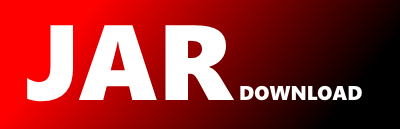
org.ow2.frascati.tinfi.IntentJoinPointImpl Maven / Gradle / Ivy
The newest version!
/***
* OW2 FraSCAti Tinfi
* Copyright (C) 2008-2018 Inria, Univ. Lille
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Contact: [email protected]
*
* Author: Lionel Seinturier
* Contributor: Philippe Merle
*/
package org.ow2.frascati.tinfi;
import java.lang.reflect.AccessibleObject;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.List;
import org.objectweb.fractal.api.Component;
import org.objectweb.fractal.api.Interface;
import org.ow2.frascati.tinfi.api.IntentHandler;
import org.ow2.frascati.tinfi.api.IntentJoinPoint;
/**
* Implementation of the {@link IntentJoinPoint} interface for introspecting
* intent join points. An intent join point corresponds to the interface where a
* service or reference invocation has been intercepted.
* @author Lionel Seinturier
* @author Philippe Merle
* @since 0.3
*/
public class IntentJoinPointImpl implements IntentJoinPoint {
protected List handlers;
protected int index = 0;
protected Component intentComponent;
protected Interface intentItf;
protected T intentTarget;
private Method intentMethod;
protected Object[] intentMethodArguments;
/**
* Initialize this intent join point.
*/
public void init(
List handlers,
Component intentComponent,
Interface intentItf,
T intentTarget,
Method intentMethod,
Object... intentMethodArguments ) {
this.handlers = handlers;
this.intentComponent = intentComponent;
this.intentItf = intentItf;
this.intentTarget = intentTarget;
this.intentMethod = intentMethod;
this.intentMethodArguments = intentMethodArguments;
}
public Component getComponent() {
return intentComponent;
}
public Interface getInterface() {
return intentItf;
}
public Object[] getArguments() {
return intentMethodArguments;
}
public Method getMethod() {
return intentMethod;
}
public AccessibleObject getStaticPart() {
return intentMethod;
}
public T getThis() {
return intentTarget;
}
public Object proceed() throws Throwable {
if( index == handlers.size() ) {
try {
Object ret =
intentMethod.invoke(intentTarget,intentMethodArguments);
return ret;
}
catch( InvocationTargetException ite ) {
// Throw the exception thrown by the invoked method
Throwable t = ite.getTargetException();
throw t;
}
/*
* The following commented lines of code correspond to a feature
* which has been removed in version 1.3.
*
* See SCAIntentInterceptorSourceCodeGenerator#generateFields() for
* further information.
*/
// String msg = "Unexpected case: index==handlers.length should have been checked by the subclass";
// throw new TinfiRuntimeException(msg);
}
IntentHandler handler = handlers.get(index);
index++;
Object ret = handler.invoke(this);
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy