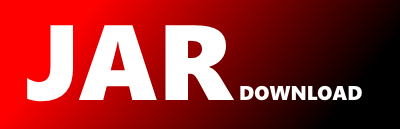
juliac.FactoryImpl Maven / Gradle / Ivy
The newest version!
package juliac;
/**
*
*
* @see org.objectweb.fractal.julia.BasicControllerMixin
* @see org.objectweb.fractal.julia.UseComponentMixin
* @see org.objectweb.fractal.julia.factory.BasicTemplateMixin
* @see org.objectweb.fractal.julia.control.attribute.UseCloneableAttributeControllerMixin
* @see org.objectweb.fractal.julia.factory.AttributeTemplateMixin
* @see org.objectweb.fractal.julia.control.name.UseNameControllerMixin
* @see org.objectweb.fractal.julia.factory.NameTemplateMixin
*/
public class FactoryImpl implements org.objectweb.fractal.julia.loader.Generated , org.objectweb.fractal.julia.Controller , org.objectweb.fractal.julia.factory.Template {
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* Does nothing.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
* @see org.objectweb.fractal.julia.BasicControllerMixin#initFcController(org.objectweb.fractal.julia.InitializationContext)
*/
private void initFcController$3(final org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* The {@link Component} interface of the component to which this controller
* object belongs.
*
* @see org.objectweb.fractal.julia.UseComponentMixin#weaveableC
*/
public org.objectweb.fractal.api.Component weaveableC;
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* The {@link NameController} interface of the component to which this
* controller object belongs.
*
* @see org.objectweb.fractal.julia.control.name.UseNameControllerMixin#weaveableOptNC
*/
public org.objectweb.fractal.api.control.NameController weaveableOptNC;
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* The {@link CloneableAttributeController} interface of the component to
* which this controller object belongs.
*
* @see org.objectweb.fractal.julia.control.attribute.UseCloneableAttributeControllerMixin#weaveableOptCAC
*/
public org.objectweb.fractal.julia.control.attribute.CloneableAttributeController weaveableOptCAC;
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* Calls the overriden method and then sets the name of the created component.
* The name of the created component is initialized to the name of this
* template, if both components have a {@link NameController} interface
* (otherwise this mixin does nothing).
*
* @return the instantiated component.
* @throws InstantiationException
* if the component controller cannot be
* instantiated.
* @see org.objectweb.fractal.julia.factory.NameTemplateMixin#newFcControllerInstance()
*/
public org.objectweb.fractal.api.Component newFcControllerInstance() throws org.objectweb.fractal.api.factory.InstantiationException {
org.objectweb.fractal.api.Component comp = newFcControllerInstance$0();
if (weaveableOptNC != null) {
try {
// copies the name of this template to the component, if applicable
java.lang.String name = weaveableOptNC.getFcName();
((org.objectweb.fractal.api.control.NameController) (comp.getFcInterface("name-controller"))).setFcName(name);
} catch (org.objectweb.fractal.api.NoSuchInterfaceException ignored) {
}
}
return comp;
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* Calls the overriden method and then sets the attributes of the created
* component. The attributes of the created component are initialized from the
* attributes name of this template, if the template has a {@link CloneableAttributeController} interface, and if the created component has
* an {@link org.objectweb.fractal.api.control.AttributeController} interface
* (otherwise this mixin does nothing).
*
* @return the instantiated component.
* @throws InstantiationException
* if the component controller cannot be
* instantiated.
* @see org.objectweb.fractal.julia.factory.AttributeTemplateMixin#newFcControllerInstance()
*/
private org.objectweb.fractal.api.Component newFcControllerInstance$0() throws org.objectweb.fractal.api.factory.InstantiationException {
org.objectweb.fractal.api.Component comp = newFcControllerInstance$1();
if (weaveableOptCAC != null) {
// copies the template's attributes into the component, if applicable
try {
weaveableOptCAC.cloneFcAttributes(((org.objectweb.fractal.api.control.AttributeController) (comp.getFcInterface("attribute-controller"))));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException ignored) {
}
}
return comp;
}
/**
* The {@link Component} interface of the component to which this controller
* object belongs.
*
* @see org.objectweb.fractal.julia.UseComponentMixin#weaveableOptC
*/
public org.objectweb.fractal.api.Component weaveableOptC;
/**
* Initializes the fields of this mixin and then calls the overriden method.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
* @see org.objectweb.fractal.julia.UseComponentMixin#initFcController(org.objectweb.fractal.julia.InitializationContext)
*/
private void initFcController$2(final org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
weaveableC = ((org.objectweb.fractal.api.Component) (ic.getInterface("component")));
weaveableOptC = weaveableC;
initFcController$3(ic);
}
/**
* Initializes the fields of this mixin and then calls the overriden method.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
* @see org.objectweb.fractal.julia.control.name.UseNameControllerMixin#initFcController(org.objectweb.fractal.julia.InitializationContext)
*/
public void initFcController(final org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
weaveableOptNC = ((org.objectweb.fractal.api.control.NameController) (ic.getOptionalInterface("name-controller")));
initFcController$0(ic);
}
/**
* Initializes the fields of this mixin and then calls the overriden method.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
* @see org.objectweb.fractal.julia.control.attribute.UseCloneableAttributeControllerMixin#initFcController(org.objectweb.fractal.julia.InitializationContext)
*/
private void initFcController$0(final org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
weaveableOptCAC = ((org.objectweb.fractal.julia.control.attribute.CloneableAttributeController) (ic.getOptionalInterface("/cloneable-attribute-controller")));
initFcController$1(ic);
}
// -------------------------------------------------------------------------
// Fields and methods required by the mixin class in the base class
// -------------------------------------------------------------------------
/**
* The {@link Controller#initFcController initFcController} method overriden
* by this mixin.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
*/
private void initFcController$4(org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* The functional type of the components instantiated by this template.
*
* @see org.objectweb.fractal.julia.factory.BasicTemplateMixin#fcInstanceType
*/
public org.objectweb.fractal.api.Type fcInstanceType;
/**
* The controller and content descriptors of the components instantiated by
* this template.
*
* @see org.objectweb.fractal.julia.factory.BasicTemplateMixin#fcContent
*/
public java.lang.Object[] fcContent;
/**
* The {@link Template#newFcControllerInstance newFcControllerInstance}
* overriden by this mixin.
*
* @return the instantiated component.
* @throws InstantiationException
* if the component controller cannot be
* instantiated.
*/
private org.objectweb.fractal.api.Component newFcControllerInstance$2() throws org.objectweb.fractal.api.factory.InstantiationException {
return null;
}
/**
* Initializes the fields of this mixin and then calls the overriden method.
*
* @param ic
* information about the component to which this controller object
* belongs.
* @throws InstantiationException
* if the initialization fails.
* @see org.objectweb.fractal.julia.factory.BasicTemplateMixin#initFcController(org.objectweb.fractal.julia.InitializationContext)
*/
private void initFcController$1(final org.objectweb.fractal.julia.InitializationContext ic) throws org.objectweb.fractal.api.factory.InstantiationException {
// initializes the fcContent field
fcContent = ((java.lang.Object[]) (ic.content));
// initializes the fcInstanceType field
// this type is computed from the type of this component by removing the
// factory interface type, and all the control interface types
org.objectweb.fractal.api.type.ComponentType tmplType = ((org.objectweb.fractal.api.type.ComponentType) (ic.type));
org.objectweb.fractal.api.type.InterfaceType[] itfTypes = tmplType.getFcInterfaceTypes();
java.util.List itfList = new java.util.ArrayList();
for (int j = 0; j < itfTypes.length; ++j) {
java.lang.String n = itfTypes[j].getFcItfName();
if ((!n.equals("factory")) && (!n.equals("/template"))) {
if (n.equals("attribute-controller")) {
itfList.add(itfTypes[j]);
} else if (!(n.equals("component") || n.endsWith("-controller"))) {
itfList.add(itfTypes[j]);
}
}
}
itfTypes = new org.objectweb.fractal.api.type.InterfaceType[itfList.size()];
itfTypes = ((org.objectweb.fractal.api.type.InterfaceType[]) (itfList.toArray(itfTypes)));
fcInstanceType = new org.objectweb.fractal.julia.type.BasicComponentType(itfTypes);
// calls the overriden method
initFcController$2(ic);
}
/**
*
*
* @see org.objectweb.fractal.julia.factory.BasicTemplateMixin#getFcInstanceType()
*/
public org.objectweb.fractal.api.Type getFcInstanceType() {
return fcInstanceType;
}
/**
*
*
* @see org.objectweb.fractal.julia.factory.BasicTemplateMixin#getFcControllerDesc()
*/
public java.lang.Object getFcControllerDesc() {
return fcContent[0];
}
/**
*
*
* @see org.objectweb.fractal.julia.factory.BasicTemplateMixin#getFcContentDesc()
*/
public java.lang.Object getFcContentDesc() {
return fcContent[1];
}
/**
*
*
* @see org.objectweb.fractal.julia.factory.BasicTemplateMixin#newFcInstance()
*/
public org.objectweb.fractal.api.Component newFcInstance() throws org.objectweb.fractal.api.factory.InstantiationException {
// finds all the direct and indirect sub templates of this template
// finds all the direct and indirect sub components of this component
org.objectweb.fractal.api.Component thisComponent;
try {
thisComponent = ((org.objectweb.fractal.api.Component) (weaveableC.getFcInterface("component")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
throw new org.objectweb.fractal.julia.factory.ChainedInstantiationException(e, weaveableC, "The template component must provide the Component interface");
}
java.util.List allSubTemplates = org.objectweb.fractal.julia.control.content.Util.getAllSubComponents(thisComponent);
// instantiates all the templates
java.util.Map instances = new java.util.HashMap();
for (int i = 0; i < allSubTemplates.size(); ++i) {
org.objectweb.fractal.api.Component tmpl = ((org.objectweb.fractal.api.Component) (allSubTemplates.get(i)));
org.objectweb.fractal.julia.factory.Template t;
try {
t = ((org.objectweb.fractal.julia.factory.Template) (tmpl.getFcInterface("factory")));
} catch (java.lang.Exception e) {
try {
t = ((org.objectweb.fractal.julia.factory.Template) (tmpl.getFcInterface("/template")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException f) {
throw new org.objectweb.fractal.julia.factory.ChainedInstantiationException(f, weaveableC, "All the (sub) templates must provide the Template interface");
}
}
org.objectweb.fractal.api.Component instance = t.newFcControllerInstance();
instances.put(tmpl, instance);
}
// adds the instances into each other
for (int i = 0; i < allSubTemplates.size(); ++i) {
org.objectweb.fractal.api.Component tmpl = ((org.objectweb.fractal.api.Component) (allSubTemplates.get(i)));
org.objectweb.fractal.api.Component instance = ((org.objectweb.fractal.api.Component) (instances.get(tmpl)));
org.objectweb.fractal.api.control.ContentController tmplCC;
org.objectweb.fractal.api.control.ContentController instanceCC;
try {
tmplCC = ((org.objectweb.fractal.api.control.ContentController) (tmpl.getFcInterface("content-controller")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
continue;
}
try {
instanceCC = ((org.objectweb.fractal.api.control.ContentController) (instance.getFcInterface("content-controller")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
throw new org.objectweb.fractal.julia.factory.ChainedInstantiationException(e, tmpl, "A component instantiated from a template with a ContentController " + "interface must provide the ContentController interface");
}
org.objectweb.fractal.api.Component[] subTemplates = tmplCC.getFcSubComponents();
org.objectweb.fractal.api.Component[] subInstances = instanceCC.getFcSubComponents();
for (int j = 0; j < subTemplates.length; ++j) {
org.objectweb.fractal.api.Component subInstance = ((org.objectweb.fractal.api.Component) (instances.get(subTemplates[j])));
boolean add = true;
for (int k = 0; k < subInstances.length; ++k) {
if (subInstances[k].equals(subInstance)) {
// if sunInstance is already a sub component of instance,
// do not add it again (this can happen with singleton templates)
add = false;
}
}
if (add) {
try {
instanceCC.addFcSubComponent(subInstance);
} catch (org.objectweb.fractal.api.control.IllegalContentException e) {
throw new org.objectweb.fractal.julia.factory.ChainedInstantiationException(e, tmpl, "Cannot set the component hierarchy from the template hierarchy");
} catch (org.objectweb.fractal.api.control.IllegalLifeCycleException e) {
throw new org.objectweb.fractal.julia.factory.ChainedInstantiationException(e, tmpl, "Cannot set the component hierarchy from the template hierarchy");
}
}
}
}
// binds the instances to each other, as the templates are bound
for (int i = 0; i < allSubTemplates.size(); ++i) {
org.objectweb.fractal.api.Component tmpl = ((org.objectweb.fractal.api.Component) (allSubTemplates.get(i)));
org.objectweb.fractal.api.Component instance = ((org.objectweb.fractal.api.Component) (instances.get(tmpl)));
org.objectweb.fractal.api.control.BindingController tmplBC;
org.objectweb.fractal.api.control.BindingController instanceBC;
try {
tmplBC = ((org.objectweb.fractal.api.control.BindingController) (tmpl.getFcInterface("binding-controller")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
continue;
}
try {
instanceBC = ((org.objectweb.fractal.api.control.BindingController) (instance.getFcInterface("binding-controller")));
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
throw new org.objectweb.fractal.julia.factory.ChainedInstantiationException(e, tmpl, "A component instantiated from a template with a BindingController " + "interface must provide the BindingController interface");
}
java.lang.String[] itfNames = tmplBC.listFc();
for (int j = 0; j < itfNames.length; ++j) {
java.lang.String itfName = itfNames[j];
org.objectweb.fractal.api.Interface serverItf;
try {
serverItf = ((org.objectweb.fractal.api.Interface) (tmplBC.lookupFc(itfName)));
} catch (java.lang.ClassCastException e) {
throw new org.objectweb.fractal.julia.factory.ChainedInstantiationException(e, tmpl, "The server interface of each binding between templates " + "must implement the Interface interface");
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
throw new org.objectweb.fractal.julia.factory.ChainedInstantiationException(e, tmpl, ("The '" + itfName) + "' interface returned by the listFc method does not exist");
}
if (serverItf == null) {
continue;
}
org.objectweb.fractal.api.Component serverTmpl = serverItf.getFcItfOwner();
org.objectweb.fractal.api.Component serverInstance = ((org.objectweb.fractal.api.Component) (instances.get(serverTmpl)));
if (serverInstance == null) {
// 'tmpl' bound to a component that does not belong to the root
// instantiated template: do not create this binding for 'instance'
continue;
}
java.lang.Object itfValue;
try {
if (serverItf.isFcInternalItf()) {
org.objectweb.fractal.api.control.ContentController cc = ((org.objectweb.fractal.api.control.ContentController) (serverInstance.getFcInterface("content-controller")));
itfValue = cc.getFcInternalInterface(serverItf.getFcItfName());
} else {
itfValue = serverInstance.getFcInterface(serverItf.getFcItfName());
}
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
throw new org.objectweb.fractal.julia.factory.ChainedInstantiationException(e, serverTmpl, ("The server interface '" + serverItf.getFcItfName()) + "'is missing in the component instantiated from the template");
}
try {
if (instanceBC.lookupFc(itfName).equals(itfValue)) {
// if the binding already exists, do not create again
// (this can happen with singleton templates)
continue;
}
} catch (java.lang.Exception e) {
}
try {
instanceBC.bindFc(itfName, itfValue);
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
throw new org.objectweb.fractal.julia.factory.ChainedInstantiationException(e, tmpl, "Cannot set the component bindings from the template bindings");
} catch (org.objectweb.fractal.api.control.IllegalBindingException e) {
throw new org.objectweb.fractal.julia.factory.ChainedInstantiationException(e, tmpl, "Cannot set the component bindings from the template bindings");
} catch (org.objectweb.fractal.api.control.IllegalLifeCycleException e) {
throw new org.objectweb.fractal.julia.factory.ChainedInstantiationException(e, tmpl, "Cannot set the component bindings from the template bindings");
}
}
}
return ((org.objectweb.fractal.api.Component) (instances.get(allSubTemplates.get(0))));
}
/**
*
*
* @see org.objectweb.fractal.julia.factory.BasicTemplateMixin#newFcControllerInstance()
*/
private org.objectweb.fractal.api.Component newFcControllerInstance$1() throws org.objectweb.fractal.api.factory.InstantiationException {
if (fcContent[1] instanceof org.objectweb.fractal.api.Component) {
return ((org.objectweb.fractal.api.Component) (fcContent[1]));
}
org.objectweb.fractal.api.factory.GenericFactory factory;
try {
factory = ((org.objectweb.fractal.api.factory.GenericFactory) (org.objectweb.fractal.api.Fractal.getBootstrapComponent().getFcInterface("generic-factory")));
} catch (org.objectweb.fractal.api.factory.InstantiationException e) {
throw new org.objectweb.fractal.julia.factory.ChainedInstantiationException(e, weaveableC, "Cannot find the GenericFactory interface of the bootstrap " + "component, which is needed to instantiate the template");
} catch (org.objectweb.fractal.api.NoSuchInterfaceException e) {
throw new org.objectweb.fractal.julia.factory.ChainedInstantiationException(e, weaveableC, "Cannot find the GenericFactory interface of the bootstrap " + "component, which is needed to instantiate the template");
}
return factory.newFcInstance(fcInstanceType, fcContent[0], fcContent[1]);
}
public java.lang.String getFcGeneratorParameters() {
return "(org.objectweb.fractal.juliac.spoon.MixinClassGenerator juliac.FactoryImpl org.objectweb.fractal.julia.BasicControllerMixin org.objectweb.fractal.julia.UseComponentMixin org.objectweb.fractal.julia.factory.BasicTemplateMixin org.objectweb.fractal.julia.control.attribute.UseCloneableAttributeControllerMixin org.objectweb.fractal.julia.factory.AttributeTemplateMixin org.objectweb.fractal.julia.control.name.UseNameControllerMixin org.objectweb.fractal.julia.factory.NameTemplateMixin)";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy