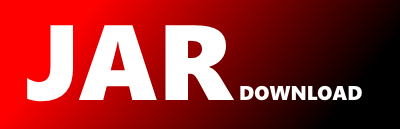
org.objectweb.fractal.juliac.runtime.comp.MembraneInitializer Maven / Gradle / Ivy
/***
* Juliac
* Copyright (C) 2019-2021 Inria, Univ. Lille
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Contact: [email protected]
*
* Author: Lionel Seinturier
*/
package org.objectweb.fractal.juliac.runtime.comp;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.objectweb.fractal.api.Component;
import org.objectweb.fractal.api.NoSuchInterfaceException;
import org.objectweb.fractal.api.control.ContentController;
import org.objectweb.fractal.api.control.LifeCycleController;
import org.objectweb.fractal.api.factory.Factory;
import org.objectweb.fractal.api.factory.InstantiationException;
import org.objectweb.fractal.julia.ComponentInterface;
import org.objectweb.fractal.julia.Controller;
import org.objectweb.fractal.julia.InitializationContext;
import org.objectweb.fractal.julia.Interceptor;
import org.objectweb.fractal.julia.factory.ChainedInstantiationException;
import org.objectweb.fractal.koch.factory.ControlComponentBoundMap;
import org.objectweb.fractal.util.ContentControllerHelper;
/**
* Root class for initializer class for Fractal components with a
* component-based control membrane.
*
* @author Lionel Seinturier
* @since 2.8
*/
public class MembraneInitializer {
/**
* Instantiate and start a membrane with the specified membrane factory.
*/
protected Component instantiateAndStartMembrane( Factory membraneFactory )
throws InstantiationException {
Component membrane = membraneFactory.newFcInstance();
try {
LifeCycleController membranelc = (LifeCycleController)
membrane.getFcInterface("lifecycle-controller");
membranelc.startFc();
return membrane;
}
catch( Exception e ) {
throw new ChainedInstantiationException(e,null,e.getMessage());
}
}
/**
* Retrieve all controllers contained in the specified membrane.
*/
protected void retrieveAllControllers(
Component membrane, InitializationContext ic, Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy