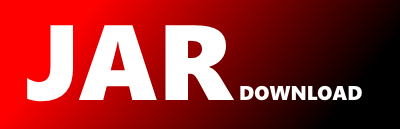
org.ow2.frascati.implementation.script.ScriptEngineComponent Maven / Gradle / Ivy
The newest version!
/**
* OW2 FraSCAti: SCA Implementation Script
* Copyright (C) 2009-2010 INRIA, USTL
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Contact: [email protected]
*
* Author: Philippe Merle
* Contributor(s):
*/
package org.ow2.frascati.implementation.script;
import javax.script.ScriptEngine;
import org.objectweb.fractal.api.Component;
import org.objectweb.fractal.api.NoSuchInterfaceException;
import org.objectweb.fractal.api.control.IllegalLifeCycleException;
import org.objectweb.fractal.api.control.LifeCycleController;
import org.ow2.frascati.tinfi.api.control.SCAPropertyController;
import org.ow2.frascati.util.AbstractLoggeable;
/**
* Implementation of a scripting engine component.
*
* @author Philippe Merle
*/
public class ScriptEngineComponent
extends AbstractLoggeable
implements ScriptEngineAttributes, LifeCycleController {
/**
* The enclosing SCA component implemented by the script.
*/
// private Component scaComponent;
/**
* The SCA property controller of the enclosing SCA component.
*/
private SCAPropertyController scaPropertyController;
/**
* The scripting engine executing the script.
*/
private ScriptEngine scriptEngine;
/**
* The state of this Fractal component.
*/
private String fcState;
/**
* Constructor.
*
* @param scaComponent the enclosing SCA component implemented by the script.
* @param scriptEngine the scripting engine executing the script.
*/
public ScriptEngineComponent(Component scaComponent, ScriptEngine scriptEngine) {
// this.scaComponent = scaComponent;
this.scriptEngine = scriptEngine;
// Obtain the SCA property controller of the enclosing SCA component.
try {
scaPropertyController = (SCAPropertyController) scaComponent
.getFcInterface("sca-property-controller");
} catch(NoSuchInterfaceException nsie) {
// Must never happen!!!
throw new Error("Internal FraSCAti error!", nsie);
}
}
/**
* @see ScriptingEngineAttributes.getLanguage()
*/
public final String getLanguage() {
return null; // TODO
}
/**
* @see ScriptingEngineAttributes.getScript()
*/
public final String getScript() {
return null; // TODO
}
/**
* @see ScriptingEngineAttributes.setScript(String)
*/
public final void setScript(String script) {
// TODO
}
/**
* @see org.objectweb.fractal.api.control.LifeCycleController#getFcState()
*/
public final String getFcState() {
return this.fcState;
}
/**
* @see org.objectweb.fractal.api.control.LifeCycleController#startFc()
*/
public final void startFc() throws IllegalLifeCycleException {
log.finer("ScriptEngineComponent starting...");
// When this component is started then it obtains all the properties of its enclosing SCA component
// and put them as variables in the scripting engine.
String [] properties = scaPropertyController.getPropertyNames();
for (String property : properties) {
Object value = scaPropertyController.getValue(property);
log.info("Affect the scripting variable '" + property + "' with the value '" + value + "'");
scriptEngine.put(property, value);
}
this.fcState = LifeCycleController.STARTED;
log.finer("ScriptEngineComponent started.");
}
/**
* @see org.objectweb.fractal.api.control.LifeCycleController#stopFc()
*/
public final void stopFc() throws IllegalLifeCycleException {
this.fcState = LifeCycleController.STOPPED;
log.finer("ScriptEngineComponent stopped.");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy