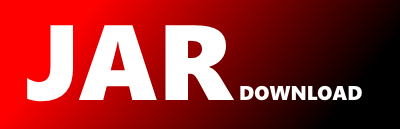
org.objectweb.fractal.juliac.runtime.comp.JuliacBootstrapComponentImpl Maven / Gradle / Ivy
/***
* Juliac
* Copyright (C) 2007-2021 Inria, Univ. Lille
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Contact: [email protected]
*
* Author: Lionel Seinturier
*/
package org.objectweb.fractal.juliac.runtime.comp;
import java.util.HashMap;
import java.util.Map;
import org.objectweb.fractal.api.Component;
import org.objectweb.fractal.api.Type;
import org.objectweb.fractal.api.factory.InstantiationException;
import org.objectweb.fractal.api.type.InterfaceType;
import org.objectweb.fractal.julia.type.BasicComponentType;
import org.objectweb.fractal.juliac.runtime.ClassLoaderFcItf;
import org.objectweb.fractal.juliac.runtime.Factory;
import org.objectweb.fractal.juliac.runtime.GenericFactoryFcItf;
import org.objectweb.fractal.juliac.runtime.TypeFactoryFcItf;
import org.objectweb.fractal.juliac.runtime.RuntimeException;
import org.objectweb.fractal.koch.factory.MembraneFactory;
/**
* The Juliac bootstrap component for the COMP optimization level.
*
* This bootstrap component provides a type-factory, a generic-factory, a
* membrane-factory and a classloader interface.
*
* @author Lionel Seinturier
* @since 2.0
*/
public class JuliacBootstrapComponentImpl
extends org.objectweb.fractal.juliac.runtime.JuliacBootstrapComponentImpl
implements MembraneFactory {
// -----------------------------------------------------------------
// Implementation of the GenericFactory interface
// -----------------------------------------------------------------
/**
* Create a new component instance.
*
* @param type the component type
* @param controllerDesc the component controller descriptor
* @param contentDesc the component content descriptor
*/
@Override
public Component newFcInstance(
Type type, Object controllerDesc, Object contentDesc )
throws InstantiationException {
/*
* If the specified controllerDesc is a Component, consider that this is
* the control membrane which is to be associated with the component.
*/
if( controllerDesc instanceof Component ) {
// TODO Koch-like factory
throw new UnsupportedOperationException();
}
Component comp = super.newFcInstance(type,controllerDesc,contentDesc);
return comp;
}
// -----------------------------------------------------------------
// Implementation of the MembraneFactory interface
// -----------------------------------------------------------------
/** The map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy