractal.api.Component server = sItf.getFcItfOwner();
if (client.equals(server)) {
return;
}
org.objectweb.fractal.api.control.SuperController cSc = null;
org.objectweb.fractal.api.control.SuperController sSc = null;
try {
cSc = ((org.objectweb.fractal.api.control.SuperController) (client.getFcInterface("super-controller")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException ignored) {
}
try {
sSc = ((org.objectweb.fractal.api.control.SuperController) (server.getFcInterface("super-controller")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException ignored) {
}
if (cItf.isFcInternalItf()) {
// check client component is a parent of server component
if (cSc != null) {
org.objectweb.fractal.api.Component[] sP = sSc.getFcSuperComponents();
for (int i = 0; i < sP.length; ++i) {
org.objectweb.fractal.api.Component p = sP[i];
if ((sId == null) || (!p.equals(sId))) {
if (p.equals(client)) {
return;
}
}
}
} else {
return;
}
} else if (sItf.isFcInternalItf()) {
// check server component is a parent of client component
if (cSc != null) {
org.objectweb.fractal.api.Component[] cP = cSc.getFcSuperComponents();
for (int i = 0; i < cP.length; ++i) {
org.objectweb.fractal.api.Component p = cP[i];
if ((cId == null) || (!p.equals(cId))) {
if (p.equals(server)) {
return;
}
}
}
} else {
return;
}
} else // check client and server components have a common parent
if ((cSc != null) && (sSc != null)) {
org.objectweb.fractal.api.Component[] cP = cSc.getFcSuperComponents();
org.objectweb.fractal.api.Component[] sP = sSc.getFcSuperComponents();
for (int i = 0; i < cP.length; ++i) {
org.objectweb.fractal.api.Component p = cP[i];
if ((cId == null) || (!p.equals(cId))) {
for (int j = 0; j < sP.length; ++j) {
org.objectweb.fractal.api.Component q = sP[j];
if ((sId == null) || (!q.equals(sId))) {
if (p.equals(q)) {
return;
}
}
}
}
}
} else {
return;
}
throw new org.objectweb.fractal.julia.control.binding.ChainedIllegalBindingException(null, weaveableOptC, sId, cItf.getFcItfName(), sItf.getFcItfName(), "Not a local binding");
}
/**
* The {@link ContentController#addFcSubComponent addFcSubComponent} method
* overriden by this mixin.
*
* @param subComponent
* the component to be added inside this component.
* @throws IllegalContentException
* if the given component cannot be added
* inside this component.
* @throws IllegalLifeCycleException
* if this component has a {@link org.objectweb.fractal.api.control.LifeCycleController} interface, but it is not in an appropriate state
* to perform this operation.
*/
private void addFcSubComponent$2(org.objectweb.fractal.api.Component subComponent) throws org.objectweb.fractal.api.control.IllegalContentException, org.objectweb.fractal.api.control.IllegalLifeCycleException {
}
/**
* The {@link ContentController#removeFcSubComponent removeFcSubComponent}
* method overriden by this mixin.
*
* @param subComponent
* the component to be removed from this component.
* @throws IllegalContentException
* if the given component cannot be removed
* from this component.
* @throws IllegalLifeCycleException
* if this component has a {@link org.objectweb.fractal.api.control.LifeCycleController} interface, but it is not in an appropriate state
* to perform this operation.
*/
private void removeFcSubComponent$4(org.objectweb.fractal.api.Component subComponent) throws org.objectweb.fractal.api.control.IllegalContentException, org.objectweb.fractal.api.control.IllegalLifeCycleException {
}
public java.lang.String getFcGeneratorParameters() {
return "(org.objectweb.fractal.juliac.spoon.MixinClassGenerator juliac.ContentControllerImpl org.objectweb.fractal.julia.BasicControllerMixin org.objectweb.fractal.julia.UseComponentMixin org.objectweb.fractal.julia.control.content.BasicContentControllerMixin org.objectweb.fractal.julia.control.content.CheckContentMixin org.objectweb.fractal.julia.control.content.TypeContentMixin org.objectweb.fractal.koch.control.interceptor.CollectionItfContentControllerMixin org.objectweb.fractal.julia.control.content.BindingContentMixin org.objectweb.fractal.julia.control.lifecycle.UseLifeCycleControllerMixin org.objectweb.fractal.julia.control.content.LifeCycleContentMixin org.objectweb.fractal.julia.control.content.SuperContentMixin)";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy